Interface for the encoder of the red fischertechnik motors
Embed:
(wiki syntax)
Show/hide line numbers
FtEncoder.h
00001 #ifndef FTENCODER_H 00002 #define FTENCODER_H 00003 00004 #include "InterruptIn.h" 00005 #include "us_ticker_api.h" 00006 #include "FunctionPointer.h" 00007 #include "TimeoutTweaked.h" 00008 00009 using namespace mbed; 00010 00011 /// Simple Interface for the simple encoder of the red fischertechnik motors. 00012 /// The interface counts both, rising and falling edges, resulting in 150 counts per revolution. 00013 /// The interface also provides a speed measurement updated with each edge. 00014 /// @note Connect the green wire to GND, the red one to +3.3V and the 00015 /// black signal line to any of mbed numbered pins. Additionally connect the signal line 00016 /// via a pullup resitor to +3.3V. A 10K resistor works fine. 00017 /// 00018 class FtEncoder 00019 { 00020 static const float c_speedFactor = 1e6/75.0; /// 1/(µs * 150/2) edges per revolution) 00021 static const int c_nTmStmps = 1<<2; /// size of ringbuffer: Has to be 2^x 00022 00023 InterruptIn m_encIRQ; 00024 TimeoutTweaked m_tmOut; 00025 00026 volatile unsigned int m_cnt; /// edge counter 00027 unsigned int m_standStillTimeout; 00028 volatile unsigned int m_cursor; /// ringbuffer cursor 00029 volatile uint32_t m_timeStamps[c_nTmStmps]; /// ringbuffer of edge timestamps 00030 00031 FunctionPointer m_callBack; 00032 00033 /// ISR called on rising and falling encoder edges 00034 void encoderISR(); 00035 /// ISR called on edge timeout i.e. standstill 00036 void timeoutISR(); 00037 00038 public: 00039 00040 /// create a simple interface to the encoder connected to the given pin 00041 /// @param name of the pin the black encoder signal wire is connected to 00042 /// @param standStillTimeout After this time [µs] without any encoder edge getSpeed() returns zero 00043 FtEncoder(PinName pwm, unsigned int standStillTimeout=50000); 00044 00045 /// get number of falling and rising encoder edges 00046 inline unsigned int getCounter() const { 00047 return m_cnt; 00048 }; 00049 00050 /// reset the encoder edge counter to the given value (default is 0) 00051 inline void resetCounter(unsigned int cnt=0) { 00052 m_cnt=cnt; 00053 }; 00054 00055 /// get period [µs] between the two latest edges of same direction (rising or falling) 00056 /// @note Returns standStillTimeout if motor stands still or no edges have been detected yet 00057 unsigned int getLastPeriod() const; 00058 00059 /// get time stamp [µs] of last update i.e. encoder edge or timeout 00060 unsigned int getTimeStampOfLastUpdate() const { 00061 return m_timeStamps[m_cursor]; 00062 }; 00063 00064 /// returns current time stamp [µs] 00065 /// @note simply calls us_ticker_read() from mbed's C-API (capi/us_ticker_api.h) 00066 inline unsigned int getCurrentTimeStamp() const { 00067 return us_ticker_read(); 00068 } 00069 00070 /// get speed in revolutions per second (Hz) 00071 float getSpeed() const; 00072 00073 /// hook in a call back to the encoder ISR e.g. for sending a RTOS signal 00074 void setCallBack(FunctionPointer callBack) { 00075 m_callBack=callBack; 00076 }; 00077 }; 00078 00079 #endif
Generated on Thu Jul 14 2022 22:48:54 by
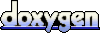