
Secure keypad
Dependencies: Keypad TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "Keypad.h" 00003 #include "TextLCD.h" 00004 Keypad kpad(PTC8, PTC1, PTB19, PTB18, PTC5, PTC7, PTC0, PTC9); 00005 Serial pc(USBTX, USBRX); 00006 TextLCD lcd(PTA1, PTB23, PTA2, PTC2, PTC3, PTC12, TextLCD::LCD16x2); 00007 /* 00008 col1, col2, col3, col4, 00009 row1, row2, row3, row4 00010 */ 00011 00012 const int number_of_chars = 4; 00013 const char pass_user1[number_of_chars] = {'1','2','3','A'}; //HA 00014 const char pass_user2[number_of_chars] = {'2','2','3','A'}; //FA 00015 const char pass_user3[number_of_chars] = {'5','2','3','A'}; //AA 00016 const char pass_user4[number_of_chars] = {'6','2','3','A'}; //MA 00017 const char pass_user5[number_of_chars] = {'7','2','3','A'}; //FF 00018 00019 char enterd_pass[number_of_chars] = {' ', ' ',' ',' '}; 00020 00021 DigitalOut led_red(LED_RED); 00022 DigitalOut led_green(LED_GREEN); 00023 DigitalOut active_buzzer(PTC4); //Buzzer on pin PTC4=D9 00024 00025 PwmOut beep(PTC4); // buzzer 00026 00027 //Serial pc(USBTX, USBRX); 00028 00029 int main() 00030 { 00031 char key; 00032 int i; 00033 int user_index1=0; 00034 int user_index2=0; 00035 int user_index3=0; 00036 int user_index4=0; 00037 int user_index5=0; 00038 00039 int released =1;; 00040 00041 00042 led_green = 0; 00043 led_red = 0; 00044 active_buzzer = 0; 00045 00046 beep.period(0.001); 00047 00048 lcd.cls(); 00049 wait(0.001); 00050 00051 00052 00053 while(true) { 00054 00055 lcd.locate(0,0); // col, row 00056 lcd.printf("Enter Password:"); 00057 00058 // Reading the password characters 00059 for(i=0; i<number_of_chars; i++) { 00060 key = kpad.ReadKey(); 00061 00062 if(key == '\0') 00063 released = 1; //set the flag when all keys are released 00064 if((key != '\0') && (released == 1)) { //if a key is pressed AND previous key was released 00065 enterd_pass[i]=key; 00066 lcd.locate(i,1); // col, row 00067 lcd.printf("*"); 00068 00069 released = 0; //clear the flag to indicate that key is still pressed 00070 } 00071 else 00072 i--; 00073 00074 00075 00076 } 00077 00078 wait(0.5); 00079 00080 lcd.locate(0,1); // col, row 00081 lcd.printf(" " ); 00082 00083 // comparing passwords 00084 00085 ///////// user 1 /////////////// 00086 for(i=0; i<number_of_chars; i++) { 00087 if( enterd_pass[i] == pass_user1[i] ) { 00088 user_index1 = 1; 00089 } else { 00090 user_index1 = 0; 00091 break; 00092 } 00093 } 00094 00095 00096 00097 ///////// user 2 /////////////// 00098 for(i=0; i<number_of_chars; i++) { 00099 if( enterd_pass[i] == pass_user2[i] ) { 00100 user_index2 = 2; 00101 } else { 00102 user_index2 = 0; 00103 break; 00104 } 00105 } 00106 ///////// user 3 /////////////// 00107 for(i=0; i<number_of_chars; i++) { 00108 if( enterd_pass[i] == pass_user3[i] ) { 00109 user_index3 = 3; 00110 } else { 00111 user_index3 = 0; 00112 break; 00113 } 00114 } 00115 ///////// user 4 /////////////// 00116 for(i=0; i<number_of_chars; i++) { 00117 if( enterd_pass[i] == pass_user4[i] ) { 00118 user_index4 = 4; 00119 } else { 00120 user_index4 = 0; 00121 break; 00122 } 00123 } 00124 ///////// user 5 /////////////// 00125 for(i=0; i<number_of_chars; i++) { 00126 if( enterd_pass[i] == pass_user5[i] ) { 00127 user_index5 = 5; 00128 } else { 00129 user_index5 = 0; 00130 break; 00131 } 00132 } 00133 ////////////////////////////////////////////////// 00134 if( (1 == user_index1)||(2 == user_index2)||(3 == user_index3)||(4 == user_index4)||(5 == user_index5) ) { 00135 pc.printf("Access granted "); 00136 led_red = 1; 00137 active_buzzer = 1; 00138 lcd.locate(0,1); // col, row 00139 lcd.printf("User: "); 00140 00141 if(1 == user_index1) { 00142 lcd.locate(6,1); // col, row 00143 lcd.printf("HA"); 00144 } 00145 if(2 == user_index2) { 00146 lcd.locate(6,1); // col, row 00147 lcd.printf("FA"); 00148 } 00149 if(3 == user_index3) { 00150 lcd.locate(6,1); // col, row 00151 lcd.printf("AA"); 00152 } 00153 if(4 == user_index4) { 00154 lcd.locate(6,1); // col, row 00155 lcd.printf("MA"); 00156 } 00157 if(5 == user_index5) { 00158 lcd.locate(6,1); // col, row 00159 lcd.printf("FF"); 00160 } 00161 00162 wait(1); 00163 active_buzzer = 1; 00164 beep = 80.3/100.0; 00165 led_red = 0; 00166 wait(1); 00167 lcd.cls(); 00168 00169 00170 } 00171 00172 else { 00173 lcd.locate(0,0); // col, row 00174 lcd.printf("Access denied "); 00175 led_green = 1; 00176 wait(1); 00177 led_green = 0; 00178 active_buzzer = 0; 00179 beep = 0.0/100.0; 00180 } 00181 00182 beep = 0.0/100.0; 00183 00184 } 00185 } 00186
Generated on Tue Jul 26 2022 11:59:50 by
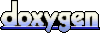