library to modify and read program variable in runtime from a serial console. You can reset as well the mbed from the console without pushing buttons. Handy for debugging from the online compiler as you can change the behavior of the program without need to recompile each time.
VarItems.cpp
00001 #include "VarItems.h" 00002 #include <string.h> 00003 #include <stdlib.h> 00004 #include <stdio.h> 00005 #include "mbed.h" 00006 00007 extern RawSerial pc; 00008 00009 #define DMP_SZ 132 00010 00011 00012 VarItem::VarItem() 00013 { 00014 VarName[0]='\0'; 00015 00016 ValInt=NULL; 00017 ValFloat=NULL; 00018 ArraySize=0; 00019 } 00020 00021 VarItem::~VarItem() 00022 { 00023 //dtor 00024 } 00025 00026 int VarItem::SetVal(char *Val) 00027 { 00028 char * Values; 00029 unsigned int Count=0; 00030 00031 Values=strtok(Val,","); 00032 if(Values) { 00033 do { 00034 switch(VarType) { 00035 00036 case T_int: 00037 *(ValInt+Count) = atoi(Values); 00038 break; 00039 00040 case T_float: 00041 *(ValFloat+Count) = atof(Values); 00042 break; 00043 }; 00044 Count++; 00045 Values=strtok(NULL,","); 00046 } while((Values !=NULL) && (Count < ArraySize)); 00047 return 1; 00048 } else 00049 return ERR; 00050 00051 } 00052 00053 void VarItem::SetVar(VarTypes VT,void* VarPtr) 00054 { 00055 00056 VarType=VT; 00057 switch(VarType) { 00058 00059 case T_int: 00060 ValInt = (int *) VarPtr; 00061 break; 00062 00063 case T_float: 00064 ValFloat = (float*) VarPtr; 00065 break; 00066 00067 }; 00068 00069 } 00070 00071 00072 void VarItem::SetVarArraySize(int Size) 00073 { 00074 ArraySize=Size; 00075 } 00076 00077 00078 void VarItem::SetVarName(char *Name) 00079 { 00080 strncpy(VarName,Name,VAR_NAME_LEN); 00081 VarName[VAR_NAME_LEN-1]='\0'; 00082 } 00083 00084 00085 char *VarItem::Dump() 00086 { 00087 static char StrDump[DMP_SZ]; 00088 unsigned int DumpSize=DMP_SZ; 00089 unsigned int DumpCounter=0, ArrayCounter=0;; 00090 char Tmp[16]; 00091 00092 memset(StrDump,0,DMP_SZ); 00093 00094 do { 00095 switch(VarType) { 00096 case T_int: 00097 sprintf(Tmp,"%d,",*(ValInt+ArrayCounter)); 00098 break; 00099 case T_float: 00100 sprintf(Tmp,"%f,",*(ValFloat+ArrayCounter)); 00101 break; 00102 }; 00103 00104 if(DumpCounter+strlen(Tmp) >= DumpSize) break; 00105 00106 strcat(StrDump+DumpCounter,Tmp); 00107 DumpCounter+=strlen(Tmp); 00108 ArrayCounter++; 00109 } while (ArrayCounter < ArraySize); 00110 00111 StrDump[DumpSize-1]='\0'; 00112 00113 return StrDump; 00114 00115 } 00116 00117 00118 char *VarItem::GetVarName() 00119 { 00120 return VarName; 00121 } 00122 00123
Generated on Thu Jul 14 2022 19:34:10 by
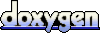