
Simple example to demonstrate custom made BLE service and characteristics.
Fork of BLE_GATT_Example by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <mbed-events/events.h> 00018 #include <mbed.h> 00019 #include "ble/BLE.h" 00020 #include "AckService.h" 00021 00022 #define DEBUG (0) 00023 #define MSD_SIZE (18) 00024 00025 #if DEBUG 00026 #define RX (p26) // SERIAL STILL DOES NOT WORK!!! 00027 #define TX (p25) 00028 #endif 00029 00030 ACKService<4> *ackServicePtr; 00031 #if DEBUG 00032 Serial pc(TX, RX); 00033 #endif 00034 00035 BLE &ble = BLE::Instance(); 00036 00037 DigitalOut redLed(p22); 00038 DigitalOut greenLed(p24); 00039 DigitalOut blueLed(p23); 00040 DigitalOut alivenessLED(p26); 00041 00042 const static char DEVICE_NAME[] = "CRP_ACK"; 00043 const static uint16_t ACK_CHARACTERISTIC_UUID = 0xA001; 00044 const static uint16_t ACK_SERVICE_UUID = 0xA000; 00045 uint8_t MSD[MSD_SIZE] = {0x59, 0x00, 0xE1, 0x61, 0x35, 0xBA, 0xC0, 0xEC, 0x47, 0x2A, 0x98, 0x00, 0xAF, 0x18, 0x43, 0xFF, 0x05, 0x00}; 00046 uint8_t macAddress[6] = {0x23, 0x24, 0x35, 0x22, 0x24, 0x45}; 00047 00048 static EventQueue eventQueue( 00049 /* event count */ 10 * /* event size */ 32 00050 ); 00051 00052 void onConnectionCallback(const Gap::ConnectionCallbackParams_t *params){ 00053 #if DEBUG 00054 pc.printf("BLE device is connected.\n"); 00055 #endif 00056 redLed = 1; 00057 greenLed = 1; 00058 blueLed = 0; 00059 } 00060 00061 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params){ 00062 (void) params; 00063 redLed = 0; 00064 greenLed = 1; 00065 blueLed = 1; 00066 ble.disconnect(Gap::LOCAL_HOST_TERMINATED_CONNECTION); 00067 BLE::Instance().gap().startAdvertising(); 00068 } 00069 00070 void blinkCallback(void){ 00071 alivenessLED = !alivenessLED; /* Do blinky on LED1 to indicate system aliveness. */ 00072 } 00073 00074 void updateMac(const GattReadCallbackParams *response){ 00075 ackServicePtr->updateMacAddress(macAddress); // Update MAC address 00076 } 00077 00078 00079 /** 00080 * This callback allows the LEDService to receive updates to the ledState Characteristic. 00081 * 00082 * @param[in] params 00083 * Information about the characterisitc being updated. 00084 */ 00085 void onDataWrittenCallback(const GattWriteCallbackParams *params) { 00086 #if DEBUG 00087 pc.printf("Data written into characteristic.\n"); 00088 #endif 00089 00090 00091 if(params->data[0] == 0xBA) 00092 if(params->data[1] == 0xBE) 00093 greenLed = 0; 00094 00095 if(params->data[0] == 0xDE) 00096 if(params->data[1] == 0xAD) 00097 greenLed = 1; 00098 } 00099 00100 /** 00101 * This function is called when the ble initialization process has failled 00102 */ 00103 void onBleInitError(BLE &ble, ble_error_t error){ 00104 /* Initialization error handling should go here */ 00105 } 00106 00107 /** 00108 * Callback triggered when the ble initialization process has finished 00109 */ 00110 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params){ 00111 BLE& ble = params->ble; 00112 ble_error_t error = params->error; 00113 00114 if (error != BLE_ERROR_NONE) { 00115 /* In case of error, forward the error handling to onBleInitError */ 00116 onBleInitError(ble, error); 00117 return; 00118 } 00119 00120 /* Ensure that it is the default instance of BLE */ 00121 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00122 return; 00123 } 00124 00125 ble.gap().onDisconnection(disconnectionCallback); 00126 ble.gap().onConnection(onConnectionCallback); 00127 ble.gattServer().onDataWritten(onDataWrittenCallback); 00128 ble.gattClient().onDataRead(updateMac); 00129 00130 uint8_t init_values[4] = {0,0,0,0}; 00131 /* Get my MAC address */ 00132 BLEProtocol::AddressType_t macAddressType; 00133 ble.gap().getAddress(&macAddressType, macAddress); 00134 ackServicePtr = new ACKService<4>(ble, init_values); 00135 ackServicePtr->updateMacAddress(macAddress); // Update MAC address 00136 00137 /* setup advertising */ 00138 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00139 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00140 //ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00141 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, (uint8_t *)MSD, MSD_SIZE); 00142 ble.gap().setAdvertisingInterval(500); /* 1000ms. */ 00143 ble.gap().startAdvertising(); 00144 } 00145 00146 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00147 BLE &ble = BLE::Instance(); 00148 eventQueue.post(Callback<void()>(&ble, &BLE::processEvents)); 00149 } 00150 00151 00152 00153 int main(){ 00154 redLed = 1; 00155 greenLed = 1; 00156 blueLed = 1; 00157 00158 eventQueue.post_every(500, blinkCallback); 00159 00160 ble.onEventsToProcess(scheduleBleEventsProcessing); 00161 ble.init(bleInitComplete); 00162 00163 while (true) { 00164 eventQueue.dispatch(); 00165 } 00166 }
Generated on Tue Jul 19 2022 20:19:12 by
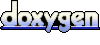