RTOS enabled i2c-driver based on the official i2c-C-api.
Fork of mbed-RtosI2cDriver by
I2CDriverTest03.h
00001 // exchange messages betwen the LPC1768's two i2c ports using low level read/write/start/stop commands 00002 // changing master and slave mode on the fly 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "I2CMasterRtos.h" 00007 #include "I2CSlaveRtos.h" 00008 00009 const int freq = 400000; 00010 const int adr = 42<<1; 00011 const int len=34; 00012 const char mstMsg[len]="We are mbed, resistance is futile"; 00013 const char slvMsg[len]="Fine with me, let's get addicted "; 00014 00015 static void slvRxMsg(I2CSlaveRtos& slv) 00016 { 00017 char rxMsg[len]; 00018 memset(rxMsg,0,len); 00019 if ( slv.receive() == I2CSlave::WriteAddressed ) { 00020 int cnt=0; 00021 while(cnt<len) rxMsg[cnt++]=slv.read(); 00022 slv.stop(); // stop sretching low level of scl 00023 printf("thread %x received message (sz=%d) as i2c slave: '%s'\n",Thread::gettid(),cnt,rxMsg); 00024 } else 00025 printf("Ouch slv rx failure\n"); 00026 } 00027 00028 static void slvTxMsg(I2CSlaveRtos& slv) 00029 { 00030 if ( slv.receive()==I2CSlave::ReadAddressed) { 00031 int cnt=0; 00032 while(cnt<len && slv.write(slvMsg[cnt++])); 00033 slv.stop(); // stop sretching low level of scl 00034 } else 00035 printf("Ouch slv tx failure\n"); 00036 } 00037 00038 static void mstTxMsg(I2CMasterRtos& mst) 00039 { 00040 mst.start(); 00041 if(!mst.write(adr & 0xfe))printf("adr+W not acked\n"); 00042 int cnt=0; 00043 while(cnt<len && mst.write(mstMsg[cnt++])); 00044 // give the slave a chance to stop stretching scl to low, otherwise we will busy wait for the stop forever 00045 while(!mst.stop())Thread::wait(1); 00046 } 00047 00048 static void mstRxMsg(I2CMasterRtos& mst) 00049 { 00050 char rxMsg[len]; 00051 memset(rxMsg,0,len); 00052 00053 mst.lock(); // no special reason, just a test 00054 mst.start(); 00055 if(!mst.write(adr | 0x01))printf("adr+R not acked\n"); 00056 int cnt=0; 00057 while(cnt<len-1) rxMsg[cnt++]=mst.read(1); 00058 mst.unlock(); 00059 rxMsg[cnt++]=mst.read(0); 00060 // give the slave a chance to stop stretching scl to low, otherwise we will busy wait for the stop forever 00061 while(!mst.stop())Thread::wait(1); 00062 printf("thread %x received message (sz=%d) as i2c master: '%s'\n",Thread::gettid(),cnt,rxMsg); 00063 } 00064 00065 static void channel1(void const *args) 00066 { 00067 I2CMasterRtos mst(p9,p10,freq); 00068 I2CSlaveRtos slv(p9,p10,freq,adr); 00069 while(1) { 00070 slvRxMsg(slv); 00071 slvTxMsg(slv); 00072 Thread::wait(100); 00073 mstTxMsg(mst); 00074 Thread::wait(100); 00075 mstRxMsg(mst); 00076 } 00077 } 00078 00079 void channel2(void const *args) 00080 { 00081 I2CMasterRtos mst(p28,p27,freq); 00082 I2CSlaveRtos slv(p28,p27,freq,adr); 00083 while(1) { 00084 Thread::wait(100); 00085 mstTxMsg(mst); 00086 Thread::wait(100); 00087 mstRxMsg(mst); 00088 slvRxMsg(slv); 00089 slvTxMsg(slv); 00090 } 00091 } 00092 00093 int doit() 00094 { 00095 Thread selftalk01(channel1,0); 00096 Thread selftalk02(channel2,0); 00097 Thread::wait(5000); 00098 return 0; 00099 } 00100
Generated on Wed Jul 13 2022 17:20:05 by
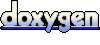