
Testing SHT75 humidity sensor on STM F303K8 board.
Fork of Nucleo-F303K8-SSD1306_OLED by
main.cpp
00001 /* Test ability to read SHT75 sensor from F303K8 board. 00002 00003 Note: Can not be on same pins as I2C due to different pull up 00004 requirements. 00005 00006 By Joseph Ellsworth CTO of A2WH 00007 Take a look at A2WH.com Producing Water from Air using Solar Energy 00008 March-2016 License: https://developer.mbed.org/handbook/MIT-Licence 00009 Please contact us http://a2wh.com for help with custom design projects. 00010 00011 Sample code to test SHT75 humidity sensor using STM F303K8 board. 00012 Uses a 3.3V from board to power sensor. 10K resistor Pull-up on data. 00013 Must not be on same pins as I2C. Uses D0 for Clk and D1 for Data. 00014 00015 I had to modify sample code supplied by https://developer.mbed.org/users/nimbusgb/code/SHT75/ 00016 because the sensor failed to read without the softReset() and readStatus() at beginning 00017 of measurement loop. I think this is caused by the 72Mhtz speed of the F303K8 but did 00018 not attempt to fully diagnose. 00019 00020 The readStatus() method from library seems to malfunction and always return a -1 00021 which never changes even when sensor is unplugged. 00022 00023 00024 */ 00025 00026 #include "mbed.h" 00027 #include <stdint.h> 00028 00029 #define sht75_clk D0 00030 #define sht75_data D1 00031 #include "sht7X.h" 00032 SHT75 sht(sht75_clk, sht75_data); 00033 00034 00035 // Host PC Communication channels 00036 Serial pc(USBTX, USBRX); // tx, rx 00037 char buff[100]; 00038 00039 DigitalOut myled(LED1); 00040 00041 int main() 00042 { 00043 pc.baud(38400); 00044 wait(1); 00045 while(1) { 00046 myled = !myled; 00047 float temperature; // temperature -40 to 120 deg C 00048 float humidity; // relative humidity 1% to 100% 00049 float humi_f,rh_lin,rh_true; // working registers for Illustration purposes 00050 int t; // temporary store for the temp ticks 00051 int h; 00052 sht.softReset(); 00053 int stat = sht.readStatus(); 00054 wait(3.0); 00055 sht.readTempTicks(&t); 00056 temperature = ((float)(t) * 0.01) - 39.61; 00057 00058 sht.readHumidityTicks(&h); 00059 humi_f = (float)(h); 00060 rh_lin = C3 * humi_f * humi_f + C2 * humi_f + C1; 00061 rh_true=(((temperature/100)-25)*(T1+T2*humi_f)+rh_lin); 00062 if(rh_true>100)rh_true=100; //cut if the value is outside 00063 if(rh_true<1)rh_true=1; //the physical possible range 00064 humidity = rh_true; 00065 pc.printf("stat=%d Temp: %2.2f RH %2.2f\n\r",stat, temperature, humidity); 00066 00067 wait(3.0); 00068 } 00069 }
Generated on Wed Jul 13 2022 18:43:57 by
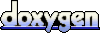