Ohms law basic calculations for calculating R2 in voltage divider when R1 is known and to calculate voltage drop ratio when both R1 and R2 are known.
diode_current_flow.h
00001 /* Compute Current Flow across Diode 00002 using voltage drop across the diode. 00003 00004 By Joseph Ellsworth CTO of A2WH 00005 Take a look at A2WH.com Producing Water from Air using Solar Energy 00006 March-2016 License: https://developer.mbed.org/handbook/MIT-Licence 00007 Please contact us http://a2wh.com for help with custom design projects. 00008 00009 00010 Based on voltage drop across a diode calculate 00011 current flowing based on known characteristics of that diode. 00012 Unlike a resistor this can not be done with straigt ohms law 00013 because it is temperature specific and is not linear. Instead we 00014 use a lookup table and aproximate between elements to compute 00015 a value. 00016 00017 A known limitation is that if voltage drop is equal or lower 00018 than smallest value in vDropArr Then all we know is that current is some place 00019 betweeen 0 and that minimum current number. Since all diodes have a minimum drop 00020 for any current flow they are not as effective when measuring very small currents. 00021 The diodes are best used when we do not want to add the 00022 loss of a resistor and we needed the diode in circuit anyway especially when 00023 there may be large current flow such as in a charging circuit. 00024 00025 If you need a temperature specific value then declare a vDrop for multiple temperature ranges 00026 then compute the voltage drop for the temperature range above and below current temp and 00027 use ratio level computation to compute a weighted average between the two. 00028 00029 Note: This would be a perfect place to use a differential ADC where 00030 the one line is on the postive side of the Diode and the other is 00031 on the load side. Unfortunately mbed doesn't provide standard support 00032 for the differential. 00033 00034 Note: Turn off the PWM for the charge circuit and turn the charge 00035 circuit all the way on before taking this reading or it mess up the ADC readings. 00036 00037 */ 00038 00039 //#include "ohms.h" 00040 00041 #ifndef diode_current_flow_H 00042 #define diode_current_flow_H 00043 // T.I SM74611 Smart Bypass Diode 00044 const float SM74611VDrop85C[] = {0.020, 0.024, 0.037, 0.040, 0.050, 0.057, 0.060, 0.075, 0.080, 0.090, 0.098, 0.106, -1}; 00045 const float SM74611Current[] = {2.000, 4.000, 6.00, 8.000, 10.000, 12.000, 14.000, 16.000, 18.000, 20.000, 22.000,24.000, -1}; 00046 // must be oriented as lowest current flow (lowest vdrop) to highest, must be terminated with -1 and arrays 00047 // must line up by postion. The more numbers supplied the more accurate the computation will be down to the 00048 // limit of the ADC. 00049 00050 // NOTE: DEFINE your own diode perfornce characteristics from the Diode datasheet. They vary a lot and 00051 // the SM74611 is a very special diode with very low vdrop so these numbers will not work for other diodes. 00052 00053 00054 00055 /* compute the current flowing across the diode based on the known diode 00056 characteristics and a known voltage drop across the diode The two arrays 00057 must both contain floats, must be the same length and must be terminated with -1.0 00058 sentinal. 00059 00060 */ 00061 float ComputeDiodeCurrent(float *vDropArr, float *currentArr, float vDrop) { 00062 int lastNdx = 0; 00063 while (vDropArr[lastNdx] != -1.0) lastNdx++; 00064 lastNdx--; 00065 00066 // find first vdrop in array that is less than our specified 00067 // vdrop which gives us a range where our vDrop must be so we 00068 // than then interpolate a current across the diode. 00069 if (vDrop >= vDropArr[lastNdx]) return currentArr[lastNdx]; // above our greatest setting 00070 00071 int ndx = lastNdx; 00072 while ((vDrop < vDropArr[ndx]) && (ndx > 0)) 00073 ndx--; 00074 00075 if (ndx == 0) return currentArr[0]; // below our lowest setting 00076 if (vDropArr[ndx] == vDrop) return currentArr[ndx]; // exact match 00077 00078 // Interpolate the ratio we are between the two points found 00079 // and use that to determine our actual current flow. This only 00080 // works assuming it is a relatively straight line between the two 00081 // points. If that is not true then you need to add more points. 00082 float vrange = vDropArr[ndx + 1] - vDropArr[ndx]; 00083 float amtOverLower = vDrop - vDropArr[ndx]; 00084 float portRange = amtOverLower / vrange; 00085 float currRange = currentArr[ndx + 1] - currentArr[ndx]; 00086 float lowCurr = currentArr[ndx]; 00087 float portCurAdd = currRange * portRange; 00088 return lowCurr + portCurAdd; 00089 } 00090 #endif
Generated on Fri Jul 22 2022 01:55:13 by
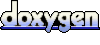