
opencv on mbed
DynamicBitset Class Reference
Class re-implementing the boost version of it This helps not depending on boost, it also does not do the bound checks and has a way to reset a block for speed. More...
#include <dynamic_bitset.h>
Public Member Functions | |
DynamicBitset () | |
default constructor | |
DynamicBitset (size_t sz) | |
only constructor we use in our code | |
void | clear () |
Sets all the bits to 0. | |
bool | empty () const |
checks if the bitset is empty | |
void | reset () |
set all the bits to 0 | |
void | reset (size_t index) |
set one bit to 0 | |
void | reset_block (size_t index) |
sets a specific bit to 0, and more bits too This function is useful when resetting a given set of bits so that the whole bitset ends up being 0: if that's the case, we don't care about setting other bits to 0 | |
void | resize (size_t sz) |
resize the bitset so that it contains at least sz bits | |
void | set (size_t index) |
set a bit to true | |
size_t | size () const |
gives the number of contained bits | |
bool | test (size_t index) const |
check if a bit is set |
Detailed Description
Class re-implementing the boost version of it This helps not depending on boost, it also does not do the bound checks and has a way to reset a block for speed.
Definition at line 57 of file dynamic_bitset.h.
Constructor & Destructor Documentation
DynamicBitset | ( | ) |
default constructor
Definition at line 62 of file dynamic_bitset.h.
DynamicBitset | ( | size_t | sz ) |
only constructor we use in our code
- Parameters:
-
sz the size of the bitset (in bits)
Definition at line 69 of file dynamic_bitset.h.
Member Function Documentation
void clear | ( | ) |
Sets all the bits to 0.
Definition at line 77 of file dynamic_bitset.h.
bool empty | ( | ) | const |
checks if the bitset is empty
- Returns:
- true if the bitset is empty
Definition at line 85 of file dynamic_bitset.h.
void reset | ( | size_t | index ) |
void reset | ( | ) |
set all the bits to 0
Definition at line 92 of file dynamic_bitset.h.
void reset_block | ( | size_t | index ) |
sets a specific bit to 0, and more bits too This function is useful when resetting a given set of bits so that the whole bitset ends up being 0: if that's the case, we don't care about setting other bits to 0
- Parameters:
-
index
Definition at line 111 of file dynamic_bitset.h.
void resize | ( | size_t | sz ) |
resize the bitset so that it contains at least sz bits
- Parameters:
-
sz
Definition at line 119 of file dynamic_bitset.h.
void set | ( | size_t | index ) |
set a bit to true
- Parameters:
-
index the index of the bit to set to 1
Definition at line 128 of file dynamic_bitset.h.
size_t size | ( | ) | const |
gives the number of contained bits
Definition at line 135 of file dynamic_bitset.h.
bool test | ( | size_t | index ) | const |
check if a bit is set
- Parameters:
-
index the index of the bit to check
- Returns:
- true if the bit is set
Definition at line 144 of file dynamic_bitset.h.
Generated on Tue Jul 12 2022 16:42:45 by
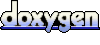