
WebAPIサンプルプログラム mbedセミナー演習5
Dependencies: EthernetNetIf TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "TextLCD.h" 00004 #include "HTTPClient.h" 00005 00006 TextLCD lcd(p24, p26, p27, p28, p29, p30); // rs, e, d4-d7 00007 00008 EthernetNetIf eth; 00009 HTTPClient http; 00010 00011 int j_paser( const char *buf , char *word , char *out ) 00012 { 00013 int i = 0; 00014 char *p; 00015 char _word[64] = "\"\0"; 00016 00017 strcat(_word , word ); 00018 strcat(_word , "\"" ); 00019 00020 p = strstr( (char*)buf , _word ) + 2 + strlen(_word); 00021 00022 while( (p[i] != ',')&&(p[i] != '\n') ) 00023 { 00024 out[i] = p[i]; 00025 i++; 00026 } 00027 out[i] = '\0'; 00028 00029 return(i); 00030 } 00031 00032 int main(void) { 00033 00034 char year[32]; 00035 char month[32]; 00036 char day[32]; 00037 char hour[32]; 00038 char usage[32]; 00039 char capacity[32]; 00040 00041 EthernetErr ethErr = eth.setup(); 00042 if(ethErr) 00043 { 00044 lcd.locate(0,0); 00045 lcd.printf("Ethernet err."); 00046 return(-1); 00047 } 00048 00049 lcd.locate(0,0); 00050 lcd.printf("Getting info."); 00051 00052 HTTPText txt; 00053 00054 HTTPResult r = http.get("http://tepco-usage-api.appspot.com/latest.json", &txt); 00055 00056 if(r==HTTP_OK) 00057 { 00058 j_paser(txt.gets() , "year" , year); 00059 j_paser(txt.gets() , "month" , month); 00060 j_paser(txt.gets() , "day" , day); 00061 j_paser(txt.gets() , "hour" , hour); 00062 j_paser(txt.gets() , "usage" , usage); 00063 j_paser(txt.gets() , "capacity" , capacity); 00064 00065 lcd.locate(0,0); 00066 lcd.printf("%s/%s/%s %sh",year,month,day,hour); 00067 lcd.locate(0,1); 00068 lcd.printf("%s/%s %3.1f%%",usage,capacity,(((float)atoi(usage)/(float)atoi(capacity))*100.0)); 00069 } 00070 else 00071 { 00072 lcd.locate(0,0); 00073 lcd.printf("Http get err."); 00074 return(-1); 00075 } 00076 00077 while(1) 00078 { 00079 00080 } 00081 00082 return(0); 00083 }
Generated on Wed Jul 20 2022 05:41:51 by
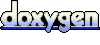