
Firmware enhancements for HSP_RPC_GUI 3.0.1
Fork of HSP_RPC_GUI by
main.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "MAX14720.h" 00035 #include "MAX30101.h" 00036 #include "MAX30205.h" 00037 #include "LIS2DH.h" 00038 #include "USBSerial.h" 00039 #include "RpcServer.h" 00040 #include "StringInOut.h" 00041 #include "Peripherals.h" 00042 #include "BMP280.h" 00043 #include "MAX30001.h" 00044 #include "DataLoggingService.h" 00045 #include "MAX30101_helper.h" 00046 #include "S25FS512.h" 00047 #include "QuadSpiInterface.h" 00048 #include "PushButton.h" 00049 #include "BLE.h" 00050 #include "HspBLE.h" 00051 #include "USBSerial.h" 00052 #include "Streaming.h" 00053 00054 /// define the HVOUT Boost Voltage default for the MAX14720 PMIC 00055 #define HVOUT_VOLTAGE 4500 // set to 4500 mV 00056 00057 /// define all I2C addresses 00058 #define MAX30205_I2C_SLAVE_ADDR_TOP (0x92) 00059 #define MAX30205_I2C_SLAVE_ADDR_BOTTOM (0x90) 00060 #define MAX14720_I2C_SLAVE_ADDR (0x54) 00061 #define BMP280_I2C_SLAVE_ADDR (0xEC) 00062 #define MAX30101_I2C_SLAVE_ADDR (0xAE) 00063 #define LIS2DH_I2C_SLAVE_ADDR (0x32) 00064 00065 /// 00066 /// wire Interfaces 00067 /// 00068 /// Define with Maxim VID and a Maxim assigned PID, set to version 0x0001 and non-blocking 00069 USBSerial usbSerial(0x0b6a, 0x0100, 0x0001, false); 00070 /// I2C Master 1 00071 I2C i2c1(I2C1_SDA, I2C1_SCL); // used by MAX30205 (1), MAX30205 (2), BMP280 00072 /// I2C Master 2 00073 I2C i2c2(I2C2_SDA, I2C2_SCL); // used by MAX14720, MAX30101, LIS2DH 00074 /// SPI Master 0 with SPI0_SS for use with MAX30001 00075 SPI spi(SPI0_MOSI, SPI0_MISO, SPI0_SCK, SPI0_SS); // used by MAX30001 00076 /// SPI Master 1 00077 QuadSpiInterface quadSpiInterface(SPI1_MOSI, SPI1_MISO, SPI1_SCK, 00078 SPI1_SS); // used by S25FS512 00079 00080 /// 00081 /// Devices 00082 /// 00083 /// Pressure Sensor 00084 BMP280 bmp280(&i2c1, BMP280_I2C_SLAVE_ADDR); 00085 /// Top Local Temperature Sensor 00086 MAX30205 MAX30205_top(&i2c1, MAX30205_I2C_SLAVE_ADDR_TOP); 00087 /// Bottom Local Temperature Sensor 00088 MAX30205 MAX30205_bottom(&i2c1, MAX30205_I2C_SLAVE_ADDR_BOTTOM); 00089 /// Accelerometer 00090 LIS2DH lis2dh(&i2c2, LIS2DH_I2C_SLAVE_ADDR); 00091 InterruptIn lis2dh_Interrupt(P4_7); 00092 /// PMIC 00093 MAX14720 max14720(&i2c2, MAX14720_I2C_SLAVE_ADDR); 00094 /// Optical Oximeter 00095 MAX30101 max30101(&i2c2, MAX30101_I2C_SLAVE_ADDR); 00096 InterruptIn max30101_Interrupt(P4_0); 00097 /// External Flash 00098 S25FS512 s25fs512(&quadSpiInterface); 00099 /// ECG device 00100 MAX30001 max30001(&spi); 00101 InterruptIn max30001_InterruptB(P3_6); 00102 InterruptIn max30001_Interrupt2B(P4_5); 00103 /// PWM used as fclk for the MAX30001 00104 PwmOut pwmout(P1_7); 00105 /// HSP platform LED 00106 HspLed hspLed(LED_RED); 00107 /// Packet TimeStamp Timer, set for 1uS 00108 Timer timestampTimer; 00109 /// HSP Platform push button 00110 PushButton pushButton(SW1); 00111 00112 /// BLE instance 00113 static BLE ble; 00114 00115 /// HSP BluetoothLE specific functions 00116 HspBLE hspBLE(&ble); 00117 USBSerial *usbSerialPtr; 00118 00119 int main() { 00120 // hold results for returning funtcoins 00121 int result; 00122 // local input state of the RPC 00123 int inputState; 00124 // RPC request buffer 00125 char request[128]; 00126 // RPC reply buffer 00127 char reply[128]; 00128 00129 // display start banner 00130 printf("Maxim Integrated mbed hSensor 3.0.0 10/14/16\n"); 00131 fflush(stdout); 00132 00133 // initialize HVOUT on the MAX14720 PMIC 00134 printf("Init MAX14720...\n"); 00135 fflush(stdout); 00136 result = max14720.init(); 00137 if (result == MAX14720_ERROR) 00138 printf("Error initializing MAX14720"); 00139 max14720.boostEn = MAX14720::BOOST_ENABLED; 00140 max14720.boostSetVoltage(HVOUT_VOLTAGE); 00141 00142 // turn on red led 00143 printf("Init HSPLED...\n"); 00144 fflush(stdout); 00145 hspLed.on(); 00146 00147 // set NVIC priorities for GPIO to prevent priority inversion 00148 printf("Init NVIC Priorities...\n"); 00149 fflush(stdout); 00150 NVIC_SetPriority(GPIO_P0_IRQn, 5); 00151 NVIC_SetPriority(GPIO_P1_IRQn, 5); 00152 NVIC_SetPriority(GPIO_P2_IRQn, 5); 00153 NVIC_SetPriority(GPIO_P3_IRQn, 5); 00154 NVIC_SetPriority(GPIO_P4_IRQn, 5); 00155 NVIC_SetPriority(GPIO_P5_IRQn, 5); 00156 NVIC_SetPriority(GPIO_P6_IRQn, 5); 00157 // used by the MAX30001 00158 NVIC_SetPriority(SPI1_IRQn, 0); 00159 00160 // Be able to statically reference these devices anywhere in the application 00161 Peripherals::setS25FS512(&s25fs512); 00162 Peripherals::setMAX30205_top(&MAX30205_top); 00163 Peripherals::setMAX30205_bottom(&MAX30205_bottom); 00164 Peripherals::setBMP280(&bmp280); 00165 Peripherals::setLIS2DH(&lis2dh); 00166 //Peripherals::setUSBSerial(&usbSerial); 00167 Peripherals::setTimestampTimer(×tampTimer); 00168 Peripherals::setHspLed(&hspLed); 00169 Peripherals::setMAX30101(&max30101); 00170 Peripherals::setI2c1(&i2c1); 00171 Peripherals::setI2c2(&i2c2); 00172 Peripherals::setPushButton(&pushButton); 00173 Peripherals::setBLE(&ble); 00174 Peripherals::setMAX14720(&max14720); 00175 Peripherals::setMAX30001(&max30001); 00176 Peripherals::setHspBLE(&hspBLE); 00177 usbSerialPtr = &usbSerial; 00178 00179 // init the S25FS256 external flash device 00180 printf("Init S25FS512...\n"); 00181 fflush(stdout); 00182 s25fs512.init(); 00183 00184 // init the BMP280 00185 printf("Init BMP280...\n"); 00186 fflush(stdout); 00187 bmp280.init(BMP280::OVERSAMPLING_X2_P, BMP280::OVERSAMPLING_X1_T, 00188 BMP280::FILT_OFF, BMP280::NORMAL_MODE, BMP280::T_62_5); 00189 00190 // Initialize BLE base layer 00191 printf("Init HSPBLE...\n"); 00192 fflush(stdout); 00193 hspBLE.init(); 00194 00195 // start blinking led1 00196 printf("Init HSPLED Blink...\n"); 00197 fflush(stdout); 00198 hspLed.blink(1000); 00199 00200 // MAX30101 initialize interrupt 00201 printf("Init MAX30101 callbacks, interrupt...\n"); 00202 fflush(stdout); 00203 max30101.onInterrupt(&MAX30101_OnInterrupt); 00204 max30101.onDataAvailable(&StreamPacketUint32); 00205 max30101_Interrupt.fall(&MAX30101::MidIntHandler); 00206 00207 // 00208 // MAX30001 00209 // 00210 printf("Init MAX30001 callbacks, interrupts...\n"); 00211 fflush(stdout); 00212 max30001_InterruptB.disable_irq(); 00213 max30001_Interrupt2B.disable_irq(); 00214 max30001_InterruptB.mode(PullUp); 00215 max30001_InterruptB.fall(&MAX30001::Mid_IntB_Handler); 00216 max30001_Interrupt2B.mode(PullUp); 00217 max30001_Interrupt2B.fall(&MAX30001::Mid_Int2B_Handler); 00218 max30001_InterruptB.enable_irq(); 00219 max30001_Interrupt2B.enable_irq(); 00220 max30001.AllowInterrupts(1); 00221 // Configuring the FCLK for the ECG, set to 32.768KHZ 00222 printf("Init MAX30001 PWM...\n"); 00223 fflush(stdout); 00224 //pwmout.period_us(31); 00225 //pwmout.write(0.5); // 0-1 is 0-100%, 0.5 = 50% duty cycle. 00226 max30001.FCLK_MaximOnly(); // mbed does not provide the resolution necessary, so for now we have a specific solution... 00227 max30001.sw_rst(); // Do a software reset of the MAX30001 00228 max30001.INT_assignment(MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, // en_enint_loc, en_eovf_loc, en_fstint_loc, 00229 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, // en_dcloffint_loc, en_bint_loc, en_bovf_loc, 00230 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_NO_INT, // en_bover_loc, en_bundr_loc, en_bcgmon_loc, 00231 MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, MAX30001::MAX30001_NO_INT, // en_pint_loc, en_povf_loc, en_pedge_loc, 00232 MAX30001::MAX30001_INT_2B, MAX30001::MAX30001_INT_B, MAX30001::MAX30001_NO_INT, // en_lonint_loc, en_rrint_loc, en_samp_loc, 00233 MAX30001::MAX30001_INT_ODNR, MAX30001::MAX30001_INT_ODNR); // intb_Type, int2b_Type) 00234 max30001.onDataAvailable(&StreamPacketUint32); 00235 00236 // initialize the LIS2DH accelerometer and interrupts 00237 printf("Init LIS2DH interrupt...\n"); 00238 fflush(stdout); 00239 lis2dh.init(); 00240 lis2dh_Interrupt.fall(&LIS2DHIntHandler); 00241 lis2dh_Interrupt.mode(PullUp); 00242 // initialize the RPC server 00243 printf("Init RPC Server...\n"); 00244 fflush(stdout); 00245 RPC_init(); 00246 // initialize the logging service 00247 printf("Init LoggingService...\n"); 00248 fflush(stdout); 00249 LoggingService_Init(); 00250 00251 // start main loop 00252 printf("Start main loop...\n"); 00253 fflush(stdout); 00254 while (1) { 00255 // get a RPC string if one is available 00256 inputState = getLine(request, sizeof(request)); 00257 // if a string has been captured, process string 00258 if (inputState == GETLINE_DONE) { 00259 // process the RPC string 00260 RPC_call(request, reply); 00261 // output the reply 00262 putStr(reply); 00263 } 00264 // process any logging or streaming requests 00265 LoggingService_ServiceRoutine(); 00266 // determine if we are doing a large USB transfer of flash datalog sensor data 00267 // skip updating the ble and sleeping, this conditional branch increases download 00268 // rates by about %450 (time to download 32M flash from 90 minutes to about 20 minutes) 00269 if (RPC_IsTransferingFlashPages() == false) { 00270 // allow for ble processing 00271 ble.waitForEvent(); 00272 } 00273 } 00274 }
Generated on Tue Jul 12 2022 22:52:23 by
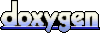