library to modify and read program variable in runtime from a serial console. You can reset as well the mbed from the console without pushing buttons. Handy for debugging from the online compiler as you can change the behavior of the program without need to recompile each time.
VarStore.h
00001 #ifndef VARSTORE_H 00002 #define VARSTORE_H 00003 #include "mbed.h" 00004 #include "VarItems.h" 00005 00006 #define STR_OK "" 00007 00008 /** VarStore Class beta !!!!!!! 00009 * Used for reading/modifying program variables from the console at runtime. 00010 * Helpful for debugging 00011 * It has a facility as well to reset the mbed 00012 * from the serial console without pressing 00013 * any button. 00014 * It does not block the serial/input channel 00015 * in case it is needed for other stuff 00016 * 00017 * From the console ( be aware that if you do not have local echo activated you wil not see what you tye. Commands are 00018 * triggered at CR 00019 * 00020 * s:var:value -> sets the value of var to value at runtime 00021 * d:var -> dump content of var 00022 * s:arr:val1,val2,val3 -> set first three values of arr to val1,val2,val3 00023 * r -> reset mbed... and reload program 00024 * w:milisecs -> (beta) release the console input for your program for a period of time7 00025 * 00026 * I do have in a note pad sets of commands, e.g. dumps of variables I want to check and then I just 00027 * copy and paste in to the terminal so see all of them at once or repeteadly show them. 00028 * 00029 * hope it helps. 00030 * 00031 * Example: 00032 * @code 00033 * 00034 * #include "mbed.h" 00035 * #include "rtos.h" 00036 * #include "VarStore.h" 00037 * 00038 * #include <RawSerial.h> 00039 * 00040 * RawSerial pc(USBTX,USBRX); // Be aware !!!! need rawserial. No printf for you anymore !! 00041 * // no malloc nither as this works in ISR 00042 * 00043 * VarStore Store(&pc,20); // create storage for 20 variables/arrays attach to serial pc 00044 * 00045 * DigitalOut led1(LED1); 00046 * DigitalOut led2(LED2); 00047 * 00048 * void led2_thread(void const *args) { 00049 * 00050 * int wait2=1000; 00051 * Store.Load("wait2",&wait2,T_int); // load variable wait2 in Store 00052 * 00053 * while (true) { 00054 * led2 = !led2; 00055 * Thread::wait(wait2); // remember, no WAIT, STOPS CPU FROM RUNNING WHOLE PROGRAM. 00056 * // use Thread::wait to stop just your path of execution. 00057 * } 00058 * } 00059 * 00060 * int main() { 00061 * 00062 * int wait1=500; 00063 * Store.Load("wait1",&wait1,T_int); // Load variable wait1 in Store 00064 * Thread VS_thread(VarStore::Worker,&Store,osPriorityNormal,DEFAULT_STACK_SIZE,NULL); // launch VarStore Thread 00065 * 00066 * Thread thread(led2_thread); 00067 * 00068 * while (true) { 00069 * led1 = !led1; 00070 * Thread::wait(wait1); // remember, no WAIT, STOPS CPU FROM RUNNING WHOLE PROGRAM. 00071 * // use Thread::wait to stop just your path of execution. 00072 * } 00073 * } 00074 * 00075 * @endcode 00076 * 00077 * 00078 * 00079 * 00080 */ 00081 class VarStore 00082 { 00083 00084 00085 // friend void Worker2(); 00086 public: 00087 /* 00088 * Constructor 00089 */ 00090 VarStore( RawSerial *ser, int sz); 00091 00092 /* 00093 * destr 00094 */ 00095 virtual ~VarStore(); 00096 00097 /* assigns (a) value(s) to a variable or array 00098 */ 00099 char * Set(char *Input); 00100 00101 /*Get contents of a variable as a string 00102 */ 00103 char* Get(char *Name); 00104 00105 /** Load a variable on VarStore 00106 * 00107 * @param Name string that will be used to query/set the value of the variable/array 00108 * @param VarPtr pointer to variable 00109 * @param VarType enumerated type indicating int/float ( only supported currently) enum VarTypes {T_int,T_float}; 00110 * 00111 * @returns ERR on error / NULL on success 00112 */ 00113 int Load(char *Name, void *VarPtr,VarTypes VarType ); 00114 00115 /** Load an array on VarStore 00116 * 00117 * @param Name string that will be used to query/set the value of the variable/array 00118 * @param VarPtr pointer to base of array 00119 * @param VarType enumerated type indicating int/float ( only supported currently) enum VarTypes {T_int,T_float}; 00120 * @param Size number of elements in the array 00121 * 00122 * @returns ERR on error / NULL on success 00123 */ 00124 int Load(char *Name, void *VarPtr,VarTypes VarType, int Size ); 00125 00126 00127 00128 /** Thread that will manage the console interaction. Main role is to remain sleeping 00129 * and only awakening to fire worker2 to deal with console input 00130 * 00131 * @param args pointer to the VarStore Object that will be managing. 00132 * 00133 * @returns ERR on error / NULL on success 00134 */ 00135 static void Worker(void const *args); 00136 00137 00138 protected: 00139 00140 private: 00141 /* Get variable item by name 00142 */ 00143 VarItem *GetVar(char *name); 00144 00145 /* do stuff with console input 00146 */ 00147 char *Do(char *str); 00148 00149 /* manages console input 00150 */ 00151 static void Worker2(); 00152 00153 00154 VarItem *Store; 00155 int sz; // number of varibales to store / array size 00156 int VarCounter; 00157 RawSerial *pc; 00158 00159 static VarStore *MyThis; // used by the workers reading the terminal 00160 // instantiation via a static fucntion so need to record 00161 // who am I 00162 }; 00163 00164 00165 00166 #endif // VARSTORE_H
Generated on Thu Jul 14 2022 19:34:10 by
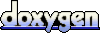