touch screen handler for the microchip AR1020
Embed:
(wiki syntax)
Show/hide line numbers
touchpanel.h
00001 /* 00002 * mbed AR1020 library 00003 * Copyright (c) 2010 Hendrik Lipka 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef __TOUCHPANEL_H_ 00025 #define __TOUCHPANEL_H_ 00026 00027 #include "FPointer.h" 00028 00029 class TouchPanel 00030 { 00031 public: 00032 /** 00033 initialize the controller 00034 */ 00035 virtual void init()=0; 00036 00037 /** 00038 read X coordinate (from last update) 00039 @returns last known X coordinate 00040 */ 00041 virtual int x()=0; 00042 /** 00043 read Y coordinate (from last update) 00044 @returns last known Y coordinate 00045 */ 00046 virtual int y()=0; 00047 00048 /** 00049 @return 0 if pen is up, >0 if pen is down (maybe different due to touch pressure, if supported) 00050 */ 00051 virtual int pen()=0; 00052 00053 /** 00054 read coordinates on request 00055 */ 00056 virtual void read()=0; 00057 00058 class T; 00059 00060 /** 00061 attach listener called on 'pen-down' 00062 @params item the callback object 00063 @params method the callback method 00064 */ 00065 template<class T> 00066 void attachPenDown(T* item, uint32_t (T::*method)(uint32_t)) { _callbackPD.attach(item, method); } 00067 /** 00068 attach listener called on 'pen-down' 00069 @params function the callback function 00070 */ 00071 void attachPenDown(uint32_t (*function)(uint32_t)) { _callbackPD.attach(function); } 00072 /** 00073 attach listener called on 'pen-move' 00074 @params item the callback object 00075 @params method the callback method 00076 */ 00077 template<class T> 00078 void attachPenMove(T* item, uint32_t (T::*method)(uint32_t)) { _callbackPM.attach(item, method); } 00079 /** 00080 attach listener called on 'pen-move' 00081 @params function the callback function 00082 */ 00083 void attachPenMove(uint32_t (*function)(uint32_t)) { _callbackPM.attach(function); } 00084 /** 00085 attach listener called on 'pen-up' 00086 @params item the callback object 00087 @params method the callback method 00088 */ 00089 template<class T> 00090 void attachPenUp(T* item, uint32_t (T::*method)(uint32_t)) { _callbackPU.attach(item, method); } 00091 /** 00092 attach listener called on 'pen-up' 00093 @params function the callback function 00094 */ 00095 void attachPenUp(uint32_t (*function)(uint32_t)) { _callbackPU.attach(function); } 00096 00097 protected: 00098 FPointer _callbackPD; 00099 FPointer _callbackPM; 00100 FPointer _callbackPU; 00101 }; 00102 00103 00104 #endif
Generated on Tue Jul 12 2022 18:15:37 by
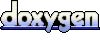