
Embed:
(wiki syntax)
Show/hide line numbers
usbhost_fat.h
00001 /* 00002 ************************************************************************************************************** 00003 * NXP USB Host Stack 00004 * 00005 * (c) Copyright 2008, NXP SemiConductors 00006 * (c) Copyright 2008, OnChip Technologies LLC 00007 * All Rights Reserved 00008 * 00009 * www.nxp.com 00010 * www.onchiptech.com 00011 * 00012 * File : usbhost_fat.h 00013 * Programmer(s) : Ravikanth.P 00014 * Version : 00015 * 00016 ************************************************************************************************************** 00017 */ 00018 00019 #ifndef USBHOST_FAT_H 00020 #define USBHOST_FAT_H 00021 00022 /* 00023 ************************************************************************************************************** 00024 * INCLUDE HEADER FILES 00025 ************************************************************************************************************** 00026 */ 00027 00028 #include "usbhost_inc.h" 00029 00030 /* 00031 ************************************************************************************************************** 00032 * FAT DEFINITIONS 00033 ************************************************************************************************************** 00034 */ 00035 00036 #define FAT_16 1 00037 00038 #define LAST_ENTRY 1 00039 #define FREE_ENTRY 2 00040 #define LFN_ENTRY 3 00041 #define SFN_ENTRY 4 00042 00043 #define RDONLY 1 00044 #define RDWR 2 00045 00046 /* 00047 ************************************************************************************************************** 00048 * TYPE DEFINITIONS 00049 ************************************************************************************************************** 00050 */ 00051 00052 typedef struct boot_sec { 00053 USB_INT32U BootSecOffset; /* Offset of the boot sector from sector 0 */ 00054 USB_INT16U BytsPerSec; /* Bytes per sector */ 00055 USB_INT08U SecPerClus; /* Sectors per cluster */ 00056 USB_INT32U BytsPerClus; /* Bytes per cluster */ 00057 USB_INT16U RsvdSecCnt; /* Reserved sector count */ 00058 USB_INT08U NumFATs; /* Number of FAT copies */ 00059 USB_INT16U RootEntCnt; /* Root entry count */ 00060 USB_INT16U TotSec16; /* Total sectors in the disk. !=0 if TotSec32 = 0 */ 00061 USB_INT32U TotSec32; /* Total sectors in the disk. !=0 if TotSec16 = 0 */ 00062 USB_INT16U FATSz16; /* Sectors occupied by single FAT table */ 00063 USB_INT08U FATType; /* File system type */ 00064 USB_INT32U RootDirSec; /* Sectors occupied by root directory */ 00065 USB_INT32U RootDirStartSec; /* Starting sector of the root directory */ 00066 USB_INT32U FirstDataSec; /* Starting sector of the first data cluster */ 00067 } BOOT_SEC; 00068 00069 typedef struct file_entry { 00070 USB_INT32U FileSize; /* Total size of the file */ 00071 USB_INT16U CurrClus; /* Current cluster of the cluster offset */ 00072 USB_INT32U CurrClusOffset; /* Current cluster offset */ 00073 USB_INT32U EntrySec; /* Sector where the file entry is located */ 00074 USB_INT32U EntrySecOffset; /* Offset in the entry sector from where the file is located */ 00075 USB_INT08U FileStatus; /* File's open status */ 00076 } FILE_ENTRY; 00077 00078 /* 00079 ************************************************************************************************************** 00080 * FUNCTION PROTOTYPES 00081 ************************************************************************************************************** 00082 */ 00083 00084 USB_INT32S FAT_Init (void); 00085 00086 USB_INT08U FAT_GetFATType (void); 00087 void PrintBootSec (void); 00088 00089 USB_INT32S FILE_Open ( USB_INT08U *file_name, 00090 USB_INT08U flags); 00091 00092 USB_INT32S FAT_FindEntry ( USB_INT08U *ent_name_given, 00093 FILE_ENTRY *entry); 00094 00095 void FAT_GetSFN (volatile USB_INT08U *entry, 00096 USB_INT08U *name); 00097 00098 void FAT_GetSfnName (volatile USB_INT08U *entry, 00099 USB_INT08U *name); 00100 00101 void FAT_GetSfnExt (volatile USB_INT08U *entry, 00102 USB_INT08U *ext_ptr); 00103 00104 USB_INT32S FAT_StrCaseCmp ( USB_INT08U *str1, 00105 USB_INT08U *str2); 00106 00107 USB_INT32U FAT_ChkEntType (volatile USB_INT08U *ent); 00108 00109 USB_INT32U FAT_ClusRead ( USB_INT16U curr_clus, 00110 USB_INT32U clus_offset, 00111 volatile USB_INT08U *buffer, 00112 USB_INT32U num_bytes); 00113 00114 USB_INT32U FILE_Read ( USB_INT32S fd, 00115 volatile USB_INT08U *buffer, 00116 USB_INT32U num_bytes); 00117 USB_INT16U FAT_GetNextClus ( USB_INT16U clus_no); 00118 00119 USB_INT32U FAT_ClusWrite ( USB_INT16U curr_clus, 00120 USB_INT32U clus_offset, 00121 volatile USB_INT08U *buffer, 00122 USB_INT32U num_bytes); 00123 USB_INT32U FILE_Write ( USB_INT32S fd, 00124 volatile USB_INT08U *buffer, 00125 USB_INT32U num_bytes); 00126 00127 void FAT_UpdateEntry ( FILE_ENTRY *entry); 00128 00129 void FAT_UpdateFAT ( USB_INT16U curr_clus, 00130 USB_INT16U value); 00131 00132 USB_INT16U FAT_GetFreeClus (void); 00133 00134 USB_INT32S FAT_GetFreeEntry( FILE_ENTRY *entry); 00135 00136 void FAT_PutSFN ( USB_INT08U *ent_name_given, 00137 FILE_ENTRY *entry); 00138 00139 USB_INT32S FAT_CreateEntry ( USB_INT08U *ent_name_given, 00140 FILE_ENTRY *entry); 00141 00142 void FILE_Close ( USB_INT32S fd); 00143 00144 USB_INT16U FAT_GetEndClus ( USB_INT16U clus_no); 00145 00146 #endif
Generated on Thu Jul 14 2022 00:28:57 by
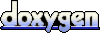