
Firmware enhancements for HSP_RPC_GUI 3.0.1
Fork of HSP_RPC_GUI by
HspLed.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef _LED_H_ 00034 #define _LED_H_ 00035 00036 #include "mbed.h" 00037 00038 /** 00039 * Driver for the HSP Led, supports different blink rates and patterns 00040 * 00041 */ 00042 00043 class HspLed { 00044 public: 00045 static const int LED_ON = 0; 00046 static const int LED_OFF = 1; 00047 00048 /// define all of the modes the LED can support 00049 typedef enum eMode { 00050 eLedOn, 00051 eLedOff, 00052 eLedPeriod, 00053 eLedPattern 00054 } eMode_t; 00055 /// define the values that turn the LED on or off at the pin 00056 #define HSP_LED_ON 0 00057 #define HSP_LED_OFF 1 00058 00059 /* 00060 * @brief Constructor where you specify the LED pin name 00061 */ 00062 HspLed(PinName ledPin); 00063 00064 /** 00065 * @brief Blink the HSP LED at a set time interval 00066 * @param mSeconds Number of seconds to set the timer interval 00067 */ 00068 void blink(uint32_t mSeconds); 00069 00070 /** 00071 * @brief Start rotating the LED through a 32-bit pattern at a mS rate specified 00072 * @param pattern 32-bit pattern to rotate through 00073 * @param mSeconds the amount of time to take per bit in the pattern 00074 */ 00075 void pattern(uint32_t pattern, uint32_t mSeconds); 00076 00077 /** 00078 * @brief Turn the LED on 00079 */ 00080 void on(void); 00081 00082 /** 00083 * @brief Turn the LED off 00084 */ 00085 void off(void); 00086 00087 /** 00088 * @brief Update the LED 00089 */ 00090 void service(void); 00091 00092 private: 00093 00094 /** 00095 * @brief Set the mode of the LED, the mode include blinking at a set rate or blinking 00096 * @brief according to a pattern 00097 * @param mode Mode to set the LED to 00098 */ 00099 void setMode(eMode_t state); 00100 00101 /** 00102 * @brief Toggle the state of the LED 00103 */ 00104 void toggle(void); 00105 00106 /** 00107 * @brief Start the LED blinking or rotating through a pattern 00108 */ 00109 void start(void); 00110 00111 /** 00112 * @brief Stop blinking or rotating through a pattern 00113 */ 00114 void stop(void); 00115 00116 /** 00117 * @brief Write the LED pin to a state 00118 * @param state A one or zero value to write to the LED pin 00119 */ 00120 void state(int state); 00121 00122 /** 00123 * @brief Single step through the pattern and output to the LED 00124 */ 00125 void patternToLed(void); 00126 00127 /** 00128 * @brief timer interval in mS 00129 */ 00130 float timerInterval; 00131 00132 /** 00133 * @brief last timer interval set to... used to prevent resetting the timer to the same value 00134 */ 00135 float timerIntervalLast; 00136 00137 /** 00138 * @brief local state of the pattern to rotate through 00139 */ 00140 uint32_t bitPattern; 00141 00142 /** 00143 * @brief current mode of the LED 00144 */ 00145 eMode_t mode; 00146 00147 /** 00148 * @brief the LED digital output 00149 */ 00150 DigitalOut redLed; 00151 00152 /** 00153 * @brief Timer service used to update the LED 00154 */ 00155 Ticker ticker; 00156 00157 /** 00158 * @brief Flag to indicate if the timer has been started 00159 */ 00160 bool isStarted; 00161 }; 00162 00163 #endif /* _LED_H_ */
Generated on Tue Jul 12 2022 22:52:23 by
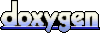