
The program sends the current location over the cellular network.
Dependencies: aconno_I2C ublox-at-cellular-interface gnss ublox-cellular-base Lis2dh12 ublox-cellular-base-n2xx ublox-at-cellular-interface-n2xx low-power-sleep
Fork of example-gnss by
tasks.cpp
00001 /** 00002 * Collection of all tasks 00003 * Made by Jurica @ aconno 00004 * More info @ aconno.de 00005 * 00006 */ 00007 00008 #include "tasks.h" 00009 #include "aconnoHelpers.h" 00010 #include "gnss.h" 00011 #include "aconnoConfig.h" 00012 00013 extern volatile MainStates state; 00014 extern char locationGlobal[5*UDP_MSG_SIZE_B]; 00015 extern Thread alarmState; 00016 locationFlag_t locationFlagGlobal = OLD; 00017 00018 void idleCallback() 00019 { 00020 while(1) 00021 { 00022 Thread::signal_wait(IDLE_SIGNAL); 00023 Thread::signal_clr(IDLE_SIGNAL); 00024 printf("In idle thread.\r\n"); 00025 while(state == STATE_IDLE) 00026 { 00027 wait_ms(1000); 00028 } 00029 } 00030 } 00031 00032 void alarmCallback(myParams_t *myParams) 00033 { 00034 char udpMsg[UDP_MSG_SIZE_B]; 00035 00036 while(1) 00037 { 00038 while(state == STATE_ALARM) 00039 { 00040 Thread::signal_wait(LOCATION_SEARCH_DONE); 00041 Thread::signal_clr(LOCATION_SEARCH_DONE); 00042 if(locationFlagGlobal) 00043 { 00044 sprintf(udpMsg, "$location_flag:%s;$type:NEW;",locationGlobal); 00045 } 00046 else 00047 { 00048 sprintf(udpMsg, "$location_flag:%s;$type:OLD;",locationGlobal); 00049 } 00050 printf("Sending location on server...\r\n"); 00051 printf("%s\r\n", udpMsg); 00052 myParams->sara->sendUdpMsg(udpMsg); 00053 wait_ms(5000); 00054 } 00055 } 00056 } 00057 00058 void alarmOffCallback() 00059 { 00060 while(1) 00061 { 00062 Thread::signal_wait(ALARM_OFF_SIGNAL); 00063 Thread::signal_clr(ALARM_OFF_SIGNAL); 00064 printf("In alarm off thread.\r\n"); 00065 while(state == STATE_ALARM_OFF) 00066 { 00067 wait_ms(1000); 00068 } 00069 } 00070 } 00071 00072 void movementCallback() 00073 { 00074 while(1) 00075 { 00076 while(state == STATE_LIS_DETECTION) 00077 { 00078 printf("In movement thread.\r\n"); 00079 wait_ms(1000); 00080 } 00081 } 00082 } 00083 00084 void gnssLocationCallback(GnssSerial *gnss) 00085 { 00086 while(1) 00087 { 00088 while(state == STATE_ALARM) 00089 { 00090 if(getGPSData(locationGlobal, gnss, MAX_TIME_GNSS_WAIT_S)) 00091 { 00092 printf("New location.\r\n"); 00093 locationFlagGlobal = NEW; 00094 } 00095 else 00096 { 00097 printf("Old location.\r\n"); 00098 locationFlagGlobal = OLD; 00099 } 00100 alarmState.signal_set(LOCATION_SEARCH_DONE); 00101 Thread::wait(UPDATE_LOCATION_TIME_MS); 00102 } 00103 } 00104 } 00105
Generated on Tue Jul 12 2022 15:46:17 by
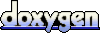