
this is a test about ble and so on
Dependencies: BLE_API LinkNode_TemperatureAdvertising mbed nRF51822
Fork of LinkNode_Temperatur by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 #include "Sensors/Sensors.h" 00004 00005 #define APP_SPECIFIC_ID_TEST 0xFEFE 00006 00007 #pragma pack(1) 00008 struct ApplicationData_t { 00009 uint16_t applicationSpecificId; /* An ID used to identify temperature value 00010 in the manufacture specific AD data field */ 00011 Sensors::tmpSensorValue_t tmpSensorValue; /* User defined application data */ 00012 }; 00013 #pragma pack() 00014 00015 BLE ble; 00016 Sensors tempSensor; 00017 DigitalOut led_r(P0_20); 00018 DigitalOut buzzer(P0_22); 00019 InterruptIn btn(P0_28); 00020 InterruptIn btn1(P0_29); 00021 static bool triggerTempValueUpdate = false; 00022 static bool start_flag = false; 00023 const static char DEVICE_NAME[8] = "Linkab"; /*The size of the DEVICE_NAME[] can't change */ 00024 00025 void periodicCallback(void) 00026 { 00027 /* Do blinky on LED1 while we're waiting for BLE events */ 00028 triggerTempValueUpdate = true; 00029 } 00030 00031 void accumulateApplicationData(ApplicationData_t &appData) 00032 { 00033 appData.applicationSpecificId = APP_SPECIFIC_ID_TEST; 00034 /* Read a new data value */ 00035 appData.tmpSensorValue = tempSensor.get(); 00036 } 00037 00038 void temperatureValueAdvertising(void) 00039 { 00040 ApplicationData_t appData; 00041 00042 accumulateApplicationData(appData); 00043 00044 /* Setup advertising payload */ 00045 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); /* Set flag */ 00046 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_THERMOMETER); /* Set appearance */ 00047 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00048 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, (uint8_t *)&appData, sizeof(ApplicationData_t)); /* Set data */ 00049 /* Setup advertising parameters */ 00050 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00051 ble.gap().setAdvertisingInterval(300); 00052 /* Start advertising */ 00053 ble.gap().startAdvertising(); 00054 } 00055 00056 void updateSensorValueInAdvPayload(void) 00057 { 00058 ApplicationData_t appData; 00059 00060 accumulateApplicationData(appData); 00061 00062 /* Stop advertising first */ 00063 ble.gap().stopAdvertising(); 00064 /* Only update data value field */ 00065 ble.gap().updateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, (uint8_t *)&appData, sizeof(ApplicationData_t)); 00066 /* Start advertising again */ 00067 ble.gap().startAdvertising(); 00068 } 00069 00070 void start_mode(void) 00071 { 00072 led_r=0; 00073 wait(0.2); 00074 led_r=1; 00075 wait(0.2); 00076 led_r=0; 00077 wait(0.2); 00078 led_r=1; 00079 ble.gap().startAdvertising(); 00080 } 00081 void stop_mode(void) 00082 { 00083 led_r=0; 00084 wait(0.5); 00085 led_r=1; 00086 wait(0.5); 00087 led_r=0; 00088 wait(0.5); 00089 led_r=1; 00090 ble.gap().stopAdvertising(); 00091 } 00092 00093 void change_startmode(void) 00094 { 00095 00096 start_flag=!start_flag; 00097 if(start_flag==true) 00098 { 00099 start_mode(); 00100 } 00101 else 00102 { 00103 stop_mode(); 00104 } 00105 printf("The state is %d\n",start_flag); 00106 } 00107 00108 00109 int main(void) 00110 { 00111 Ticker ticker; 00112 ticker.attach(periodicCallback,300); 00113 ble.init(); 00114 led_r=1; 00115 buzzer=0; 00116 temperatureValueAdvertising(); 00117 btn.fall(&change_startmode); 00118 btn1.fall(&updateSensorValueInAdvPayload); 00119 printf("This is a test!\n"); 00120 while(true) 00121 { 00122 if(start_flag==true) 00123 { 00124 if (triggerTempValueUpdate) 00125 { 00126 updateSensorValueInAdvPayload(); 00127 triggerTempValueUpdate = false; 00128 } 00129 ble.waitForEvent(); 00130 } 00131 else 00132 { 00133 ble.gap().stopAdvertising(); 00134 ble.waitForEvent(); 00135 } 00136 } 00137 }
Generated on Sat Jul 23 2022 00:24:36 by
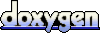