Peripheral_1
Dependencies: BLE_API mbed nRF51822
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ble/BLE.h" 00019 #include "ble/DiscoveredCharacteristic.h" 00020 #include "ble/DiscoveredService.h" 00021 BLE ble; 00022 DigitalOut led1(LED1); 00023 Serial pc(USBTX, USBRX); 00024 00025 00026 void periodicCallback(void) 00027 { 00028 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00029 } 00030 00031 void advertisementCallback(const Gap::AdvertisementCallbackParams_t *params) { 00032 if (params->peerAddr[0] == 0xcb) { /* !ALERT! Alter this filter to suit your device. */ 00033 return; 00034 } 00035 pc.printf("Adv peerAddr: [%02x %02x %02x %02x %02x %02x] rssi %d, ScanResp: %u, AdvType: %u\r\n", 00036 params->peerAddr[5], params->peerAddr[4], params->peerAddr[3], params->peerAddr[2], params->peerAddr[1], params->peerAddr[0], 00037 params->rssi, params->isScanResponse, params->type); 00038 00039 for (unsigned index = 0; index < params->advertisingDataLen; index++) { 00040 pc.printf("%02x ", params->advertisingData[index]); 00041 } 00042 pc.printf("\r\n"); 00043 /* DUMP_ADV_DATA */ 00044 /* 00045 ble.gap().connect(params->peerAddr, Gap::ADDR_TYPE_RANDOM_STATIC, NULL, NULL); 00046 */ 00047 } 00048 00049 void serviceDiscoveryCallback(const DiscoveredService *service) { 00050 if (service->getUUID().shortOrLong() == UUID::UUID_TYPE_SHORT) { 00051 pc.printf("S UUID-%x attrs[%u %u]\r\n", service->getUUID().getShortUUID(), service->getStartHandle(), service->getEndHandle()); 00052 } else { 00053 printf("S UUID-"); 00054 const uint8_t *longUUIDBytes = service->getUUID().getBaseUUID(); 00055 for (unsigned i = 0; i < UUID::LENGTH_OF_LONG_UUID; i++) { 00056 printf("%02x", longUUIDBytes[i]); 00057 } 00058 pc.printf(" attrs[%u %u]\r\n", service->getStartHandle(), service->getEndHandle()); 00059 } 00060 } 00061 00062 void characteristicDiscoveryCallback(const DiscoveredCharacteristic *characteristicP) { 00063 00064 } 00065 00066 void discoveryTerminationCallback(Gap::Handle_t connectionHandle) { 00067 pc.printf("terminated SD for handle %u\r\n", connectionHandle); 00068 } 00069 00070 void connectionCallback(const Gap::ConnectionCallbackParams_t *params) { 00071 pc.printf("Connected!!\r\n"); 00072 ble.stopAdvertising(); 00073 wait(1); 00074 if (params->role == Gap::CENTRAL) { 00075 ble.gattClient().onServiceDiscoveryTermination(discoveryTerminationCallback); 00076 ble.gattClient().launchServiceDiscovery(params->handle, serviceDiscoveryCallback, characteristicDiscoveryCallback, 0xa000, 0xa001); 00077 } 00078 ble.gap().disconnect(0,Gap::REMOTE_USER_TERMINATED_CONNECTION); 00079 } 00080 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00081 { 00082 pc.printf("Disconnected\r\n"); 00083 pc.printf("Rescan\r\n"); 00084 ble.startScan(advertisementCallback); 00085 ble.startAdvertising(); 00086 } 00087 00088 00089 int main(void) 00090 { 00091 led1 = 1; 00092 Ticker ticker; 00093 ticker.attach(periodicCallback, 1); 00094 ble.onConnection(connectionCallback); 00095 ble.onDisconnection(disconnectionCallback); 00096 00097 ble.init(); 00098 00099 pc.baud(9600); 00100 pc.printf("Observer Init \r\n"); 00101 00102 00103 /* */ 00104 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00105 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00106 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00107 (const uint8_t *)"DDUDDU", sizeof("DDUDDU") - 1); 00108 00109 ble.gap().setAdvertisingInterval(100); /* 1second. */ 00110 ble.gap().startAdvertising(); 00111 00112 00113 00114 while (true) { 00115 ble.waitForEvent(); 00116 } 00117 }
Generated on Fri Jul 15 2022 16:25:50 by
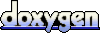