Fork of original mRotaryEncoder library supporting more types of encoders (ones with full rise and fall cycle per one rotations).
mRotaryEncoder Class Reference
This Class handles a rotary encoder with mechanical switches and an integrated pushbutton It uses two pins, one creating an interrupt on change. More...
#include <mRotaryEncoder.h>
Public Member Functions | |
mRotaryEncoder (PinName pinA, PinName pinB, PinName pinSW, PinMode pullMode=PullUp, int debounceTime_us=1000, int detectRise=1, int detectFall=1) | |
Create a mechanical rotary encoder object connected to the specified pins. | |
~mRotaryEncoder () | |
destroy object | |
int | Get (void) |
Get the actual value of the rotary position. | |
void | Set (int value) |
Set the current position value. | |
void | attachSW (void(*fptr)(void)) |
attach a function to be called when switch is pressed | |
template<typename T > | |
void | attachSW (T *tptr, void(T::*mptr)(void)) |
attach an object member function to be called when switch is pressed | |
void | attachROT (void(*fptr)(void)) |
callback-System for rotation of shaft | |
template<typename T > | |
void | attachROT (T *tptr, void(T::*mptr)(void)) |
attach an object member function to be called when shaft is rotated | |
void | attachROTCW (void(*fptr)(void)) |
callback-System for rotation of shaft CW | |
template<typename T > | |
void | attachROTCW (T *tptr, void(T::*mptr)(void)) |
attach an object member function to be called when shaft is rotated clockwise | |
void | attachROTCCW (void(*fptr)(void)) |
callback-System for rotation of shaft CCW | |
template<typename T > | |
void | attachROTCCW (T *tptr, void(T::*mptr)(void)) |
attach an object member function to be called when shaft is rotated CCW | |
Protected Attributes | |
FunctionPointer | rotIsr |
Callback system. | |
FunctionPointer | rotCWIsr |
clockwise rotated | |
FunctionPointer | rotCCWIsr |
counterclockwise rotated |
Detailed Description
This Class handles a rotary encoder with mechanical switches and an integrated pushbutton It uses two pins, one creating an interrupt on change.
Rotation direction is determined by checking the state of the other pin. Additionally a pushbutton switch is detected
Operating the encoder changes an internal integer value that can be read by Get() or the operator int() functions. A new value can be set by Set(value) or opperator=.
Autor: Thomas Raab (Raabinator) Extended by Karl Zweimueller (charly)
Dent steady point ! ! ! +-----+ +-----+ pinA (interrupt) | | | | --+ +-----+ +--- +-----+ +-----+ pinB | | | | ----+ +-----+ +- --> C.W CW: increases position value CCW: decreases position value
changelog:
09. Nov. 2010 First version published Thomas Raab raabinator 26.11.2010 extended by charly - pushbutton, pullmode, debounce, callback-system Feb2011 Changes InterruptIn to PinDetect which does the debounce of mechanical switches Mar2020 Configurable detection of rise/fall events to account for different types of encoders (half as much dent points)
Definition at line 40 of file mRotaryEncoder.h.
Constructor & Destructor Documentation
mRotaryEncoder | ( | PinName | pinA, |
PinName | pinB, | ||
PinName | pinSW, | ||
PinMode | pullMode = PullUp , |
||
int | debounceTime_us = 1000 , |
||
int | detectRise = 1 , |
||
int | detectFall = 1 |
||
) |
Create a mechanical rotary encoder object connected to the specified pins.
- Parameters:
-
pinA Switch A of quadrature encoder pinB Switch B of quadrature encoder pinSW Pin for push-button switch pullmode mode for pinA pinB and pinSW like DigitalIn.mode debounceTime_us time in micro-seconds to wait for bouncing of mechanical switches to end detectRise Detect rise event as new rotation detectFall Detect fall event as new rotation
Definition at line 5 of file mRotaryEncoder.cpp.
~mRotaryEncoder | ( | ) |
destroy object
Definition at line 36 of file mRotaryEncoder.cpp.
Member Function Documentation
void attachROT | ( | void(*)(void) | fptr ) |
callback-System for rotation of shaft
attach a function to be called when the shaft is rotated keep this function short, as no interrrupts can occour within
- Parameters:
-
fprt Pointer to callback-function
Definition at line 109 of file mRotaryEncoder.h.
void attachROT | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
attach an object member function to be called when shaft is rotated
- Parameters:
-
tptr pointer to object mprt pointer ro member function
Definition at line 121 of file mRotaryEncoder.h.
void attachROTCCW | ( | void(*)(void) | fptr ) |
callback-System for rotation of shaft CCW
attach a function to be called when the shaft is rotated counterclockwise keep this function short, as no interrrupts can occour within
- Parameters:
-
fprt Pointer to callback-function
Definition at line 159 of file mRotaryEncoder.h.
void attachROTCCW | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
attach an object member function to be called when shaft is rotated CCW
- Parameters:
-
tptr pointer to object mprt pointer ro member function
Definition at line 171 of file mRotaryEncoder.h.
void attachROTCW | ( | void(*)(void) | fptr ) |
callback-System for rotation of shaft CW
attach a function to be called when the shaft is rotated clockwise keep this function short, as no interrrupts can occour within
- Parameters:
-
fprt Pointer to callback-function
Definition at line 134 of file mRotaryEncoder.h.
void attachROTCW | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
attach an object member function to be called when shaft is rotated clockwise
- Parameters:
-
tptr pointer to object mprt pointer ro member function
Definition at line 146 of file mRotaryEncoder.h.
void attachSW | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
attach an object member function to be called when switch is pressed
- Parameters:
-
tptr pointer to object mprt pointer ro member function
Definition at line 96 of file mRotaryEncoder.h.
void attachSW | ( | void(*)(void) | fptr ) |
attach a function to be called when switch is pressed
keep this function short, as no interrrupts can occour within
- Parameters:
-
fptr Pointer to callback-function
Definition at line 85 of file mRotaryEncoder.h.
int Get | ( | void | ) |
Get the actual value of the rotary position.
- Returns:
- position int value of position
Definition at line 42 of file mRotaryEncoder.cpp.
void Set | ( | int | value ) |
Set the current position value.
- Parameters:
-
value the new position to set
Definition at line 48 of file mRotaryEncoder.cpp.
Field Documentation
FunctionPointer rotCCWIsr [protected] |
counterclockwise rotated
Definition at line 207 of file mRotaryEncoder.h.
FunctionPointer rotCWIsr [protected] |
clockwise rotated
Definition at line 202 of file mRotaryEncoder.h.
FunctionPointer rotIsr [protected] |
Generated on Tue Jul 12 2022 19:37:44 by
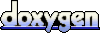