C Library for mbedWSE project based single board computer for hardware peripherals
Dependents: Lab7_wse Lab7_wse_student mbed_WSEPRJSBC_EncoderTest mbed_WSEPRJSBC_ADC_test ... more
mbedWSEsbc.h
00001 /* C Library for the WSE-PROJ-SBC 00002 J Bradshaw 00003 20140912 00004 20140918 J Bradshaw - Found CS mistake in Encoder routines 00005 Added comments in Init function, encoder functions 00006 20150210 J Bradshaw - Initialized DigitalOuts with pre-defined logic 00007 levels (CS's high, etc) 00008 20161011 J Bradshaw - Changed MAX1270 ADC SCLK to 5MHz and format(12, 0) 00009 for conversion mode to match datasheet (200ns SCLK max PW high and low) 00010 */ 00011 00012 00013 // LS7366 ENCODER IC DEFINITIONS 00014 //============================================================================= 00015 // Four commands for the Instruction Register (B7,B6) - LS7366 00016 //============================================================================= 00017 #define CLR 0x00 //Clear Instruction 00018 #define RD 0x01 //Read Instruction 00019 #define WR 0x02 //Write Instruction 00020 #define LOAD 0x03 //Load Instruction 00021 00022 //============================================================================= 00023 // Register to Select from the Instruction Register (B5,B4,B3) - LS7366 00024 //============================================================================= 00025 #define NONE 0x00 //No Register Selected 00026 #define MDR0 0x01 //Mode Register 0 00027 #define MDR1 0x02 //Mode Register 1 00028 #define DTR 0x03 //Data Transfer Register 00029 #define CNTR 0x04 //Software Configurable Counter Register 00030 #define OTR 0x05 //Output Transfer Register 00031 #define STR 0x06 //Status Register 00032 #define NONE_REG 0x07 //No Register Selected 00033 00034 // Set-up hardwired IO 00035 SPI spi_max1270(p5, p6, p7); 00036 SPI spi(p5, p6, p7); 00037 DigitalOut max1270_cs(p8, 1); //CS for MAX1270 ADC (U3) 00038 DigitalOut max522_cs(p11, 1); //CS for MAX522 DAC (U5) 00039 00040 DigitalOut ls7166_cs1(p19, 1); //CS for LS7366-1 (U8) 00041 DigitalOut ls7166_cs2(p20, 1); //CS for LS7366-2 (U9) 00042 00043 DigitalOut mot1_ph1(p21, 0); 00044 DigitalOut mot1_ph2(p22, 0); 00045 PwmOut mot_en1(p23); 00046 00047 DigitalOut mot2_ph1(p24, 0); 00048 DigitalOut mot2_ph2(p25, 0); 00049 PwmOut mot_en2(p26); 00050 00051 DigitalOut led1(LED1, 0); 00052 DigitalOut led2(LED2, 0); 00053 DigitalOut led3(LED3, 0); 00054 DigitalOut led4(LED4, 0); 00055 00056 Serial pc(USBTX, USBRX); // tx, rx for serial USB interface to pc 00057 Serial xbee(p13, p14); // tx, rx for Xbee 00058 Timer t; // create timer instance 00059 00060 // ------ Prototypes ----------- 00061 int read_max1270(int chan, int range, int bipol); 00062 float read_max1270_volts(int chan, int range, int bipol); 00063 void mot_control(int drv_num, float dc); 00064 void LS7366_cmd(int inst, int reg); 00065 long LS7366_read_counter(int chan_num); 00066 void LS7366_quad_mode_x4(int chan_num); 00067 void LS7366_reset_counter(int chan_num); 00068 void LS7366_write_DTR(int chan_num,long enc_value); 00069 void write_max522(int chan, float volts); 00070 00071 //---- Function Listing ------------------------------- 00072 int read_max1270(int chan, int range, int bipol){ 00073 int cword=0x80; //set the start bit 00074 00075 spi_max1270.frequency(5000000); //5MHz Max 00076 spi_max1270.format(8, 0); // 8 data bits, CPOL0, and CPHA0 (datasheet Digital Interface) 00077 00078 cword |= (chan << 4); //shift channel 00079 cword |= (range << 3); 00080 cword |= (bipol << 2); 00081 00082 max1270_cs = 0; 00083 00084 spi_max1270.write(cword); 00085 wait_us(15); //15us 00086 spi_max1270.format(12, 0); 00087 00088 int result = spi_max1270.write(0); 00089 00090 max1270_cs = 1; 00091 spi_max1270.format(8, 0); 00092 return result; 00093 } 00094 00095 float read_max1270_volts(int chan, int range, int bipol){ 00096 float rangevolts=0.0; 00097 float volts=0.0; 00098 int adc_res; 00099 00100 //read the ADC converter 00101 adc_res = read_max1270(chan, range, bipol) & 0xFFF; 00102 00103 //Determine the voltage range 00104 if(range) //RNG bit 00105 rangevolts=10.0; 00106 else 00107 rangevolts=5.0; 00108 00109 //bi-polar input range 00110 if(bipol){ //BIP is set, input is +/- 00111 if(adc_res < 0x800){ //if result was positive 00112 volts = ((float)adc_res/0x7FF) * rangevolts; 00113 } 00114 else{ //result was negative 00115 volts = -(-((float)adc_res/0x7FF) * rangevolts) - (rangevolts * 2.0); 00116 } 00117 } 00118 else{ //input is positive polarity only 00119 volts = ((float)adc_res/0xFFF) * rangevolts; 00120 } 00121 00122 return volts; 00123 } 00124 00125 //Motor control routine for PWM on 5 pin motor driver header 00126 // drv_num is 1 or 2 (defaults to 1, anything but 2) 00127 // dc is signed duty cycle (+/-1.0) 00128 00129 void mot_control(int drv_num, float dc){ 00130 if(dc>1.0) 00131 dc=1.0; 00132 if(dc<-1.0) 00133 dc=-1.0; 00134 00135 if(drv_num != 2){ 00136 if(dc > 0.0){ 00137 mot1_ph2 = 0; 00138 mot1_ph1 = 1; 00139 mot_en1 = dc; 00140 } 00141 else if(dc < -0.0){ 00142 mot1_ph1 = 0; 00143 mot1_ph2 = 1; 00144 mot_en1 = abs(dc); 00145 } 00146 else{ 00147 mot1_ph1 = 0; 00148 mot1_ph2 = 0; 00149 mot_en1 = 0.0; 00150 } 00151 } 00152 else{ 00153 if(dc > 0.0){ 00154 mot2_ph2 = 0; 00155 mot2_ph1 = 1; 00156 mot_en2 = dc; 00157 } 00158 else if(dc < -0.0){ 00159 mot2_ph1 = 0; 00160 mot2_ph2 = 1; 00161 mot_en2 = abs(dc); 00162 } 00163 else{ 00164 mot2_ph1 = 0; 00165 mot2_ph2 = 0; 00166 mot_en2 = 0.0; 00167 } 00168 } 00169 } 00170 00171 //----- LS7366 Encoder/Counter Routines -------------------- 00172 void LS7366_cmd(int inst, int reg){ 00173 char cmd; 00174 00175 spi.format(8, 0); 00176 spi.frequency(2000000); 00177 cmd = (inst << 6) | (reg << 3); 00178 // printf("\r\ncmd=0X%2X", cmd); 00179 spi.write(cmd); 00180 } 00181 00182 long LS7366_read_counter(int chan_num){ 00183 union bytes{ 00184 char byte_enc[4]; 00185 long long_enc; 00186 }counter; 00187 00188 counter.long_enc = 0; 00189 00190 spi.format(8, 0); 00191 spi.frequency(2000000); 00192 00193 if(chan_num!=2){ 00194 ls7166_cs1 = 0; 00195 wait_us(1); 00196 LS7366_cmd(LOAD,OTR);//cmd = 0xe8, LOAD to OTR 00197 ls7166_cs1 = 1; 00198 wait_us(1); 00199 ls7166_cs1 = 0; 00200 } 00201 else{ 00202 ls7166_cs2 = 0; 00203 wait_us(1); 00204 LS7366_cmd(LOAD,OTR);//cmd = 0xe8, LOAD to OTR 00205 ls7166_cs2 = 1; 00206 wait_us(1); 00207 00208 ls7166_cs2 = 0; 00209 } 00210 wait_us(1); 00211 LS7366_cmd(RD,CNTR); //cmd = 0x60, READ from CNTR 00212 counter.byte_enc[3] = spi.write(0x00); 00213 counter.byte_enc[2] = spi.write(0x00); 00214 counter.byte_enc[1] = spi.write(0x00); 00215 counter.byte_enc[0] = spi.write(0x00); 00216 00217 if(chan_num!=2){ 00218 ls7166_cs1 = 1; 00219 } 00220 else{ 00221 ls7166_cs2 = 1; 00222 } 00223 00224 return counter.long_enc; //return count 00225 } 00226 00227 void LS7366_quad_mode_x4(int chan_num){ 00228 00229 spi.format(8, 0); 00230 spi.frequency(2000000); 00231 00232 if(chan_num!=2){ 00233 ls7166_cs1 = 0; 00234 } 00235 else{ 00236 ls7166_cs2 = 0; 00237 } 00238 wait_us(1); 00239 LS7366_cmd(WR,MDR0);// Write to the MDR0 register 00240 wait_us(1); 00241 spi.write(0x03); // X4 quadrature count mode 00242 if(chan_num!=2){ 00243 ls7166_cs1 = 1; 00244 } 00245 else{ 00246 ls7166_cs2 = 1; 00247 } 00248 } 00249 00250 void LS7366_reset_counter(int chan_num){ 00251 spi.format(8, 0); // set up SPI for 8 data bits, mode 0 00252 spi.frequency(2000000); // 2MHz SPI clock 00253 00254 if(chan_num!=2){ // activate chip select 00255 ls7166_cs1 = 0; 00256 } 00257 else{ 00258 ls7166_cs2 = 0; 00259 } 00260 wait_us(1); // short delay 00261 LS7366_cmd(CLR,CNTR); // Clear the counter register 00262 if(chan_num!=2){ // de-activate chip select 00263 ls7166_cs1 = 1; 00264 } 00265 else{ 00266 ls7166_cs2 = 1; 00267 } 00268 wait_us(1); // short delay 00269 00270 if(chan_num!=2){ // activate chip select 00271 ls7166_cs1 = 0; 00272 } 00273 else{ 00274 ls7166_cs2 = 0; 00275 } 00276 wait_us(1); // short delay 00277 LS7366_cmd(LOAD,CNTR); // load counter reg 00278 if(chan_num!=2){ // de-activate chip select 00279 ls7166_cs1 = 1; 00280 } 00281 else{ 00282 ls7166_cs2 = 1; 00283 } 00284 } 00285 00286 void LS7366_write_DTR(int chan_num, long enc_value){ 00287 union bytes // Union to speed up byte writes 00288 { 00289 char byte_enc[4]; 00290 long long_enc; 00291 }counter; 00292 00293 spi.format(8, 0); // set up SPI for 8 data bits, mode 0 00294 spi.frequency(2000000); // 2MHz SPI clock 00295 00296 counter.long_enc = enc_value; // pass enc_value to Union 00297 00298 if(chan_num!=2){ // activate chip select 00299 ls7166_cs1 = 0; 00300 } 00301 else{ 00302 ls7166_cs2 = 0; 00303 } 00304 wait_us(1); // short delay 00305 LS7366_cmd(WR,DTR); // Write to the Data Transfer Register 00306 spi.write(counter.byte_enc[3]); // Write the 32-bit encoder value 00307 spi.write(counter.byte_enc[2]); 00308 spi.write(counter.byte_enc[1]); 00309 spi.write(counter.byte_enc[0]); 00310 if(chan_num!=2){ // de-activate the chip select 00311 ls7166_cs1 = 1; 00312 } 00313 else{ 00314 ls7166_cs2 = 1; 00315 } 00316 00317 wait_us(1); // short delay 00318 if(chan_num!=2){ // activate chip select 00319 ls7166_cs1 = 0; 00320 } 00321 else{ 00322 ls7166_cs2 = 0; 00323 } 00324 wait_us(1); // short delay 00325 LS7366_cmd(LOAD,CNTR); // load command to the counter register from DTR 00326 if(chan_num!=2){ // de-activate chip select 00327 ls7166_cs1 = 1; 00328 } 00329 else{ 00330 ls7166_cs2 = 1; 00331 } 00332 } 00333 00334 //------- MAX522 routines --------------------------------- 00335 void write_max522(int chan, float volts){ 00336 int cmd=0x20; //set UB3 00337 int data_word = (int)((volts/5.0) * 256.0); 00338 if(chan != 2) 00339 cmd |= 0x01; //set DAC A out 00340 else 00341 cmd |= 0x02; //set DACB out 00342 00343 // pc.printf("cmd=0x%4X data_word=0x%4X \r\n", cmd, data_word); 00344 00345 spi.format(8, 0); 00346 spi.frequency(2000000); 00347 max522_cs = 0; 00348 spi.write(cmd & 0xFF); 00349 spi.write(data_word & 0xFF); 00350 max522_cs = 1; 00351 } 00352 00353 void mbedWSEsbcInit(unsigned long pcbaud){ 00354 led1 = 0; //Initialize all LEDs as off 00355 led2 = 0; 00356 led3 = 0; 00357 led4 = 0; 00358 max1270_cs = 1; //Initialize all chip selects as off 00359 max522_cs = 1; 00360 ls7166_cs1 = 1; 00361 ls7166_cs2 = 1; 00362 00363 wait(.2); //delay at beginning for voltage settle purposes 00364 00365 mot_en1.period_us(50); //20KHz for DC motor control PWM 00366 pc.baud(pcbaud); //Set up serial port baud rate 00367 pc.printf("\r\n"); 00368 xbee.baud(9600); 00369 00370 LS7366_reset_counter(1); 00371 LS7366_quad_mode_x4(1); 00372 LS7366_write_DTR(1,0); 00373 00374 LS7366_reset_counter(2); 00375 LS7366_quad_mode_x4(2); 00376 LS7366_write_DTR(2,0); 00377 00378 t.start(); // Set up timer 00379 }//mbedWSEsbc_init()
Generated on Thu Jul 21 2022 05:24:47 by
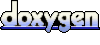