Modified phase table in stepper.cpp to enable stepping a bipolar motor using a twin H-bridge driver.
Fork of stepper by
stepper.h
00001 /* 00002 * mbed library program 00003 * Stepping Motor 00004 * 00005 * Copyright (c) 2014 Kenji Arai / JH1PJL 00006 * http://www.page.sannet.ne.jp/kenjia/index.html 00007 * http://mbed.org/users/kenjiArai/ 00008 * Created: August 20th, 2014 00009 * Revised: August 23rd, 2014 00010 * 00011 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00012 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00013 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00014 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00015 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00016 */ 00017 00018 #ifndef MBED_STEPPER 00019 #define MBED_STEPPER 00020 00021 #include "mbed.h" 00022 00023 #define MT_SLOP_STEP 10 00024 #define MT_MIN_STEP (MT_SLOP_STEP+MT_SLOP_STEP) 00025 #define MT_PLS_WIDTH_MIN 2 // 2mS 00026 00027 /** Unipolar Type Stepping Motor Driver using 4 Outputs(DigitalOut) with timer interrupt(Ticker) 00028 * 00029 * Driver circuit: Low side driver (4ch for one Stepper) e.g. TD62003AP 00030 * CAUTION: This is only for Unipolar Type Stepping Motor! 00031 * Cannot use for Bipolar Type 00032 * Plese refer http://en.wikipedia.org/wiki/Stepper_motor 00033 * 00034 * @code 00035 * #include "mbed.h" 00036 * #include "stepper.h" 00037 * 00038 * #define TIMEBASE 15000 // 15mS 00039 * 00040 * STEPPER sm(D5, D4, D3, D2); 00041 * 00042 * uint8_t pls_width[10] = {5, 4, 3, 2, 1, 1, 1, 1, 1, 1 }; 00043 * 00044 * int main() { 00045 * sm_r.set_max_speed(TIMEBASE); 00046 * sm.move(+100); 00047 * while (sm.status){ ;} 00048 * sm.move(-1000); 00049 * wait(10); 00050 * sm.move(0); 00051 * while(true){;} 00052 * } 00053 * @endcode 00054 */ 00055 00056 class STEPPER { 00057 public: 00058 // Please copy following pls_width[] part in to your main.cpp 00059 /** pulse width definition -> Use for start and stop phase 00060 * = data * TIMEBASE -> e.g following data = 5 then 5*15000/1000 = 75mS 00061 */ 00062 //uint8_t pls_width[MT_SLOP_STEP] = {5, 4, 3, 2, 1, 1, 1, 1, 1, 1 }; 00063 00064 /** Motor Status */ 00065 enum MOTOR_STATE { M_STOP = 0, M_UP, M_CONTINUE, M_DOWN, M_CHANGE, M_UNKOWN = 0xff}; 00066 00067 /** Configure data pin 00068 * @param data SDA and SCL pins 00069 */ 00070 STEPPER (PinName xp, PinName xn, PinName yp, PinName yn); 00071 00072 /** Move steps 00073 * @param number of steps 00074 * @return none 00075 */ 00076 void move (int32_t steps); 00077 00078 /** Set time period (max speed of stepper) 00079 * @param time: e.g. 10mS(=10000) -> 100 PPS(Puls per Sec) 00080 * @return none 00081 */ 00082 void set_max_speed (uint32_t time_base_us); 00083 00084 /** Check status 00085 * @param none 00086 * @return running(= 1), stopped(= 0) 00087 */ 00088 //surely other states can be returned too eg UP, CONTINUE, CHANGE, DOWN? 00089 uint8_t status (void); 00090 00091 /** De-energize coils 00092 * @param none 00093 * @return none 00094 */ 00095 void stop(); 00096 00097 00098 protected: 00099 // Rotation Direction 00100 enum { D_CCW = -1, D_UNKOWN = 0, D_CW = 1}; 00101 00102 typedef struct{ 00103 int8_t direction; 00104 uint32_t total_step; 00105 } Motor_Inf; 00106 00107 typedef struct{ 00108 uint8_t state; 00109 int8_t direction; 00110 uint8_t up_cnt; 00111 uint8_t up_cnt_keep; 00112 uint8_t down_cnt; 00113 uint8_t change_cnt; 00114 uint8_t pls_width; 00115 uint8_t ongoing; 00116 uint32_t continue_cnt; 00117 uint8_t motor_step; //keeps track of phase only, no need of uint32_t 00118 } Motor_Control; 00119 00120 DigitalOut _xp, _xn, _yp, _yn; 00121 Ticker _smdrv; 00122 00123 void set4ports (void); 00124 void setup_mtr_drv_dt(Motor_Inf *mi, Motor_Control *mt); 00125 void millisec_inteval(); 00126 00127 private: 00128 uint8_t busy_sm_drv; 00129 Motor_Inf inf; 00130 Motor_Control cntl; 00131 00132 }; 00133 00134 #endif // MBED_STEPPER
Generated on Thu Jul 14 2022 02:17:18 by
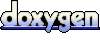