
The program sends the current location over the cellular network.
Dependencies: aconno_I2C ublox-at-cellular-interface gnss ublox-cellular-base Lis2dh12 ublox-cellular-base-n2xx ublox-at-cellular-interface-n2xx low-power-sleep
Fork of example-gnss by
aconnoHelpers.cpp
00001 /** 00002 * aconno helpers 00003 * Set of general purpose fuctions used within aconno projects 00004 * 00005 */ 00006 00007 #include "mbed.h" 00008 #include "aconnoHelpers.h" 00009 #include "stdlib.h" 00010 #include "gnss.h" 00011 #include "aconnoConfig.h" 00012 00013 bool getGPSData(char *location, GnssSerial *gnss, uint32_t timeoutS) 00014 { 00015 int gnssReturnCode; 00016 int length; 00017 char buffer[505]; 00018 bool gotLocationFlag = false; 00019 int begin; 00020 int end; 00021 00022 if(!timeoutS) 00023 { 00024 // Max number of seconds 00025 timeoutS -= 1; 00026 } 00027 00028 Timer timeoutTimer; 00029 timeoutTimer.start(); 00030 begin = timeoutTimer.read(); 00031 do 00032 { 00033 gnssReturnCode = gnss->getMessage(buffer, sizeof(buffer)); 00034 if (gnssReturnCode > 0) 00035 { 00036 // Msg from GNSS module received 00037 length = LENGTH(gnssReturnCode); 00038 if ((PROTOCOL(gnssReturnCode) == GnssParser::NMEA) && (length > 6)) 00039 { 00040 // Talker is $GA=Galileo $GB=Beidou $GL=Glonass 00041 // $GN=Combined $GP=GNSS 00042 if ((buffer[0] == '$') || buffer[1] == 'G') 00043 { 00044 if (CHECK_TALKER("GLL")) 00045 { 00046 00047 double latitude = 0, longitude = 0; 00048 char ch; 00049 if (gnss->getNmeaAngle(1, buffer, length, latitude) && 00050 gnss->getNmeaAngle(3, buffer, length, longitude) && 00051 gnss->getNmeaItem(6, buffer, length, ch) && 00052 (ch == 'A')) 00053 { 00054 sprintf(location, "%.5f %.5f", latitude, longitude); 00055 printf("Got location!\r\n"); 00056 gotLocationFlag = true; 00057 } 00058 } 00059 } 00060 } 00061 } 00062 end = timeoutTimer.read(); 00063 } while (!gotLocationFlag && (end - begin) < timeoutS); 00064 00065 printf("Location time: %d\r\n", (end - begin)); 00066 return gotLocationFlag; 00067 } 00068
Generated on Tue Jul 12 2022 15:46:17 by
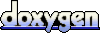