This library lets you connect an MRF24J40 tranceiver to your mbed. The MRF24J40 is intended for use as a zigbee tranceiver. However, it can also be used to simply send data from one tranceiver to another. The tranceiver is also available as a module on a small PCB with antenna etc. It requires no other components and can be connected to the mbed using 5 pins.
Dependents: mrf24jclient_vest1
MRF24J40 Class Reference
#include <MRF24J40.h>
Public Member Functions | |
MRF24J40 (PinName mosi, PinName miso, PinName sck, PinName cs, PinName reset) | |
Create a MRF24J40 object and initizalize it. | |
void | Reset (void) |
Reset the MRF24J40 and initialize it. | |
void | Send (uint8_t *data, uint8_t length) |
Send data. | |
uint8_t | Receive (uint8_t *data, uint8_t maxLength) |
Check if any data was received. |
Detailed Description
MRF24J40 class.
Provides a simple send/receive API for a microchip MFR24J40 IEEE 802.15.4 tranceiver. The tranceiver is available on a module that can easilly be soldered to some header pins to use it with an mbed on a breadboard. The module is called 'MRF24J40MA' and can be ordered for example by www.farnell.com.
Example:
#include "mbed.h" #include "MRF24J40.h" // RF tranceiver to link with handheld. MRF24J40 mrf(p11, p12, p13, p14, p21); // LEDs DigitalOut led1(LED1); DigitalOut led2(LED2); DigitalOut led3(LED3); DigitalOut led4(LED4); // Timer. Timer timer; // Serial port for showing RX data. Serial pc(USBTX, USBRX); // Send / receive buffers. // IMPORTANT: The MRF24J40 is intended as zigbee tranceiver; it tends // to reject data that doesn't have the right header. So the first // 8 bytes in txBuffer look like a valid header. The remaining 120 // bytes can be used for anything you like. uint8_t txBuffer[128]= {1, 8, 0, 0xA1, 0xB2, 0xC3, 0xD4, 0x00}; uint8_t rxBuffer[128]; uint8_t rxLen; int main (void) { uint8_t count = 0; pc.baud(115200); timer.start(); while(1) { // Check if any data was received. rxLen = mrf.Receive(rxBuffer, 128); if(rxLen) { // Toggle LED 1 upon each reception of data. led1 = led1^1; // Send to serial. // IMPORTANT: The last two bytes of the received data // are the checksum used in the transmission. for(uint8_t i=0; i<rxLen; i++) { pc.printf("0x%02X ", rxBuffer[i]); } pc.printf("\r\n"); } // Each second, send some data. if(timer.read_ms() >= 1000) { timer.reset(); // Toggle LED 2. led2 = led2^1; // UART. pc.printf("TXD\r\n"); // Send counter value. count++; txBuffer[8] = count; mrf.Send(txBuffer, 9); } } }
Definition at line 108 of file MRF24J40.h.
Constructor & Destructor Documentation
MRF24J40 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sck, | ||
PinName | cs, | ||
PinName | reset | ||
) |
Create a MRF24J40 object and initizalize it.
- Parameters:
-
pin mosi Spi MOSI pin connected to MRF's SDI. pin miso Spi MISO pin connected to MRF's SDO. pin sck Spi SCK pin connected to MRF's SCK. pin cs Pin connected to MRF's CS. pin reset Pin connected to MRF's Reset.
Definition at line 69 of file MRF24J40.cpp.
Member Function Documentation
uint8_t Receive | ( | uint8_t * | data, |
uint8_t | maxLength | ||
) |
Check if any data was received.
Note that the MRF24J40 appends two bytes of CRC for each packet. So you will receive two bytes more than were send with the 'Send' function.
- Parameters:
-
data Pointer to buffer where received data can be placed. maxLength Maximum amount of data to be placed in the buffer. returns The number of bytes written into the buffer.
Definition at line 168 of file MRF24J40.cpp.
void Reset | ( | void | ) |
Reset the MRF24J40 and initialize it.
Definition at line 111 of file MRF24J40.cpp.
void Send | ( | uint8_t * | data, |
uint8_t | length | ||
) |
Send data.
Note that the MRF24J40 only handles data with a valid IEEE 802.15.4 header. See the example how to get around this.
- Parameters:
-
data Pointer to data to be send. length Length of the data to be send in bytes.
Definition at line 156 of file MRF24J40.cpp.
Generated on Wed Jul 13 2022 11:44:15 by
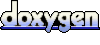