touch screen handler for the microchip AR1020
AR1020 Class Reference
class handling all the connections to a AR1020 touch screen controller SPI is handled by bit-banging, since the SPI-mode is non-standard. More...
#include <ar1020.h>
Inherits TouchPanel.
Public Member Functions | |
AR1020 (PinName mosi, PinName miso, PinName clk, PinName enable, PinName siq, PinName power) | |
mosi the MOSI pin name (SDI) miso the MISO pin name (SDO) clk the CLK pin (SCL) enable the enable pin name (/CS) siq the SIQ pin name (SIQ) power the power pin name (connected to Vdd - this library does power handling for the AR1020) | |
virtual void | init () |
initialize the controller | |
virtual int | x () |
read X coordinate (from last update) | |
virtual int | y () |
read Y coordinate (from last update) | |
virtual int | pen () |
virtual void | read () |
read coordinates on request | |
void | calibrate () |
execute touch screen calibration | |
template<class T > | |
void | attachPenDown (T *item, uint32_t(T::*method)(uint32_t)) |
attach listener called on 'pen-down' item the callback object method the callback method | |
void | attachPenDown (uint32_t(*function)(uint32_t)) |
attach listener called on 'pen-down' function the callback function | |
template<class T > | |
void | attachPenMove (T *item, uint32_t(T::*method)(uint32_t)) |
attach listener called on 'pen-move' item the callback object method the callback method | |
void | attachPenMove (uint32_t(*function)(uint32_t)) |
attach listener called on 'pen-move' function the callback function | |
template<class T > | |
void | attachPenUp (T *item, uint32_t(T::*method)(uint32_t)) |
attach listener called on 'pen-up' item the callback object method the callback method | |
void | attachPenUp (uint32_t(*function)(uint32_t)) |
attach listener called on 'pen-up' function the callback function |
Detailed Description
class handling all the connections to a AR1020 touch screen controller SPI is handled by bit-banging, since the SPI-mode is non-standard.
Therefore all pins can be used for the connection. Since the AR1020 seems to be sensitive to the changing pin signals during reset and startup, this library controls the AR1020 power (so it's Vcc must be connected to an mbed pin)
Definition at line 37 of file ar1020.h.
Constructor & Destructor Documentation
AR1020 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | clk, | ||
PinName | enable, | ||
PinName | siq, | ||
PinName | power | ||
) |
mosi the MOSI pin name (SDI) miso the MISO pin name (SDO) clk the CLK pin (SCL) enable the enable pin name (/CS) siq the SIQ pin name (SIQ) power the power pin name (connected to Vdd - this library does power handling for the AR1020)
Definition at line 27 of file ar1020.cpp.
Member Function Documentation
void attachPenDown | ( | T * | item, |
uint32_t(T::*)(uint32_t) | method | ||
) | [inherited] |
attach listener called on 'pen-down' item the callback object method the callback method
Definition at line 66 of file touchpanel.h.
void attachPenDown | ( | uint32_t(*)(uint32_t) | function ) | [inherited] |
attach listener called on 'pen-down' function the callback function
Definition at line 71 of file touchpanel.h.
void attachPenMove | ( | T * | item, |
uint32_t(T::*)(uint32_t) | method | ||
) | [inherited] |
attach listener called on 'pen-move' item the callback object method the callback method
Definition at line 78 of file touchpanel.h.
void attachPenMove | ( | uint32_t(*)(uint32_t) | function ) | [inherited] |
attach listener called on 'pen-move' function the callback function
Definition at line 83 of file touchpanel.h.
void attachPenUp | ( | T * | item, |
uint32_t(T::*)(uint32_t) | method | ||
) | [inherited] |
attach listener called on 'pen-up' item the callback object method the callback method
Definition at line 90 of file touchpanel.h.
void attachPenUp | ( | uint32_t(*)(uint32_t) | function ) | [inherited] |
attach listener called on 'pen-up' function the callback function
Definition at line 95 of file touchpanel.h.
void calibrate | ( | ) |
execute touch screen calibration
Definition at line 241 of file ar1020.cpp.
void init | ( | ) | [virtual] |
initialize the controller
Definition at line 51 of file ar1020.cpp.
int pen | ( | ) | [virtual] |
- Returns:
- 0 if pen is up, 1 if pen is down
Definition at line 99 of file ar1020.cpp.
void read | ( | ) | [virtual] |
read coordinates on request
Definition at line 104 of file ar1020.cpp.
int x | ( | ) | [virtual] |
read X coordinate (from last update)
- Returns:
- last known X coordinate
Definition at line 91 of file ar1020.cpp.
int y | ( | ) | [virtual] |
read Y coordinate (from last update)
- Returns:
- last known Y coordinate
Definition at line 95 of file ar1020.cpp.
Generated on Tue Jul 12 2022 18:15:38 by
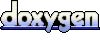