
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
util_inl.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Third party copyrights are property of their respective owners. 00016 // 00017 // Redistribution and use in source and binary forms, with or without modification, 00018 // are permitted provided that the following conditions are met: 00019 // 00020 // * Redistribution's of source code must retain the above copyright notice, 00021 // this list of conditions and the following disclaimer. 00022 // 00023 // * Redistribution's in binary form must reproduce the above copyright notice, 00024 // this list of conditions and the following disclaimer in the documentation 00025 // and/or other materials provided with the distribution. 00026 // 00027 // * The name of the copyright holders may not be used to endorse or promote products 00028 // derived from this software without specific prior written permission. 00029 // 00030 // This software is provided by the copyright holders and contributors "as is" and 00031 // any express or implied warranties, including, but not limited to, the implied 00032 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00033 // In no event shall the Intel Corporation or contributors be liable for any direct, 00034 // indirect, incidental, special, exemplary, or consequential damages 00035 // (including, but not limited to, procurement of substitute goods or services; 00036 // loss of use, data, or profits; or business interruption) however caused 00037 // and on any theory of liability, whether in contract, strict liability, 00038 // or tort (including negligence or otherwise) arising in any way out of 00039 // the use of this software, even if advised of the possibility of such damage. 00040 // 00041 //M*/ 00042 00043 #ifndef __OPENCV_STITCHING_UTIL_INL_HPP__ 00044 #define __OPENCV_STITCHING_UTIL_INL_HPP__ 00045 00046 #include <queue> 00047 #include "opencv2/core.hpp" 00048 #include "util.hpp" // Make your IDE see declarations 00049 00050 //! @cond IGNORED 00051 00052 namespace cv { 00053 namespace detail { 00054 00055 template <typename B> 00056 B Graph::forEach(B body) const 00057 { 00058 for (int i = 0; i < numVertices(); ++i) 00059 { 00060 std::list<GraphEdge>::const_iterator edge = edges_[i].begin(); 00061 for (; edge != edges_[i].end(); ++edge) 00062 body(*edge); 00063 } 00064 return body; 00065 } 00066 00067 00068 template <typename B> 00069 B Graph::walkBreadthFirst(int from, B body) const 00070 { 00071 std::vector<bool> was(numVertices(), false); 00072 std::queue<int> vertices; 00073 00074 was[from] = true; 00075 vertices.push(from); 00076 00077 while (!vertices.empty()) 00078 { 00079 int vertex = vertices.front(); 00080 vertices.pop(); 00081 00082 std::list<GraphEdge>::const_iterator edge = edges_[vertex].begin(); 00083 for (; edge != edges_[vertex].end(); ++edge) 00084 { 00085 if (!was[edge->to]) 00086 { 00087 body(*edge); 00088 was[edge->to] = true; 00089 vertices.push(edge->to); 00090 } 00091 } 00092 } 00093 00094 return body; 00095 } 00096 00097 00098 ////////////////////////////////////////////////////////////////////////////// 00099 // Some auxiliary math functions 00100 00101 static inline 00102 float normL2(const Point3f& a) 00103 { 00104 return a.x * a.x + a.y * a.y + a.z * a.z; 00105 } 00106 00107 00108 static inline 00109 float normL2(const Point3f& a, const Point3f& b) 00110 { 00111 return normL2(a - b); 00112 } 00113 00114 00115 static inline 00116 double normL2sq(const Mat &r) 00117 { 00118 return r.dot(r); 00119 } 00120 00121 00122 static inline int sqr(int x) { return x * x; } 00123 static inline float sqr(float x) { return x * x; } 00124 static inline double sqr(double x) { return x * x; } 00125 00126 } // namespace detail 00127 } // namespace cv 00128 00129 //! @endcond 00130 00131 #endif // __OPENCV_STITCHING_UTIL_INL_HPP__ 00132
Generated on Tue Jul 12 2022 16:42:40 by
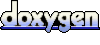