mbed library sources: Modified to operate FRDM-KL25Z at 48MHz from internal 32kHz oscillator (nothing else changed).
Fork of mbed-src by
Ticker.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_TICKER_H 00017 #define MBED_TICKER_H 00018 00019 #include "TimerEvent.h" 00020 #include "FunctionPointer.h" 00021 #include "CallChain.h" 00022 00023 namespace mbed { 00024 00025 /** A Ticker is used to call a function at a recurring interval 00026 * 00027 * You can use as many seperate Ticker objects as you require. 00028 * 00029 * Example: 00030 * @code 00031 * // Toggle the blinking led after 5 seconds 00032 * 00033 * #include "mbed.h" 00034 * 00035 * Ticker timer; 00036 * DigitalOut led1(LED1); 00037 * DigitalOut led2(LED2); 00038 * 00039 * int flip = 0; 00040 * 00041 * void attime() { 00042 * flip = !flip; 00043 * } 00044 * 00045 * int main() { 00046 * timer.attach(&attime, 5); 00047 * while(1) { 00048 * if(flip == 0) { 00049 * led1 = !led1; 00050 * } else { 00051 * led2 = !led2; 00052 * } 00053 * wait(0.2); 00054 * } 00055 * } 00056 * @endcode 00057 */ 00058 class Ticker : public TimerEvent { 00059 00060 public: 00061 00062 /** Attach a function to be called by the Ticker, specifiying the interval in seconds 00063 * 00064 * @param fptr pointer to the function to be called 00065 * @param t the time between calls in seconds 00066 * 00067 * @returns 00068 * The function object created for 'fptr' 00069 */ 00070 pFunctionPointer_t attach(void (*fptr)(void), float t) { 00071 return attach_us(fptr, t * 1000000.0f); 00072 } 00073 00074 /** Add a function to be called by the Ticker at the end of the call chain 00075 * 00076 * @param fptr the function to add 00077 * 00078 * @returns 00079 * The function object created for 'fptr' 00080 */ 00081 pFunctionPointer_t add_function(void (*fptr)(void)) { 00082 return add_function_helper(fptr); 00083 } 00084 00085 /** Add a function to be called by the Ticker at the beginning of the call chain 00086 * 00087 * @param fptr the function to add 00088 * 00089 * @returns 00090 * The function object created for 'fptr' 00091 */ 00092 pFunctionPointer_t add_function_front(void (*fptr)(void)) { 00093 return add_function_helper(fptr, true); 00094 } 00095 00096 /** Attach a member function to be called by the Ticker, specifiying the interval in seconds 00097 * 00098 * @param tptr pointer to the object to call the member function on 00099 * @param mptr pointer to the member function to be called 00100 * @param t the time between calls in seconds 00101 * 00102 * @returns 00103 * The function object created for 'tptr' and 'mptr' 00104 */ 00105 template<typename T> 00106 pFunctionPointer_t attach(T* tptr, void (T::*mptr)(void), float t) { 00107 return attach_us(tptr, mptr, t * 1000000.0f); 00108 } 00109 00110 /** Add a function to be called by the Ticker at the end of the call chain 00111 * 00112 * @param tptr pointer to the object to call the member function on 00113 * @param mptr pointer to the member function to be called 00114 * 00115 * @returns 00116 * The function object created for 'tptr' and 'mptr' 00117 */ 00118 template<typename T> 00119 pFunctionPointer_t add_function(T* tptr, void (T::*mptr)(void)) { 00120 return add_function_helper(tptr, mptr); 00121 } 00122 00123 /** Add a function to be called by the Ticker at the beginning of the call chain 00124 * 00125 * @param tptr pointer to the object to call the member function on 00126 * @param mptr pointer to the member function to be called 00127 * 00128 * @returns 00129 * The function object created for 'tptr' and 'mptr' 00130 */ 00131 template<typename T> 00132 pFunctionPointer_t add_function_front(T* tptr, void (T::*mptr)(void)) { 00133 return add_function_helper(tptr, mptr, true); 00134 } 00135 00136 /** Attach a function to be called by the Ticker, specifiying the interval in micro-seconds 00137 * 00138 * @param fptr pointer to the function to be called 00139 * @param t the time between calls in micro-seconds 00140 * 00141 * @returns 00142 * The function object created for 'fptr' 00143 */ 00144 pFunctionPointer_t attach_us(void (*fptr)(void), unsigned int t) { 00145 _chain.clear(); 00146 pFunctionPointer_t pf = _chain.add(fptr); 00147 setup(t); 00148 return pf; 00149 } 00150 00151 /** Attach a member function to be called by the Ticker, specifiying the interval in micro-seconds 00152 * 00153 * @param tptr pointer to the object to call the member function on 00154 * @param mptr pointer to the member function to be called 00155 * @param t the time between calls in micro-seconds 00156 * 00157 * @returns 00158 * The function object created for 'tptr' and 'mptr' 00159 */ 00160 template<typename T> 00161 pFunctionPointer_t attach_us(T* tptr, void (T::*mptr)(void), unsigned int t) { 00162 _chain.clear(); 00163 pFunctionPointer_t pf = _chain.add(tptr, mptr); 00164 setup(t); 00165 return pf; 00166 } 00167 00168 /** Detach the function 00169 */ 00170 void detach(); 00171 00172 /** Remove a function from the Ticker's call chain 00173 * 00174 * @param pf the function object to remove 00175 * 00176 * @returns 00177 * true if the function was found and removed, false otherwise 00178 */ 00179 bool remove_function(pFunctionPointer_t pf) { 00180 bool res = _chain.remove(pf); 00181 if (res && _chain.size() == 0) 00182 detach(); 00183 return res; 00184 } 00185 00186 protected: 00187 void setup(unsigned int t); 00188 pFunctionPointer_t add_function_helper(void (*fptr)(void), bool front=false); 00189 virtual void handler(); 00190 00191 template<typename T> 00192 pFunctionPointer_t add_function_helper(T* tptr, void (T::*mptr)(void), bool front=false) { 00193 if (_chain.size() == 0) 00194 return NULL; 00195 return front ? _chain.add_front(tptr, mptr) : _chain.add(tptr, mptr); 00196 } 00197 00198 unsigned int _delay; 00199 CallChain _chain; 00200 }; 00201 00202 } // namespace mbed 00203 00204 #endif
Generated on Wed Jul 13 2022 19:29:54 by
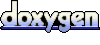