
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
neon_utils.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2015, Itseez Inc., all rights reserved. 00014 // Third party copyrights are property of their respective owners. 00015 // 00016 // Redistribution and use in source and binary forms, with or without modification, 00017 // are permitted provided that the following conditions are met: 00018 // 00019 // * Redistribution's of source code must retain the above copyright notice, 00020 // this list of conditions and the following disclaimer. 00021 // 00022 // * Redistribution's in binary form must reproduce the above copyright notice, 00023 // this list of conditions and the following disclaimer in the documentation 00024 // and/or other materials provided with the distribution. 00025 // 00026 // * The name of the copyright holders may not be used to endorse or promote products 00027 // derived from this software without specific prior written permission. 00028 // 00029 // This software is provided by the copyright holders and contributors "as is" and 00030 // any express or implied warranties, including, but not limited to, the implied 00031 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00032 // In no event shall the Intel Corporation or contributors be liable for any direct, 00033 // indirect, incidental, special, exemplary, or consequential damages 00034 // (including, but not limited to, procurement of substitute goods or services; 00035 // loss of use, data, or profits; or business interruption) however caused 00036 // and on any theory of liability, whether in contract, strict liability, 00037 // or tort (including negligence or otherwise) arising in any way out of 00038 // the use of this software, even if advised of the possibility of such damage. 00039 // 00040 //M*/ 00041 00042 #ifndef __OPENCV_HAL_NEON_UTILS_HPP__ 00043 #define __OPENCV_HAL_NEON_UTILS_HPP__ 00044 00045 #include "opencv2/core/cvdef.h" 00046 00047 //! @addtogroup core_utils_neon 00048 //! @{ 00049 00050 #if CV_NEON 00051 00052 inline int32x2_t cv_vrnd_s32_f32(float32x2_t v) 00053 { 00054 static int32x2_t v_sign = vdup_n_s32(1 << 31), 00055 v_05 = vreinterpret_s32_f32(vdup_n_f32(0.5f)); 00056 00057 int32x2_t v_addition = vorr_s32(v_05, vand_s32(v_sign, vreinterpret_s32_f32(v))); 00058 return vcvt_s32_f32(vadd_f32(v, vreinterpret_f32_s32(v_addition))); 00059 } 00060 00061 inline int32x4_t cv_vrndq_s32_f32(float32x4_t v) 00062 { 00063 static int32x4_t v_sign = vdupq_n_s32(1 << 31), 00064 v_05 = vreinterpretq_s32_f32(vdupq_n_f32(0.5f)); 00065 00066 int32x4_t v_addition = vorrq_s32(v_05, vandq_s32(v_sign, vreinterpretq_s32_f32(v))); 00067 return vcvtq_s32_f32(vaddq_f32(v, vreinterpretq_f32_s32(v_addition))); 00068 } 00069 00070 inline uint32x2_t cv_vrnd_u32_f32(float32x2_t v) 00071 { 00072 static float32x2_t v_05 = vdup_n_f32(0.5f); 00073 return vcvt_u32_f32(vadd_f32(v, v_05)); 00074 } 00075 00076 inline uint32x4_t cv_vrndq_u32_f32(float32x4_t v) 00077 { 00078 static float32x4_t v_05 = vdupq_n_f32(0.5f); 00079 return vcvtq_u32_f32(vaddq_f32(v, v_05)); 00080 } 00081 00082 inline float32x4_t cv_vrecpq_f32(float32x4_t val) 00083 { 00084 float32x4_t reciprocal = vrecpeq_f32(val); 00085 reciprocal = vmulq_f32(vrecpsq_f32(val, reciprocal), reciprocal); 00086 reciprocal = vmulq_f32(vrecpsq_f32(val, reciprocal), reciprocal); 00087 return reciprocal; 00088 } 00089 00090 inline float32x2_t cv_vrecp_f32(float32x2_t val) 00091 { 00092 float32x2_t reciprocal = vrecpe_f32(val); 00093 reciprocal = vmul_f32(vrecps_f32(val, reciprocal), reciprocal); 00094 reciprocal = vmul_f32(vrecps_f32(val, reciprocal), reciprocal); 00095 return reciprocal; 00096 } 00097 00098 inline float32x4_t cv_vrsqrtq_f32(float32x4_t val) 00099 { 00100 float32x4_t e = vrsqrteq_f32(val); 00101 e = vmulq_f32(vrsqrtsq_f32(vmulq_f32(e, e), val), e); 00102 e = vmulq_f32(vrsqrtsq_f32(vmulq_f32(e, e), val), e); 00103 return e; 00104 } 00105 00106 inline float32x2_t cv_vrsqrt_f32(float32x2_t val) 00107 { 00108 float32x2_t e = vrsqrte_f32(val); 00109 e = vmul_f32(vrsqrts_f32(vmul_f32(e, e), val), e); 00110 e = vmul_f32(vrsqrts_f32(vmul_f32(e, e), val), e); 00111 return e; 00112 } 00113 00114 inline float32x4_t cv_vsqrtq_f32(float32x4_t val) 00115 { 00116 return cv_vrecpq_f32(cv_vrsqrtq_f32(val)); 00117 } 00118 00119 inline float32x2_t cv_vsqrt_f32(float32x2_t val) 00120 { 00121 return cv_vrecp_f32(cv_vrsqrt_f32(val)); 00122 } 00123 00124 #endif 00125 00126 //! @} 00127 00128 #endif // __OPENCV_HAL_NEON_UTILS_HPP__ 00129
Generated on Tue Jul 12 2022 16:42:39 by
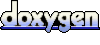