
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
saturate.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Copyright (C) 2014, Itseez Inc., all rights reserved. 00017 // Third party copyrights are property of their respective owners. 00018 // 00019 // Redistribution and use in source and binary forms, with or without modification, 00020 // are permitted provided that the following conditions are met: 00021 // 00022 // * Redistribution's of source code must retain the above copyright notice, 00023 // this list of conditions and the following disclaimer. 00024 // 00025 // * Redistribution's in binary form must reproduce the above copyright notice, 00026 // this list of conditions and the following disclaimer in the documentation 00027 // and/or other materials provided with the distribution. 00028 // 00029 // * The name of the copyright holders may not be used to endorse or promote products 00030 // derived from this software without specific prior written permission. 00031 // 00032 // This software is provided by the copyright holders and contributors "as is" and 00033 // any express or implied warranties, including, but not limited to, the implied 00034 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00035 // In no event shall the Intel Corporation or contributors be liable for any direct, 00036 // indirect, incidental, special, exemplary, or consequential damages 00037 // (including, but not limited to, procurement of substitute goods or services; 00038 // loss of use, data, or profits; or business interruption) however caused 00039 // and on any theory of liability, whether in contract, strict liability, 00040 // or tort (including negligence or otherwise) arising in any way out of 00041 // the use of this software, even if advised of the possibility of such damage. 00042 // 00043 //M*/ 00044 00045 #ifndef __OPENCV_CORE_SATURATE_HPP__ 00046 #define __OPENCV_CORE_SATURATE_HPP__ 00047 00048 #include "opencv2/core/cvdef.h" 00049 #include "opencv2/core/fast_math.hpp" 00050 00051 namespace cv 00052 { 00053 00054 //! @addtogroup core_utils 00055 //! @{ 00056 00057 /////////////// saturate_cast (used in image & signal processing) /////////////////// 00058 00059 /** @brief Template function for accurate conversion from one primitive type to another. 00060 00061 The functions saturate_cast resemble the standard C++ cast operations, such as static_cast<T>() 00062 and others. They perform an efficient and accurate conversion from one primitive type to another 00063 (see the introduction chapter). saturate in the name means that when the input value v is out of the 00064 range of the target type, the result is not formed just by taking low bits of the input, but instead 00065 the value is clipped. For example: 00066 @code 00067 uchar a = saturate_cast<uchar>(-100); // a = 0 (UCHAR_MIN) 00068 short b = saturate_cast<short>(33333.33333); // b = 32767 (SHRT_MAX) 00069 @endcode 00070 Such clipping is done when the target type is unsigned char , signed char , unsigned short or 00071 signed short . For 32-bit integers, no clipping is done. 00072 00073 When the parameter is a floating-point value and the target type is an integer (8-, 16- or 32-bit), 00074 the floating-point value is first rounded to the nearest integer and then clipped if needed (when 00075 the target type is 8- or 16-bit). 00076 00077 This operation is used in the simplest or most complex image processing functions in OpenCV. 00078 00079 @param v Function parameter. 00080 @sa add, subtract, multiply, divide, Mat::convertTo 00081 */ 00082 template<typename _Tp> static inline _Tp saturate_cast(uchar v) { return _Tp(v); } 00083 /** @overload */ 00084 template<typename _Tp> static inline _Tp saturate_cast(schar v) { return _Tp(v); } 00085 /** @overload */ 00086 template<typename _Tp> static inline _Tp saturate_cast(ushort v) { return _Tp(v); } 00087 /** @overload */ 00088 template<typename _Tp> static inline _Tp saturate_cast(short v) { return _Tp(v); } 00089 /** @overload */ 00090 template<typename _Tp> static inline _Tp saturate_cast(unsigned v) { return _Tp(v); } 00091 /** @overload */ 00092 template<typename _Tp> static inline _Tp saturate_cast(int v) { return _Tp(v); } 00093 /** @overload */ 00094 template<typename _Tp> static inline _Tp saturate_cast(float v) { return _Tp(v); } 00095 /** @overload */ 00096 template<typename _Tp> static inline _Tp saturate_cast(double v) { return _Tp(v); } 00097 /** @overload */ 00098 template<typename _Tp> static inline _Tp saturate_cast(int64 v) { return _Tp(v); } 00099 /** @overload */ 00100 template<typename _Tp> static inline _Tp saturate_cast(uint64 v) { return _Tp(v); } 00101 00102 template<> inline uchar saturate_cast<uchar>(schar v) { return (uchar)std::max((int)v, 0); } 00103 template<> inline uchar saturate_cast<uchar>(ushort v) { return (uchar)std::min((unsigned)v, (unsigned)UCHAR_MAX); } 00104 template<> inline uchar saturate_cast<uchar>(int v) { return (uchar)((unsigned)v <= UCHAR_MAX ? v : v > 0 ? UCHAR_MAX : 0); } 00105 template<> inline uchar saturate_cast<uchar>(short v) { return saturate_cast<uchar>((int)v); } 00106 template<> inline uchar saturate_cast<uchar>(unsigned v) { return (uchar)std::min(v, (unsigned)UCHAR_MAX); } 00107 template<> inline uchar saturate_cast<uchar>(float v) { int iv = cvRound(v); return saturate_cast<uchar>(iv); } 00108 template<> inline uchar saturate_cast<uchar>(double v) { int iv = cvRound(v); return saturate_cast<uchar>(iv); } 00109 template<> inline uchar saturate_cast<uchar>(int64 v) { return (uchar)((uint64)v <= (uint64)UCHAR_MAX ? v : v > 0 ? UCHAR_MAX : 0); } 00110 template<> inline uchar saturate_cast<uchar>(uint64 v) { return (uchar)std::min(v, (uint64)UCHAR_MAX); } 00111 00112 template<> inline schar saturate_cast<schar>(uchar v) { return (schar)std::min((int)v, SCHAR_MAX); } 00113 template<> inline schar saturate_cast<schar>(ushort v) { return (schar)std::min((unsigned)v, (unsigned)SCHAR_MAX); } 00114 template<> inline schar saturate_cast<schar>(int v) { return (schar)((unsigned)(v-SCHAR_MIN) <= (unsigned)UCHAR_MAX ? v : v > 0 ? SCHAR_MAX : SCHAR_MIN); } 00115 template<> inline schar saturate_cast<schar>(short v) { return saturate_cast<schar>((int)v); } 00116 template<> inline schar saturate_cast<schar>(unsigned v) { return (schar)std::min(v, (unsigned)SCHAR_MAX); } 00117 template<> inline schar saturate_cast<schar>(float v) { int iv = cvRound(v); return saturate_cast<schar>(iv); } 00118 template<> inline schar saturate_cast<schar>(double v) { int iv = cvRound(v); return saturate_cast<schar>(iv); } 00119 template<> inline schar saturate_cast<schar>(int64 v) { return (schar)((uint64)((int64)v-SCHAR_MIN) <= (uint64)UCHAR_MAX ? v : v > 0 ? SCHAR_MAX : SCHAR_MIN); } 00120 template<> inline schar saturate_cast<schar>(uint64 v) { return (schar)std::min(v, (uint64)SCHAR_MAX); } 00121 00122 template<> inline ushort saturate_cast<ushort>(schar v) { return (ushort)std::max((int)v, 0); } 00123 template<> inline ushort saturate_cast<ushort>(short v) { return (ushort)std::max((int)v, 0); } 00124 template<> inline ushort saturate_cast<ushort>(int v) { return (ushort)((unsigned)v <= (unsigned)USHRT_MAX ? v : v > 0 ? USHRT_MAX : 0); } 00125 template<> inline ushort saturate_cast<ushort>(unsigned v) { return (ushort)std::min(v, (unsigned)USHRT_MAX); } 00126 template<> inline ushort saturate_cast<ushort>(float v) { int iv = cvRound(v); return saturate_cast<ushort>(iv); } 00127 template<> inline ushort saturate_cast<ushort>(double v) { int iv = cvRound(v); return saturate_cast<ushort>(iv); } 00128 template<> inline ushort saturate_cast<ushort>(int64 v) { return (ushort)((uint64)v <= (uint64)USHRT_MAX ? v : v > 0 ? USHRT_MAX : 0); } 00129 template<> inline ushort saturate_cast<ushort>(uint64 v) { return (ushort)std::min(v, (uint64)USHRT_MAX); } 00130 00131 template<> inline short saturate_cast<short>(ushort v) { return (short)std::min((int)v, SHRT_MAX); } 00132 template<> inline short saturate_cast<short>(int v) { return (short)((unsigned)(v - SHRT_MIN) <= (unsigned)USHRT_MAX ? v : v > 0 ? SHRT_MAX : SHRT_MIN); } 00133 template<> inline short saturate_cast<short>(unsigned v) { return (short)std::min(v, (unsigned)SHRT_MAX); } 00134 template<> inline short saturate_cast<short>(float v) { int iv = cvRound(v); return saturate_cast<short>(iv); } 00135 template<> inline short saturate_cast<short>(double v) { int iv = cvRound(v); return saturate_cast<short>(iv); } 00136 template<> inline short saturate_cast<short>(int64 v) { return (short)((uint64)((int64)v - SHRT_MIN) <= (uint64)USHRT_MAX ? v : v > 0 ? SHRT_MAX : SHRT_MIN); } 00137 template<> inline short saturate_cast<short>(uint64 v) { return (short)std::min(v, (uint64)SHRT_MAX); } 00138 00139 template<> inline int saturate_cast<int>(float v) { return cvRound(v); } 00140 template<> inline int saturate_cast<int>(double v) { return cvRound(v); } 00141 00142 // we intentionally do not clip negative numbers, to make -1 become 0xffffffff etc. 00143 template<> inline unsigned saturate_cast<unsigned>(float v) { return cvRound(v); } 00144 template<> inline unsigned saturate_cast<unsigned>(double v) { return cvRound(v); } 00145 00146 //! @} 00147 00148 } // cv 00149 00150 #endif // __OPENCV_CORE_SATURATE_HPP__ 00151
Generated on Tue Jul 12 2022 16:42:40 by
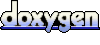