
EdgeCounter on mbed Counts falling edges on p5. Used as test setup for interfacing a hall sensor to our water/gas sensor. Whenever the hall sensor triggers, edgecount is incremented and the current value is transmitted to the serial port. Any character sent to the serial port returns the current count value
main.cpp
00001 // EdgeCounter on mbed 00002 // 00003 // Counts falling edges on p5. 00004 // Used as test setup for interfacing a hall sensor to our water/gas sensor. 00005 // 00006 // Whenever the hall sensor triggers, edgecount is incremented and the 00007 // current value is transmitted to the serial port. 00008 // 00009 // Any character sent to the serial port returns the current count value 00010 // 00011 // Written by Lieven Hollevoet 00012 00013 #include "mbed.h" 00014 00015 // Create objects 00016 Serial pc(USBTX, USBRX); 00017 DigitalOut status(LED4); 00018 DigitalOut count(LED2); 00019 InterruptIn sensor(p5); 00020 00021 int edgecount; 00022 00023 void ISR_serial(void) { 00024 00025 char tmp = pc.getc(); 00026 status = 1; 00027 00028 pc.printf("Current edge count is %i\r\n", edgecount); 00029 status = 0; 00030 } 00031 00032 void falling_edge(void){ 00033 edgecount++; 00034 count = 1; 00035 pc.printf("Falling edge, count is %i!\r\n", edgecount); 00036 count = 0; 00037 } 00038 00039 int main() { 00040 00041 count = 0; 00042 edgecount = 0; 00043 00044 pc.printf("Edgecounter started"); 00045 00046 // Attach interrupt handlers 00047 pc.attach(&ISR_serial); 00048 sensor.fall(&falling_edge); 00049 00050 } 00051
Generated on Wed Jul 13 2022 21:42:46 by
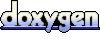