
Initial Commit
BNO055 Class Reference
Class for operating Bosch BNO055 sensor over I2C. More...
#include <BNO055.h>
Public Member Functions | |
BNO055 (PinName SDA, PinName SCL) | |
Create BNO055 instance. | |
void | reset () |
Perform a power-on reset of the BNO055. | |
bool | check () |
Check that the BNO055 is connected and download the software details and serial number of chip and store in ID structure. | |
void | SetExternalCrystal (bool yn) |
Turn the external timing crystal on/off. | |
void | setmode (char mode) |
Set the operation mode of the sensor. | |
void | setpowermode (char mode) |
Set the power mode of the sensor. | |
void | set_accel_units (char units) |
Set the output units from the accelerometer, either MPERSPERS or MILLIG. | |
void | set_anglerate_units (char units) |
Set the output units from the gyroscope, either DEG_PER_SEC or RAD_PER_SEC. | |
void | set_angle_units (char units) |
Set the output units from the IMU, either DEGREES or RADIANS. | |
void | set_temp_units (char units) |
Set the output units from the temperature sensor, either CENTIGRADE or FAHRENHEIT. | |
void | set_orientation (int position) |
Set the data output format to either WINDOWS or ANDROID. | |
void | set_mapping (char orient) |
Set the mapping of the exes/directions as per page 25 of datasheet range 0-7, any value outside this will set the orientation to P1 (default at power up) | |
void | get_accel (void) |
Get the current values from the accelerometer. | |
void | get_gyro (void) |
Get the current values from the gyroscope. | |
void | get_mag (void) |
Get the current values from the magnetometer. | |
void | get_lia (void) |
Get the corrected linear acceleration. | |
void | get_grv (void) |
Get the current gravity vector. | |
void | get_quat (void) |
Get the output quaternion. | |
void | get_angles (void) |
Get the current Euler angles. | |
void | get_temp (void) |
Get the current temperature. | |
void | get_calib (void) |
Read the calibration status register and store the result in the calib variable. | |
void | read_calibration_data (void) |
Read the offset and radius values into the calibration array. | |
void | write_calibration_data (void) |
Write the contents of the calibration array into the registers. | |
Data Fields | |
values | accel |
Structures containing 3-axis data for acceleration, rate of turn and magnetic field. | |
angles | euler |
Stucture containing the Euler angles as yaw, pitch, roll as scaled floating point and rawyaw, rawroll & rollpitch as the int16_t values loaded from the registers. | |
quaternion | quat |
Quaternion values as w,x,y,z (scaled floating point) and raww etc... | |
char | calib |
Current contents of calibration status register. | |
char | calibration [22] |
Contents of the 22 registers containing offset and radius values used as calibration by the sensor. | |
chip | ID |
Structure containing sensor numbers, software version and chip UID. | |
int | temperature |
Current temperature. |
Detailed Description
Class for operating Bosch BNO055 sensor over I2C.
Definition at line 177 of file BNO055.h.
Constructor & Destructor Documentation
BNO055 | ( | PinName | SDA, |
PinName | SCL | ||
) |
Create BNO055 instance.
Definition at line 4 of file BNO055.cpp.
Member Function Documentation
bool check | ( | ) |
Check that the BNO055 is connected and download the software details and serial number of chip and store in ID structure.
Definition at line 23 of file BNO055.cpp.
void get_accel | ( | void | ) |
Get the current values from the accelerometer.
Definition at line 157 of file BNO055.cpp.
void get_angles | ( | void | ) |
Get the current Euler angles.
Definition at line 231 of file BNO055.cpp.
void get_calib | ( | void | ) |
Read the calibration status register and store the result in the calib variable.
Definition at line 249 of file BNO055.cpp.
void get_grv | ( | void | ) |
Get the current gravity vector.
Definition at line 205 of file BNO055.cpp.
void get_gyro | ( | void | ) |
Get the current values from the gyroscope.
Definition at line 169 of file BNO055.cpp.
void get_lia | ( | void | ) |
Get the corrected linear acceleration.
Definition at line 193 of file BNO055.cpp.
void get_mag | ( | void | ) |
Get the current values from the magnetometer.
Definition at line 181 of file BNO055.cpp.
void get_quat | ( | void | ) |
Get the output quaternion.
Definition at line 217 of file BNO055.cpp.
void get_temp | ( | void | ) |
Get the current temperature.
Definition at line 244 of file BNO055.cpp.
void read_calibration_data | ( | void | ) |
Read the offset and radius values into the calibration array.
Definition at line 254 of file BNO055.cpp.
void reset | ( | ) |
Perform a power-on reset of the BNO055.
Definition at line 14 of file BNO055.cpp.
void set_accel_units | ( | char | units ) |
Set the output units from the accelerometer, either MPERSPERS or MILLIG.
Definition at line 54 of file BNO055.cpp.
void set_angle_units | ( | char | units ) |
Set the output units from the IMU, either DEGREES or RADIANS.
Definition at line 80 of file BNO055.cpp.
void set_anglerate_units | ( | char | units ) |
Set the output units from the gyroscope, either DEG_PER_SEC or RAD_PER_SEC.
Definition at line 67 of file BNO055.cpp.
void set_mapping | ( | char | orient ) |
Set the mapping of the exes/directions as per page 25 of datasheet range 0-7, any value outside this will set the orientation to P1 (default at power up)
Definition at line 276 of file BNO055.cpp.
void set_orientation | ( | int | position ) |
Set the data output format to either WINDOWS or ANDROID.
Definition at line 106 of file BNO055.cpp.
void set_temp_units | ( | char | units ) |
Set the output units from the temperature sensor, either CENTIGRADE or FAHRENHEIT.
Definition at line 93 of file BNO055.cpp.
void SetExternalCrystal | ( | bool | yn ) |
Turn the external timing crystal on/off.
Definition at line 46 of file BNO055.cpp.
void setmode | ( | char | mode ) |
Set the operation mode of the sensor.
Definition at line 147 of file BNO055.cpp.
void setpowermode | ( | char | mode ) |
Set the power mode of the sensor.
Definition at line 152 of file BNO055.cpp.
void write_calibration_data | ( | void | ) |
Write the contents of the calibration array into the registers.
Definition at line 265 of file BNO055.cpp.
Field Documentation
values accel |
char calib |
char calibration[22] |
angles euler |
chip ID |
quaternion quat |
int temperature |
Generated on Wed Jul 20 2022 08:19:56 by
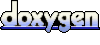