
opencv on mbed
Seq< _Tp > Class Template Reference
[Connections with C++]
#include <core_c.h>
Public Member Functions | |
Seq () | |
the default constructor | |
Seq (const CvSeq *seq) | |
the constructor for wrapping CvSeq structure. The real element type in CvSeq should match _Tp. | |
Seq (MemStorage &storage, int headerSize=sizeof(CvSeq)) | |
creates the empty sequence that resides in the specified storage | |
_Tp & | operator[] (int idx) |
returns read-write reference to the specified element | |
const _Tp & | operator[] (int idx) const |
returns read-only reference to the specified element | |
SeqIterator< _Tp > | begin () const |
returns iterator pointing to the beginning of the sequence | |
SeqIterator< _Tp > | end () const |
returns iterator pointing to the element following the last sequence element | |
size_t | size () const |
returns the number of elements in the sequence | |
int | type () const |
returns the type of sequence elements (CV_8UC1 ... CV_64FC(CV_CN_MAX) ...) | |
int | depth () const |
returns the depth of sequence elements (CV_8U ... CV_64F) | |
int | channels () const |
returns the number of channels in each sequence element | |
size_t | elemSize () const |
returns the size of each sequence element | |
size_t | index (const _Tp &elem) const |
returns index of the specified sequence element | |
void | push_back (const _Tp &elem) |
appends the specified element to the end of the sequence | |
void | push_front (const _Tp &elem) |
appends the specified element to the front of the sequence | |
void | push_back (const _Tp *elems, size_t count) |
appends zero or more elements to the end of the sequence | |
void | push_front (const _Tp *elems, size_t count) |
appends zero or more elements to the front of the sequence | |
void | insert (int idx, const _Tp &elem) |
inserts the specified element to the specified position | |
void | insert (int idx, const _Tp *elems, size_t count) |
inserts zero or more elements to the specified position | |
void | remove (int idx) |
removes element at the specified position | |
void | remove (const Range &r) |
removes the specified subsequence | |
_Tp & | front () |
returns reference to the first sequence element | |
const _Tp & | front () const |
returns read-only reference to the first sequence element | |
_Tp & | back () |
returns reference to the last sequence element | |
const _Tp & | back () const |
returns read-only reference to the last sequence element | |
bool | empty () const |
returns true iff the sequence contains no elements | |
void | clear () |
removes all the elements from the sequence | |
void | pop_front () |
removes the first element from the sequence | |
void | pop_back () |
removes the last element from the sequence | |
void | pop_front (_Tp *elems, size_t count) |
removes zero or more elements from the beginning of the sequence | |
void | pop_back (_Tp *elems, size_t count) |
removes zero or more elements from the end of the sequence | |
void | copyTo (std::vector< _Tp > &vec, const Range &range=Range::all()) const |
copies the whole sequence or the sequence slice to the specified vector | |
operator std::vector< _Tp > () const | |
returns the vector containing all the sequence elements |
Detailed Description
template<typename _Tp>
class cv::Seq< _Tp >
Template Sequence Class derived from CvSeq
The class provides more convenient access to sequence elements, STL-style operations and iterators.
- Note:
- The class is targeted for simple data types, i.e. no constructors or destructors are called for the sequence elements.
Generated on Tue Jul 12 2022 16:42:43 by
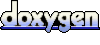