
Demo to get the die sensors value of 51822
Dependencies: BLE_API Sensors mbed nRF51822
Fork of LinkNode_DataAdvertising by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 #include "Sensors/Sensors.h" 00004 00005 #define APP_SPECIFIC_ID_TEST 0xFEFE 00006 00007 #pragma pack(1) 00008 struct ApplicationData_t 00009 { 00010 uint16_t applicationSpecificId; /* An ID used to identify temperature value 00011 in the manufacture specific AD data field */ 00012 Sensors::tmpSensorValue_t tmpSensorValue; /* User defined application data */ 00013 }; 00014 #pragma pack() 00015 00016 BLE ble; 00017 Sensors tempSensor; 00018 DigitalOut led(P0_19); 00019 static bool triggerTempValueUpdate = false; 00020 const static char DEVICE_NAME[8] = "Linkaa"; /*The size of the DEVICE_NAME[] can't change */ 00021 00022 void periodicCallback(void) 00023 { 00024 /* Do blinky on LED1 while we're waiting for BLE events */ 00025 led = !led; 00026 triggerTempValueUpdate = true; 00027 } 00028 00029 void accumulateApplicationData(ApplicationData_t &appData) 00030 { 00031 appData.applicationSpecificId = APP_SPECIFIC_ID_TEST; 00032 /* Read a new data value */ 00033 appData.tmpSensorValue = tempSensor.get(); 00034 } 00035 00036 void temperatureValueAdvertising(void) 00037 { 00038 ApplicationData_t appData; 00039 00040 accumulateApplicationData(appData); 00041 00042 /* Setup advertising payload */ 00043 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); /* Set flag */ 00044 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_THERMOMETER); /* Set appearance */ 00045 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00046 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, (uint8_t *)&appData, sizeof(ApplicationData_t)); /* Set data */ 00047 /* Setup advertising parameters */ 00048 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00049 ble.gap().setAdvertisingInterval(500); 00050 /* Start advertising */ 00051 ble.gap().startAdvertising(); 00052 } 00053 00054 void updateSensorValueInAdvPayload(void) 00055 { 00056 ApplicationData_t appData; 00057 00058 accumulateApplicationData(appData); 00059 00060 /* Stop advertising first */ 00061 ble.gap().stopAdvertising(); 00062 /* Only update data value field */ 00063 ble.gap().updateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, (uint8_t *)&appData, sizeof(ApplicationData_t)); 00064 /* Start advertising again */ 00065 ble.gap().startAdvertising(); 00066 } 00067 00068 int main(void) 00069 { 00070 Ticker ticker; 00071 ticker.attach(periodicCallback, 0.3); 00072 ble.init(); 00073 /* Start data advertising */ 00074 temperatureValueAdvertising(); 00075 00076 while (true) 00077 { 00078 00079 if (triggerTempValueUpdate) 00080 { 00081 /* Update data value */ 00082 updateSensorValueInAdvPayload(); 00083 triggerTempValueUpdate = false; 00084 } 00085 ble.waitForEvent(); 00086 } 00087 }
Generated on Sat Jul 23 2022 00:40:58 by
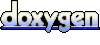