Timer for accumulating 10 ms intervals that does not overflow after ~30 min
Embed:
(wiki syntax)
Show/hide line numbers
RunTimer.cpp
00001 #include "RunTimer.h" 00002 00003 RunTimer::RunTimer(){ 00004 this->ms=0; 00005 this->sec =0; 00006 this->min = 0; 00007 this->hour = 0; 00008 this->day = 0; 00009 00010 this->timer_10ms.attach(this, &RunTimer::timeAcc, .01); 00011 } 00012 00013 void RunTimer::timeAcc(void){ 00014 ms_total += 10.0; 00015 this->ms +=10; 00016 if(this->ms == 1000){ 00017 this->ms = 0; 00018 this->sec++; 00019 if(this->sec==60){ 00020 this->sec=0; 00021 this->min++; 00022 if(this->min == 60){ 00023 this->min=0; 00024 this->hour++; 00025 if(this->hour==24){ 00026 this->hour=0; 00027 day++; 00028 } 00029 } 00030 } 00031 } 00032 }//timeAcc 00033 00034 void RunTimer::Reset(void){ 00035 this->ms=0; 00036 this->sec =0; 00037 this->min = 0; 00038 this->hour = 0; 00039 this->day = 0; 00040 }
Generated on Sat Jul 16 2022 08:37:27 by
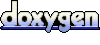