Measurement of low frequencys based on timing between pulses
Dependents: Energy_Meter_S0_Example
Pulses Class Reference
A class to calculate frequencys based on timing between pulses. More...
#include <Pulses.h>
Public Types | |
enum | PulseType |
Pulses type format. More... | |
Public Member Functions | |
Pulses (PinName inPin, PulseType type=FALL, unsigned int timeout=600, unsigned int counter=0) | |
constructor of Pulses object | |
float | getAct () |
Gets the frequency based on the last 2 pulses. | |
float | getAverage () |
Gets the average of frequency based on all pulses since last call of this function. | |
void | get (float *pAverage, float *pMin, float *pMax, float *pSum=NULL) |
Gets the average, min, max and sum of frequency based on all pulses since last call of this function. | |
unsigned int | getCounter () |
Gets the number of pulses from the input pin since start. | |
void | setFactor (float factor) |
Sets the factor for the getter-functions to convert in another unit (1.0=Hz, 60.0=rpm, ...) |
Detailed Description
A class to calculate frequencys based on timing between pulses.
Pulse-frequency can be about or slower than loop/aquisition time
Example:
#include "mbed.h" #include "Pulses.h" Pulses pulses(p8, Pulses::FALL); Serial pc(USBTX, USBRX); // tx, rx int main() { // choose on of the following unit scales pulses.setFactor(1.0f); // Hz pulses.setFactor(60.0f); // rpm pulses.setFactor(3600.0f/2000.0f); // kWh; energy meter with SO interface - 2000 pulses per kWh while(1) { pc.printf ( "Pulses: act=%.3f average=%.3f counter=%d \r\n", pulses.getAct(), pulses.getAverage(), pulses.getCounter() ); wait(3.14); } }
Definition at line 67 of file Pulses.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
Pulses | ( | PinName | inPin, |
PulseType | type = FALL , |
||
unsigned int | timeout = 600 , |
||
unsigned int | counter = 0 |
||
) | [explicit] |
constructor of Pulses object
- Parameters:
-
inPin Pin number of input pin. All port pins are possible except p19 and p20 type Type of edge detection. RISE, FALL, CHANGE timeout Timeout in seconds to handle a stopped pulse-generator (standing motor). Max 15 minutes. counter Start value of the internal pulses counter to offset pSum (in get()) e.g. after reboot
Definition at line 5 of file Pulses.cpp.
Member Function Documentation
void get | ( | float * | pAverage, |
float * | pMin, | ||
float * | pMax, | ||
float * | pSum = NULL |
||
) |
Gets the average, min, max and sum of frequency based on all pulses since last call of this function.
- Parameters:
-
pAverage Pointer to float value to return average frequency. Use NULL to skip param. -1 if no new pulses occurred since last call. pMin Pointer to float value to return min. frequency. Use NULL to skip param. -1 if no new pulses occurred since last call. pMax Pointer to float value to return max. frequency. Use NULL to skip param. -1 if no new pulses occurred since last call. pSum Pointer to float value to return the accumulated average values. Use NULL to skip param.
Definition at line 65 of file Pulses.cpp.
float getAct | ( | ) |
Gets the frequency based on the last 2 pulses.
It's only a snapshot and its not representative.
- Returns:
- Actual frequency
Definition at line 39 of file Pulses.cpp.
float getAverage | ( | ) |
Gets the average of frequency based on all pulses since last call of this function.
- Returns:
- Average frequency. -1 if no new pulses occurred since last call
Definition at line 47 of file Pulses.cpp.
unsigned int getCounter | ( | ) |
Gets the number of pulses from the input pin since start.
- Returns:
- Number of pulses
Definition at line 112 of file Pulses.cpp.
void setFactor | ( | float | factor ) |
Sets the factor for the getter-functions to convert in another unit (1.0=Hz, 60.0=rpm, ...)
- Parameters:
-
factor Factor to scale from Hz to user unit
Definition at line 118 of file Pulses.cpp.
Generated on Wed Jul 27 2022 14:17:37 by
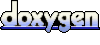