
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
optical_flow.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009-2011, Willow Garage Inc., all rights reserved. 00015 // Third party copyrights are property of their respective owners. 00016 // 00017 // Redistribution and use in source and binary forms, with or without modification, 00018 // are permitted provided that the following conditions are met: 00019 // 00020 // * Redistribution's of source code must retain the above copyright notice, 00021 // this list of conditions and the following disclaimer. 00022 // 00023 // * Redistribution's in binary form must reproduce the above copyright notice, 00024 // this list of conditions and the following disclaimer in the documentation 00025 // and/or other materials provided with the distribution. 00026 // 00027 // * The name of the copyright holders may not be used to endorse or promote products 00028 // derived from this software without specific prior written permission. 00029 // 00030 // This software is provided by the copyright holders and contributors "as is" and 00031 // any express or implied warranties, including, but not limited to, the implied 00032 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00033 // In no event shall the Intel Corporation or contributors be liable for any direct, 00034 // indirect, incidental, special, exemplary, or consequential damages 00035 // (including, but not limited to, procurement of substitute goods or services; 00036 // loss of use, data, or profits; or business interruption) however caused 00037 // and on any theory of liability, whether in contract, strict liability, 00038 // or tort (including negligence or otherwise) arising in any way out of 00039 // the use of this software, even if advised of the possibility of such damage. 00040 // 00041 //M*/ 00042 00043 #ifndef __OPENCV_VIDEOSTAB_OPTICAL_FLOW_HPP__ 00044 #define __OPENCV_VIDEOSTAB_OPTICAL_FLOW_HPP__ 00045 00046 #include "opencv2/core.hpp" 00047 #include "opencv2/opencv_modules.hpp" 00048 00049 #ifdef HAVE_OPENCV_CUDAOPTFLOW 00050 #include "opencv2/cudaoptflow.hpp" 00051 #endif 00052 00053 namespace cv 00054 { 00055 namespace videostab 00056 { 00057 00058 //! @addtogroup videostab 00059 //! @{ 00060 00061 class CV_EXPORTS ISparseOptFlowEstimator 00062 { 00063 public: 00064 virtual ~ISparseOptFlowEstimator() {} 00065 virtual void run( 00066 InputArray frame0, InputArray frame1, InputArray points0, InputOutputArray points1, 00067 OutputArray status, OutputArray errors) = 0; 00068 }; 00069 00070 class CV_EXPORTS IDenseOptFlowEstimator 00071 { 00072 public: 00073 virtual ~IDenseOptFlowEstimator() {} 00074 virtual void run( 00075 InputArray frame0, InputArray frame1, InputOutputArray flowX, InputOutputArray flowY, 00076 OutputArray errors) = 0; 00077 }; 00078 00079 class CV_EXPORTS PyrLkOptFlowEstimatorBase 00080 { 00081 public: 00082 PyrLkOptFlowEstimatorBase() { setWinSize(Size(21, 21)); setMaxLevel(3); } 00083 00084 virtual void setWinSize(Size val) { winSize_ = val; } 00085 virtual Size winSize() const { return winSize_; } 00086 00087 virtual void setMaxLevel(int val) { maxLevel_ = val; } 00088 virtual int maxLevel() const { return maxLevel_; } 00089 virtual ~PyrLkOptFlowEstimatorBase() {} 00090 00091 protected: 00092 Size winSize_; 00093 int maxLevel_; 00094 }; 00095 00096 class CV_EXPORTS SparsePyrLkOptFlowEstimator 00097 : public PyrLkOptFlowEstimatorBase, public ISparseOptFlowEstimator 00098 { 00099 public: 00100 virtual void run( 00101 InputArray frame0, InputArray frame1, InputArray points0, InputOutputArray points1, 00102 OutputArray status, OutputArray errors); 00103 }; 00104 00105 #ifdef HAVE_OPENCV_CUDAOPTFLOW 00106 00107 class CV_EXPORTS SparsePyrLkOptFlowEstimatorGpu 00108 : public PyrLkOptFlowEstimatorBase, public ISparseOptFlowEstimator 00109 { 00110 public: 00111 SparsePyrLkOptFlowEstimatorGpu(); 00112 00113 virtual void run( 00114 InputArray frame0, InputArray frame1, InputArray points0, InputOutputArray points1, 00115 OutputArray status, OutputArray errors); 00116 00117 void run(const cuda::GpuMat &frame0, const cuda::GpuMat &frame1, const cuda::GpuMat &points0, cuda::GpuMat &points1, 00118 cuda::GpuMat &status, cuda::GpuMat &errors); 00119 00120 void run(const cuda::GpuMat &frame0, const cuda::GpuMat &frame1, const cuda::GpuMat &points0, cuda::GpuMat &points1, 00121 cuda::GpuMat &status); 00122 00123 private: 00124 Ptr<cuda::SparsePyrLKOpticalFlow> optFlowEstimator_; 00125 cuda::GpuMat frame0_, frame1_, points0_, points1_, status_, errors_; 00126 }; 00127 00128 class CV_EXPORTS DensePyrLkOptFlowEstimatorGpu 00129 : public PyrLkOptFlowEstimatorBase, public IDenseOptFlowEstimator 00130 { 00131 public: 00132 DensePyrLkOptFlowEstimatorGpu(); 00133 00134 virtual void run( 00135 InputArray frame0, InputArray frame1, InputOutputArray flowX, InputOutputArray flowY, 00136 OutputArray errors); 00137 00138 private: 00139 Ptr<cuda::DensePyrLKOpticalFlow> optFlowEstimator_; 00140 cuda::GpuMat frame0_, frame1_, flowX_, flowY_, errors_; 00141 }; 00142 00143 #endif 00144 00145 //! @} 00146 00147 } // namespace videostab 00148 } // namespace cv 00149 00150 #endif 00151
Generated on Tue Jul 12 2022 16:42:39 by
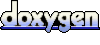