interface library to support TI hdc1080 humidity and temperature sensor
Dependents: xj-Nucleo-F303K8-hdc1080-test HelloWorld_NFC02A1laatste
hdc1080.h
00001 /* 00002 By Joseph Ellsworth CTO of A2WH 00003 Take a look at A2WH.com Producing Water from Air using Solar Energy 00004 March-2016 License: https://developer.mbed.org/handbook/MIT-Licence 00005 Please contact us http://a2wh.com for help with custom design projects. 00006 00007 See Also: 00008 http://www.ti.com/lit/ds/symlink/hdc1080.pdf 00009 https://github.com/closedcube/ClosedCube_HDC1080_Arduino/blob/master/src/ClosedCube_HDC1080.cpp 00010 http://e.pavlin.si/2016/06/04/hdlc-like-data-link-via-rs485/ 00011 */ 00012 00013 /** 00014 #include "mbed.h" 00015 #include <stdint.h> 00016 00017 //Pin Defines for I2C Bus 00018 #define D_SDA PB_7 // specific for Nucleo-F303K8 00019 #define D_SCL PB_6 // specific for Nucleo-F303K8 00020 I2C hdc_i2c(D_SDA, D_SCL); 00021 #include "hdc1080.h" 00022 00023 // Host PC Communication channels 00024 Serial pc(USBTX, USBRX); // tx, rx 00025 DigitalOut myled(LED1); 00026 00027 int main() 00028 { 00029 pc.baud(9600); 00030 while(1) { 00031 printf("\r\\nHDC1080 Test\r\n"); 00032 myled = !myled; 00033 hdc_begin(); 00034 uint16_t manId = hdc_readManufactId(); 00035 float tempC = hdc_readTemp(); 00036 float humid = hdc_readHumid(); 00037 unsigned long serNum = hdc_readSerial(); 00038 printf("manId=x%x, tempC=%0.3f humid=%0.3f serfNum=%ld\r\n", 00039 manId, tempC, humid, serNum); 00040 wait(3.0f); 00041 } 00042 } 00043 **/ 00044 00045 00046 00047 #ifndef hdc1080_h 00048 #define hdc1080_h 00049 #include "mbed.h" 00050 00051 #define hdc_chip_addr (0x40 << 1) // Page #8.5.1.1 - 1000000 00052 // left shift 1 bit for 7 bit address required by 00053 // I2C library 00054 //#define hdc_chip_addr 0x40 // Page #8.5.1.1 - 1000000 00055 const int hdc_off_temp = 0x00; 00056 const int hdc_off_humid = 0x01; 00057 const int hdc_off_config = 0x02; 00058 const int hdc_off_man_id = 0xFE; 00059 const int hdc_off_serial_first = 0xFB; 00060 const int hdc_off_serial_mid = 0xFC; 00061 const int hdc_off_serial_last = 0xFD; 00062 char hdc_comm = hdc_off_man_id; 00063 const float hdc_chip_error = -255; 00064 const unsigned long hdc_chip_err_l = 0; 00065 char hdc_buff[5]; 00066 00067 void hdc_begin() 00068 { 00069 //printf("hdc_begin\r\n"); 00070 memset(hdc_buff,0,3); 00071 hdc_buff[0] = hdc_off_config; 00072 int res = hdc_i2c.write(hdc_chip_addr, hdc_buff, 2); 00073 #ifdef DEBUG3 00074 printf("hdc_begin write res=%d\r\n", res); 00075 #endif 00076 } 00077 00078 uint16_t hdc_readData16(int chip_addr, int offset) 00079 { 00080 memset(hdc_buff,0,3); 00081 // send chip address onto buss 00082 hdc_buff[0] = offset; 00083 int res = hdc_i2c.write(chip_addr, hdc_buff, 1); 00084 if (res != 0) { 00085 #ifdef DEBUG3 00086 printf("error talking to chip %d offst=%d\r\n", chip_addr, offset); 00087 #endif 00088 return 0; 00089 } 00090 // read data from chip 00091 wait(0.015); 00092 memset(hdc_buff,0,3); 00093 res = hdc_i2c.read(hdc_chip_addr, hdc_buff,2); 00094 if (res != 0) { 00095 #ifdef DEBUG3 00096 printf("error reading chip %d offst=%d\r\n", chip_addr, offset); 00097 #endif 00098 return 0; 00099 } 00100 return hdc_buff[0] << 8 | hdc_buff[1]; 00101 } 00102 00103 /* Read temperature from hdc_1080 chip. Returns float 00104 containing the Celcius temperature or hdc_chip_error if 00105 error occurs reading the sensor */ 00106 float hdc_readTemp() 00107 { 00108 uint16_t rawT = hdc_readData16(hdc_chip_addr, hdc_off_temp); 00109 if (rawT == 0) { 00110 #ifdef DEBUG3 00111 printf("error reading hdc chip temp\r\n"); 00112 #endif 00113 return hdc_chip_error; 00114 } else { 00115 float temp = ((float) rawT / pow(2.0f, 16.0f)) * 165.0f - 40.0f; 00116 #ifdef DEBUG3 00117 printf("temp=%0.3f\r\n", temp); 00118 #endif 00119 return temp; 00120 } 00121 } 00122 00123 /* Read humidity from hdc_1080 chip. Returns a float 00124 containing the humidity or hdc_chip_error if error 00125 occurs reading the sensor */ 00126 float hdc_readHumid() 00127 { 00128 uint16_t rawH = hdc_readData16(hdc_chip_addr, hdc_off_humid); 00129 if (rawH == 0) { 00130 #ifdef DEBUG3 00131 printf("error reading hdc chip humid\r\n"); 00132 #endif 00133 return hdc_chip_error; 00134 } else { 00135 float humid = ((float) rawH / pow(2.0f, 16.0f)) * 100.0f; 00136 #ifdef DEBUG3 00137 printf("humid humid=%0.3f\r\n", humid); 00138 #endif 00139 return humid; 00140 } 00141 } 00142 00143 int hdc_readManufactId() 00144 { 00145 uint16_t rawid = hdc_readData16(hdc_chip_addr, hdc_off_man_id); 00146 if (rawid == 0) { 00147 #ifdef DEBUG3 00148 printf("error reading hdc chip manId\r\n"); 00149 #endif 00150 return (int) hdc_chip_error; 00151 } else { 00152 #ifdef DEBUG3 00153 printf("man id=%x\r\n", (int) rawid); 00154 #endif 00155 return rawid; 00156 } 00157 } 00158 00159 unsigned long hdc_readSerial() 00160 { 00161 wait(0.015); 00162 memset(hdc_buff,0,4); 00163 hdc_buff[0] = hdc_off_man_id; 00164 int res = hdc_i2c.write(hdc_chip_addr, hdc_buff, 1); 00165 if (res != 0) { 00166 #ifdef DEBUG3 00167 printf("Error writing chip addr res=%d\r\n", res); 00168 #endif 00169 return (unsigned long) hdc_chip_err_l; 00170 } 00171 00172 wait(0.015); 00173 memset(hdc_buff,0,4); 00174 res = hdc_i2c.read(hdc_chip_addr, hdc_buff,4); 00175 if (res != 0) { 00176 #ifdef DEBUG3 00177 printf("Errot reading chip serial res=%d#\r\n", res); 00178 #endif 00179 return (unsigned long) hdc_chip_err_l; 00180 } 00181 00182 unsigned long rawser = hdc_buff[0] << 16 | hdc_buff[1] << 8 | hdc_buff[0]; 00183 #ifdef DEBUG3 00184 printf("ser=%lu\r\n", rawser); 00185 #endif 00186 return rawser; 00187 } 00188 00189 #endif
Generated on Thu Jul 14 2022 01:02:13 by
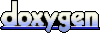