
Programa realizado para el trabajo: SISTEMA DE DETECCIÓN Y ALERTAS POR APROXIMACIÓN DE VEHÍCULOS EN ZONAS CIEGAS DE UNA MOTOCICLETA // JOSE ALBERTO RUIZ ORTEGA // UNIVERSIDAD NACIONAL DE COLOMBIA // SEDE MEDELLÍN // Realizado con colaboración del estudiante Jair Hernan Vargas y asesoría de los docentes: Gustavo Ramirez y Jesus Hernandez
Dependencies: DebouncedIn HCSR04 HCSR042 HCSR043 HCSR044 MMA8451Q TextLCD USBDevice mbed
main.cpp
00001 // 00002 // 00003 // SISTEMA DE DETECCIÓN Y ALERTAS POR APROXIMACIÓN DE VEHÍCULOS EN 00004 // ZONAS CIEGAS DE UNA MOTOCICLETA 00005 // 00006 // JOSE ALBERTO RUIZ ORTEGA 00007 // 1085262530 00008 // 00009 // INGENIERIA DE CONTROL 00010 // 00011 // UNIVERSIDAD NACIONAL DE COLOMBIA 00012 // 00013 // Programa realizado para la detección de vehiculos que se acercan a una motocicleta. 00014 // Para la realización de este trabajo se contó con la colaboracion del estudiante Jair Hernán Vargas 00015 // 00016 00017 00018 //:::::::::::::::::: Librerías 00019 #include "mbed.h" 00020 #include "HCSR04.h" 00021 #include "HCSR042.h" 00022 #include "HCSR043.h" 00023 #include "HCSR044.h" 00024 #include "TextLCD.h" 00025 #include "MMA8451Q.h" 00026 #include "DebouncedIn.h" 00027 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00028 00029 //:::::::::::::::::: Puertos 00030 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00031 PwmOut rojo(LED_RED); 00032 PwmOut verde(LED_GREEN); 00033 PwmOut azul(LED_BLUE); 00034 DigitalOut led2(PTE23); 00035 DigitalOut led3(PTE29); 00036 DigitalOut led4(PTC17); 00037 DigitalOut led5(PTA16); 00038 PwmOut ledD(PTC8); 00039 PwmOut ledI(PTC9); 00040 PwmOut sound(PTA12); 00041 DigitalOut ledbD(PTC7); 00042 DigitalOut ledbI(PTC0); 00043 DigitalOut ledgD(PTC5); 00044 DigitalOut ledgI(PTC6); 00045 HCSR04 sensor(PTD4, PTA13); 00046 HCSR042 sensor2(PTA5, PTD5); 00047 HCSR043 sensor3(PTA1, PTD0); 00048 HCSR044 sensor4(PTA4, PTD2); 00049 DebouncedIn button1(PTC12); 00050 00051 //:::::::::::::::::::::::::::::: Display 00052 00053 TextLCD lcd(PTB10, PTB11, PTE2, PTE3, PTE4, PTE5); 00054 00055 //:::::::::::::::::::::::::::::: Variables 00056 00057 float X,Y,Z; 00058 bool b; 00059 bool a; 00060 bool c; 00061 bool d; 00062 int e; 00063 float DD1; 00064 float DD2; 00065 00066 //::::::::::::::::::::::::::::::::: PROGRAMA 00067 int main() 00068 { 00069 00070 while(1){ 00071 //a=0; 00072 00073 //b=0; 00074 //:::::::::::::::::::::::::: Acelerómetro 00075 00076 00077 X=acc.getAccX(); 00078 Y=acc.getAccY(); 00079 Z=acc.getAccZ(); 00080 rojo=1.0 - abs(X); 00081 verde=1.0 - abs(Y); 00082 azul=1.0 - abs(Z); 00083 wait(0.1); 00084 00085 if (Y>=0.1) 00086 { 00087 led2=1; 00088 c=1; 00089 d=0; 00090 } 00091 else 00092 { 00093 led2=0; 00094 c=0; 00095 } 00096 if (Y<=-0.1) 00097 { 00098 d=1; 00099 c=0; 00100 led3=1; 00101 } 00102 else 00103 { 00104 led3=0; 00105 d=0; 00106 } 00107 00108 // :::::::::::::::::::::::::::::::::: Selección de Modos 00109 00110 lcd.locate(0,0); 00111 lcd.printf("e:%d ",e); 00112 wait(0.1); 00113 00114 if (button1.falling()) 00115 { 00116 e=e+1; 00117 00118 } 00119 if (e==3) 00120 { 00121 e=0; 00122 } 00123 00124 // MODO CARRETERA 00125 if (e==0) 00126 { 00127 DD1=120; 00128 DD2=400; 00129 lcd.locate(0,0); 00130 lcd.printf("Modo Carretera"); 00131 wait(0.1); 00132 } 00133 00134 // MODO CIUDAD 00135 00136 if (e==1) 00137 { 00138 DD1=70; 00139 DD2=300; 00140 lcd.locate(0,1); 00141 lcd.printf("Modo Ciudad"); 00142 wait(0.1); 00143 } 00144 00145 // MODO SIN ALARMAS 00146 00147 if (e==2) 00148 { 00149 sound=0; 00150 lcd.locate(0,1); 00151 lcd.printf("Modo Sin Alarma"); 00152 wait(0.1); 00153 } 00154 00155 //:::::::::::::::::::::::::::::::::::::::::::::SENSADO 00156 00157 // SENSOR 1 DERECHA ________________________________________________________________________________________ 00158 long d = sensor.distance(1); 00159 lcd.locate(0,0); 00160 lcd.printf("DER:%d ",sensor.distance(1)); 00161 00162 00163 // SENSOR 2 IZQUIERDA ________________________________________________________________________________________ 00164 long s = sensor2.distance(1); 00165 lcd.locate(8,0); 00166 lcd.printf("IZQ:%d ",sensor2.distance(1)); 00167 00168 // SENSOR 3 POSTERIOR ________________________________________________________________________________________ 00169 long p = sensor3.distance(1); 00170 lcd.locate(0,1); 00171 lcd.printf("FRN:%d ",sensor3.distance(1)); 00172 //wait(0.5); 00173 00174 // SENSOR 4 FRONTAL ________________________________________________________________________________________ 00175 long q = sensor4.distance(1); 00176 lcd.locate(8,1); 00177 lcd.printf("TRS:%d ",sensor4.distance(1)); 00178 //wait(0.5); 00179 00180 //:::::::::::::::::::::::: ALARMAS 00181 00182 // ::::::::::::::::::::::::::::::::ALERTA MUY CERCANA DERECHA 00183 00184 if (d>-1 && d<=DD1) 00185 { 00186 lcd.locate(0,0); 00187 lcd.printf("AMAXD "); 00188 ledbD=0; 00189 ledgD=0; 00190 00191 ledD.period(4.0); // 4 second period 00192 //ledD.pulsewidth(0.2); // 2 second pulse (on) 00193 ledD.write(0.20f); // 50% duty cycle 00194 //while(1); // led flashing 00195 a=1; 00196 } 00197 00198 // ::::::::::::::::::::::::::::::::ALERTA MUY CERCANA IZQUIERDA 00199 if(s>-1 && s<=DD1) 00200 { 00201 lcd.locate(8,0); 00202 lcd.printf("AMAXI "); 00203 ledbI=0; 00204 ledgI=0; 00205 00206 ledI.period(6.0); // 4 second period 00207 //ledI.pulsewidth(0.2); // 2 second pulse (on) 00208 ledI.write(0.20f); // 50% duty cycle 00209 //while(1); // led flashing 00210 a=1; 00211 } 00212 00213 // ::::::::::::::::::::::::::::::::ALERTA CERCANA DERECHA 00214 if(d>DD1 && d<=DD2) 00215 { 00216 ledD=0; 00217 ledbD=1; 00218 ledgD=0; 00219 a=1; 00220 lcd.locate(0,0); 00221 lcd.printf("AMIND "); 00222 00223 } 00224 00225 // ::::::::::::::::::::::::::::::::ALERTA CERCANA IZQUIERDA 00226 if(s>DD1 && s<=DD2) 00227 { 00228 ledI=0; 00229 ledbI=1; 00230 ledgI=0; 00231 a=1; 00232 lcd.locate(8,0); 00233 lcd.printf("AMINI "); 00234 } 00235 00236 00237 // ::::::::::::::::::::::::::::::::SIN ALERTAS DERECHA 00238 if(d>DD2) 00239 { 00240 ledD=0; 00241 ledbD=0; 00242 ledgD=1; 00243 a=0; 00244 lcd.locate(0,0); 00245 lcd.printf("NOALD "); 00246 } 00247 00248 //:::::::::::::::::::::::::::::::: SIN ALERTAS IZQ 00249 if(s>DD2) 00250 { 00251 ledI=0; 00252 ledbI=0; 00253 ledgI=1; 00254 a=0; 00255 lcd.locate(8,0); 00256 lcd.printf("NOALI "); 00257 } 00258 // :::::::::::::::::::::::::::::::: SIN ALERTAS DERECHA 00259 if(d==-1) 00260 { 00261 ledD=0; 00262 ledbD=0; 00263 ledgD=1; 00264 a=0; 00265 lcd.locate(0,0); 00266 lcd.printf("NOALD "); 00267 } 00268 00269 // :::::::::::::::::::::::::::::::: SIN ALERTAS IZQUIERDA 00270 if(s==-1) 00271 { 00272 ledI=0; 00273 ledbI=0; 00274 ledgI=1; 00275 a=0; 00276 lcd.locate(8,0); 00277 lcd.printf("NOALI "); 00278 } 00279 00280 00281 // ::::::::::::::::::::::::::::::::alarma POSTERIOR 00282 if (p<=100) 00283 { 00284 led4=1; 00285 } 00286 else 00287 { 00288 led4=0; 00289 } 00290 00291 // ::::::::::::::::::::::::::::::::alarma POSTERIOR 00292 if (q<=100) 00293 { 00294 led5=1; 00295 } 00296 else 00297 { 00298 led5=0; 00299 } 00300 // :::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::SONIDO 00301 00302 if (abs(Y)>=0.1 && a==1) 00303 { 00304 sound=1; 00305 wait(0.07); 00306 sound=0; 00307 } 00308 00309 00310 00311 } 00312 }
Generated on Tue Jul 12 2022 21:11:50 by
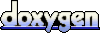