
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
l6474.h
00001 /** 00002 ****************************************************************************** 00003 * @file l6474.h 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief Header for L6474 driver (fully integrated microstepping motor driver) 00008 * @note (C) COPYRIGHT 2014 STMicroelectronics 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __L6474_H 00041 #define __L6474_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "l6474_target_config.h" 00049 #include "motor.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup L6474 00056 * @{ 00057 */ 00058 00059 /* Exported Constants --------------------------------------------------------*/ 00060 00061 /** @defgroup L6474_Exported_Constants 00062 * @{ 00063 */ 00064 00065 /// Current FW version 00066 #define L6474_FW_VERSION (5) 00067 00068 /// L6474 max number of bytes of command & arguments to set a parameter 00069 #define L6474_CMD_ARG_MAX_NB_BYTES (4) 00070 00071 /// L6474 command + argument bytes number for GET_STATUS command 00072 #define L6474_CMD_ARG_NB_BYTES_GET_STATUS (1) 00073 00074 /// L6474 response bytes number 00075 #define L6474_RSP_NB_BYTES_GET_STATUS (2) 00076 00077 /// L6474 value mask for ABS_POS register 00078 #define L6474_ABS_POS_VALUE_MASK ((uint32_t) 0x003FFFFF) 00079 00080 /// L6474 sign bit mask for ABS_POS register 00081 #define L6474_ABS_POS_SIGN_BIT_MASK ((uint32_t) 0x00200000) 00082 00083 /** 00084 * @} 00085 */ 00086 00087 /** @addtogroup L6474_Exported_Variables 00088 * @{ 00089 */ 00090 extern motorDrv_t l6474Drv; 00091 /** 00092 * @} 00093 */ 00094 00095 /* Exported Types -------------------------------------------------------*/ 00096 00097 /** @defgroup L6474_Exported_Types 00098 * @{ 00099 */ 00100 00101 /** @defgroup L6474_Fast_Decay_Time_Options 00102 * @{ 00103 */ 00104 ///TOFF_FAST values for T_FAST register 00105 typedef enum { 00106 L6474_TOFF_FAST_2us = ((uint8_t) 0x00 << 4), 00107 L6474_TOFF_FAST_4us = ((uint8_t) 0x01 << 4), 00108 L6474_TOFF_FAST_6us = ((uint8_t) 0x02 << 4), 00109 L6474_TOFF_FAST_8us = ((uint8_t) 0x03 << 4), 00110 L6474_TOFF_FAST_10us = ((uint8_t) 0x04 << 4), 00111 L6474_TOFF_FAST_12us = ((uint8_t) 0x05 << 4), 00112 L6474_TOFF_FAST_14us = ((uint8_t) 0x06 << 4), 00113 L6474_TOFF_FAST_16us = ((uint8_t) 0x07 << 4), 00114 L6474_TOFF_FAST_18us = ((uint8_t) 0x08 << 4), 00115 L6474_TOFF_FAST_20us = ((uint8_t) 0x09 << 4), 00116 L6474_TOFF_FAST_22us = ((uint8_t) 0x0A << 4), 00117 L6474_TOFF_FAST_24us = ((uint8_t) 0x0B << 4), 00118 L6474_TOFF_FAST_26us = ((uint8_t) 0x0C << 4), 00119 L6474_TOFF_FAST_28us = ((uint8_t) 0x0D << 4), 00120 L6474_TOFF_FAST_30us = ((uint8_t) 0x0E << 4), 00121 L6474_TOFF_FAST_32us = ((uint8_t) 0x0F << 4) 00122 } L6474_TOFF_FAST_t; 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup L6474_Fall_Step_Time_Options 00128 * @{ 00129 */ 00130 ///FAST_STEP values for T_FAST register 00131 typedef enum { 00132 L6474_FAST_STEP_2us = ((uint8_t) 0x00), 00133 L6474_FAST_STEP_4us = ((uint8_t) 0x01), 00134 L6474_FAST_STEP_6us = ((uint8_t) 0x02), 00135 L6474_FAST_STEP_8us = ((uint8_t) 0x03), 00136 L6474_FAST_STEP_10us = ((uint8_t) 0x04), 00137 L6474_FAST_STEP_12us = ((uint8_t) 0x05), 00138 L6474_FAST_STEP_14us = ((uint8_t) 0x06), 00139 L6474_FAST_STEP_16us = ((uint8_t) 0x07), 00140 L6474_FAST_STEP_18us = ((uint8_t) 0x08), 00141 L6474_FAST_STEP_20us = ((uint8_t) 0x09), 00142 L6474_FAST_STEP_22us = ((uint8_t) 0x0A), 00143 L6474_FAST_STEP_24us = ((uint8_t) 0x0B), 00144 L6474_FAST_STEP_26us = ((uint8_t) 0x0C), 00145 L6474_FAST_STEP_28us = ((uint8_t) 0x0D), 00146 L6474_FAST_STEP_30us = ((uint8_t) 0x0E), 00147 L6474_FAST_STEP_32us = ((uint8_t) 0x0F) 00148 } L6474_FAST_STEP_t; 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup L6474_Overcurrent_Threshold_options 00154 * @{ 00155 */ 00156 ///OCD_TH register 00157 typedef enum { 00158 L6474_OCD_TH_375mA = ((uint8_t) 0x00), 00159 L6474_OCD_TH_750mA = ((uint8_t) 0x01), 00160 L6474_OCD_TH_1125mA = ((uint8_t) 0x02), 00161 L6474_OCD_TH_1500mA = ((uint8_t) 0x03), 00162 L6474_OCD_TH_1875mA = ((uint8_t) 0x04), 00163 L6474_OCD_TH_2250mA = ((uint8_t) 0x05), 00164 L6474_OCD_TH_2625mA = ((uint8_t) 0x06), 00165 L6474_OCD_TH_3000mA = ((uint8_t) 0x07), 00166 L6474_OCD_TH_3375mA = ((uint8_t) 0x08), 00167 L6474_OCD_TH_3750mA = ((uint8_t) 0x09), 00168 L6474_OCD_TH_4125mA = ((uint8_t) 0x0A), 00169 L6474_OCD_TH_4500mA = ((uint8_t) 0x0B), 00170 L6474_OCD_TH_4875mA = ((uint8_t) 0x0C), 00171 L6474_OCD_TH_5250mA = ((uint8_t) 0x0D), 00172 L6474_OCD_TH_5625mA = ((uint8_t) 0x0E), 00173 L6474_OCD_TH_6000mA = ((uint8_t) 0x0F) 00174 } L6474_OCD_TH_t; 00175 /** 00176 * @} 00177 */ 00178 00179 /** @defgroup L6474_STEP_MODE_Register_Masks 00180 * @{ 00181 */ 00182 ///STEP_MODE register 00183 typedef enum { 00184 L6474_STEP_MODE_STEP_SEL = ((uint8_t) 0x07), 00185 L6474_STEP_MODE_SYNC_SEL = ((uint8_t) 0x70) 00186 } L6474_STEP_MODE_Masks_t; 00187 /** 00188 * @} 00189 */ 00190 00191 /** @defgroup L6474_STEP_SEL_Options_For_STEP_MODE_Register 00192 * @{ 00193 */ 00194 ///STEP_SEL field of STEP_MODE register 00195 typedef enum { 00196 L6474_STEP_SEL_1 = ((uint8_t) 0x08), //full step 00197 L6474_STEP_SEL_1_2 = ((uint8_t) 0x09), //half step 00198 L6474_STEP_SEL_1_4 = ((uint8_t) 0x0A), //1/4 microstep 00199 L6474_STEP_SEL_1_8 = ((uint8_t) 0x0B), //1/8 microstep 00200 L6474_STEP_SEL_1_16 = ((uint8_t) 0x0C) //1/16 microstep 00201 } L6474_STEP_SEL_t; 00202 /** 00203 * @} 00204 */ 00205 00206 /** @defgroup L6474_SYNC_SEL_Options_For_STEP_MODE_Register 00207 * @{ 00208 */ 00209 ///SYNC_SEL field of STEP_MODE register 00210 typedef enum { 00211 L6474_SYNC_SEL_1_2 = ((uint8_t) 0x80), 00212 L6474_SYNC_SEL_1 = ((uint8_t) 0x90), 00213 L6474_SYNC_SEL_2 = ((uint8_t) 0xA0), 00214 L6474_SYNC_SEL_4 = ((uint8_t) 0xB0), 00215 L6474_SYNC_SEL_8 = ((uint8_t) 0xC0), 00216 L6474_SYNC_SEL_UNUSED = ((uint8_t) 0xD0) 00217 } L6474_SYNC_SEL_t; 00218 /** 00219 * @} 00220 */ 00221 00222 /** @defgroup L6474_ALARM_EN_Register_Options 00223 * @{ 00224 */ 00225 ///ALARM_EN register 00226 typedef enum { 00227 L6474_ALARM_EN_OVERCURRENT = ((uint8_t) 0x01), 00228 L6474_ALARM_EN_THERMAL_SHUTDOWN = ((uint8_t) 0x02), 00229 L6474_ALARM_EN_THERMAL_WARNING = ((uint8_t) 0x04), 00230 L6474_ALARM_EN_UNDERVOLTAGE = ((uint8_t) 0x08), 00231 L6474_ALARM_EN_SW_TURN_ON = ((uint8_t) 0x40), 00232 L6474_ALARM_EN_WRONG_NPERF_CMD = ((uint8_t) 0x80) 00233 } L6474_ALARM_EN_t; 00234 /** 00235 * @} 00236 */ 00237 00238 /** @defgroup L6474_CONFIG_Register_Masks 00239 * @{ 00240 */ 00241 ///CONFIG register 00242 typedef enum { 00243 L6474_CONFIG_OSC_SEL = ((uint16_t) 0x0007), 00244 L6474_CONFIG_EXT_CLK = ((uint16_t) 0x0008), 00245 L6474_CONFIG_EN_TQREG = ((uint16_t) 0x0020), 00246 L6474_CONFIG_OC_SD = ((uint16_t) 0x0080), 00247 L6474_CONFIG_POW_SR = ((uint16_t) 0x0300), 00248 L6474_CONFIG_TOFF = ((uint16_t) 0x7C00) 00249 } L6474_CONFIG_Masks_t; 00250 /** 00251 * @} 00252 */ 00253 00254 /** @defgroup L6474_Clock_Source_Options_For_CONFIG_Register 00255 * @{ 00256 */ 00257 ///Clock source option for CONFIG register 00258 typedef enum { 00259 L6474_CONFIG_INT_16MHZ = ((uint16_t) 0x0000), 00260 L6474_CONFIG_INT_16MHZ_OSCOUT_2MHZ = ((uint16_t) 0x0008), 00261 L6474_CONFIG_INT_16MHZ_OSCOUT_4MHZ = ((uint16_t) 0x0009), 00262 L6474_CONFIG_INT_16MHZ_OSCOUT_8MHZ = ((uint16_t) 0x000A), 00263 L6474_CONFIG_INT_16MHZ_OSCOUT_16MHZ = ((uint16_t) 0x000B), 00264 L6474_CONFIG_EXT_8MHZ_XTAL_DRIVE = ((uint16_t) 0x0004), 00265 L6474_CONFIG_EXT_16MHZ_XTAL_DRIVE = ((uint16_t) 0x0005), 00266 L6474_CONFIG_EXT_24MHZ_XTAL_DRIVE = ((uint16_t) 0x0006), 00267 L6474_CONFIG_EXT_32MHZ_XTAL_DRIVE = ((uint16_t) 0x0007), 00268 L6474_CONFIG_EXT_8MHZ_OSCOUT_INVERT = ((uint16_t) 0x000C), 00269 L6474_CONFIG_EXT_16MHZ_OSCOUT_INVERT = ((uint16_t) 0x000D), 00270 L6474_CONFIG_EXT_24MHZ_OSCOUT_INVERT = ((uint16_t) 0x000E), 00271 L6474_CONFIG_EXT_32MHZ_OSCOUT_INVERT = ((uint16_t) 0x000F) 00272 } L6474_CONFIG_OSC_MGMT_t; 00273 /** 00274 * @} 00275 */ 00276 00277 /** @defgroup L6474_External_Torque_Regulation_Options_For_CONFIG_Register 00278 * @{ 00279 */ 00280 ///External Torque regulation options for CONFIG register 00281 typedef enum { 00282 L6474_CONFIG_EN_TQREG_TVAL_USED = ((uint16_t) 0x0000), 00283 L6474_CONFIG_EN_TQREG_ADC_OUT = ((uint16_t) 0x0020) 00284 } L6474_CONFIG_EN_TQREG_t; 00285 /** 00286 * @} 00287 */ 00288 00289 /** @defgroup L6474_Over_Current_Shutdown_Options_For_CONFIG_Register 00290 * @{ 00291 */ 00292 ///Over Current Shutdown options for CONFIG register 00293 typedef enum { 00294 L6474_CONFIG_OC_SD_DISABLE = ((uint16_t) 0x0000), 00295 L6474_CONFIG_OC_SD_ENABLE = ((uint16_t) 0x0080) 00296 } L6474_CONFIG_OC_SD_t; 00297 /** 00298 * @} 00299 */ 00300 00301 /** @defgroup L6474_Power_Bridge_Output_Slew_Rate_Options 00302 * @{ 00303 */ 00304 /// POW_SR values for CONFIG register 00305 typedef enum { 00306 L6474_CONFIG_SR_320V_us =((uint16_t)0x0000), 00307 L6474_CONFIG_SR_075V_us =((uint16_t)0x0100), 00308 L6474_CONFIG_SR_110V_us =((uint16_t)0x0200), 00309 L6474_CONFIG_SR_260V_us =((uint16_t)0x0300) 00310 } L6474_CONFIG_POW_SR_t; 00311 /** 00312 * @} 00313 */ 00314 00315 /** @defgroup L6474_Off_Time_Options 00316 * @{ 00317 */ 00318 /// TOFF values for CONFIG register 00319 typedef enum { 00320 L6474_CONFIG_TOFF_004us = (((uint16_t) 0x01) << 10), 00321 L6474_CONFIG_TOFF_008us = (((uint16_t) 0x02) << 10), 00322 L6474_CONFIG_TOFF_012us = (((uint16_t) 0x03) << 10), 00323 L6474_CONFIG_TOFF_016us = (((uint16_t) 0x04) << 10), 00324 L6474_CONFIG_TOFF_020us = (((uint16_t) 0x05) << 10), 00325 L6474_CONFIG_TOFF_024us = (((uint16_t) 0x06) << 10), 00326 L6474_CONFIG_TOFF_028us = (((uint16_t) 0x07) << 10), 00327 L6474_CONFIG_TOFF_032us = (((uint16_t) 0x08) << 10), 00328 L6474_CONFIG_TOFF_036us = (((uint16_t) 0x09) << 10), 00329 L6474_CONFIG_TOFF_040us = (((uint16_t) 0x0A) << 10), 00330 L6474_CONFIG_TOFF_044us = (((uint16_t) 0x0B) << 10), 00331 L6474_CONFIG_TOFF_048us = (((uint16_t) 0x0C) << 10), 00332 L6474_CONFIG_TOFF_052us = (((uint16_t) 0x0D) << 10), 00333 L6474_CONFIG_TOFF_056us = (((uint16_t) 0x0E) << 10), 00334 L6474_CONFIG_TOFF_060us = (((uint16_t) 0x0F) << 10), 00335 L6474_CONFIG_TOFF_064us = (((uint16_t) 0x10) << 10), 00336 L6474_CONFIG_TOFF_068us = (((uint16_t) 0x11) << 10), 00337 L6474_CONFIG_TOFF_072us = (((uint16_t) 0x12) << 10), 00338 L6474_CONFIG_TOFF_076us = (((uint16_t) 0x13) << 10), 00339 L6474_CONFIG_TOFF_080us = (((uint16_t) 0x14) << 10), 00340 L6474_CONFIG_TOFF_084us = (((uint16_t) 0x15) << 10), 00341 L6474_CONFIG_TOFF_088us = (((uint16_t) 0x16) << 10), 00342 L6474_CONFIG_TOFF_092us = (((uint16_t) 0x17) << 10), 00343 L6474_CONFIG_TOFF_096us = (((uint16_t) 0x18) << 10), 00344 L6474_CONFIG_TOFF_100us = (((uint16_t) 0x19) << 10), 00345 L6474_CONFIG_TOFF_104us = (((uint16_t) 0x1A) << 10), 00346 L6474_CONFIG_TOFF_108us = (((uint16_t) 0x1B) << 10), 00347 L6474_CONFIG_TOFF_112us = (((uint16_t) 0x1C) << 10), 00348 L6474_CONFIG_TOFF_116us = (((uint16_t) 0x1D) << 10), 00349 L6474_CONFIG_TOFF_120us = (((uint16_t) 0x1E) << 10), 00350 L6474_CONFIG_TOFF_124us = (((uint16_t) 0x1F) << 10) 00351 } L6474_CONFIG_TOFF_t; 00352 /** 00353 * @} 00354 */ 00355 00356 /** @defgroup L6474_STATUS_Register_Bit_Masks 00357 * @{ 00358 */ 00359 ///STATUS Register Bit Masks 00360 typedef enum { 00361 L6474_STATUS_HIZ = (((uint16_t) 0x0001)), 00362 L6474_STATUS_DIR = (((uint16_t) 0x0010)), 00363 L6474_STATUS_NOTPERF_CMD = (((uint16_t) 0x0080)), 00364 L6474_STATUS_WRONG_CMD = (((uint16_t) 0x0100)), 00365 L6474_STATUS_UVLO = (((uint16_t) 0x0200)), 00366 L6474_STATUS_TH_WRN = (((uint16_t) 0x0400)), 00367 L6474_STATUS_TH_SD = (((uint16_t) 0x0800)), 00368 L6474_STATUS_OCD = (((uint16_t) 0x1000)) 00369 } L6474_STATUS_Masks_t; 00370 /** 00371 * @} 00372 */ 00373 00374 /** @defgroup L6474_Direction_Field_Of_STATUS_Register 00375 * @{ 00376 */ 00377 ///Diretion field of STATUS register 00378 typedef enum { 00379 L6474_STATUS_DIR_FORWARD = (((uint16_t) 0x0001) << 4), 00380 L6474_STATUS_DIR_REVERSE = (((uint16_t) 0x0000) << 4) 00381 } L6474_STATUS_DIR_t; 00382 /** 00383 * @} 00384 */ 00385 00386 /** @defgroup L6474_Internal_Register_Addresses 00387 * @{ 00388 */ 00389 /// Internal L6474 register addresses 00390 typedef enum { 00391 L6474_ABS_POS = ((uint8_t) 0x01), 00392 L6474_EL_POS = ((uint8_t) 0x02), 00393 L6474_MARK = ((uint8_t) 0x03), 00394 L6474_RESERVED_REG01 = ((uint8_t) 0x04), 00395 L6474_RESERVED_REG02 = ((uint8_t) 0x05), 00396 L6474_RESERVED_REG03 = ((uint8_t) 0x06), 00397 L6474_RESERVED_REG04 = ((uint8_t) 0x07), 00398 L6474_RESERVED_REG05 = ((uint8_t) 0x08), 00399 L6474_RESERVED_REG06 = ((uint8_t) 0x15), 00400 L6474_TVAL = ((uint8_t) 0x09), 00401 L6474_RESERVED_REG07 = ((uint8_t) 0x0A), 00402 L6474_RESERVED_REG08 = ((uint8_t) 0x0B), 00403 L6474_RESERVED_REG09 = ((uint8_t) 0x0C), 00404 L6474_RESERVED_REG10 = ((uint8_t) 0x0D), 00405 L6474_T_FAST = ((uint8_t) 0x0E), 00406 L6474_TON_MIN = ((uint8_t) 0x0F), 00407 L6474_TOFF_MIN = ((uint8_t) 0x10), 00408 L6474_RESERVED_REG11 = ((uint8_t) 0x11), 00409 L6474_ADC_OUT = ((uint8_t) 0x12), 00410 L6474_OCD_TH = ((uint8_t) 0x13), 00411 L6474_RESERVED_REG12 = ((uint8_t) 0x14), 00412 L6474_STEP_MODE = ((uint8_t) 0x16), 00413 L6474_ALARM_EN = ((uint8_t) 0x17), 00414 L6474_CONFIG = ((uint8_t) 0x18), 00415 L6474_STATUS = ((uint8_t) 0x19), 00416 L6474_RESERVED_REG13 = ((uint8_t) 0x1A), 00417 L6474_RESERVED_REG14 = ((uint8_t) 0x1B), 00418 L6474_INEXISTENT_REG = ((uint8_t) 0x1F) 00419 } L6474_Registers_t; 00420 /** 00421 * @} 00422 */ 00423 00424 /** @defgroup L6474_Command_Set 00425 * @{ 00426 */ 00427 /// L6474 command set 00428 typedef enum { 00429 L6474_NOP = ((uint8_t) 0x00), 00430 L6474_SET_PARAM = ((uint8_t) 0x00), 00431 L6474_GET_PARAM = ((uint8_t) 0x20), 00432 L6474_ENABLE = ((uint8_t) 0xB8), 00433 L6474_DISABLE = ((uint8_t) 0xA8), 00434 L6474_GET_STATUS = ((uint8_t) 0xD0), 00435 L6474_RESERVED_CMD1 = ((uint8_t) 0xEB), 00436 L6474_RESERVED_CMD2 = ((uint8_t) 0xF8) 00437 } L6474_Commands_t; 00438 /** 00439 * @} 00440 */ 00441 00442 00443 /** @defgroup Device_Commands 00444 * @{ 00445 */ 00446 /// Device commands 00447 typedef enum { 00448 RUN_CMD, 00449 MOVE_CMD, 00450 SOFT_STOP_CMD, 00451 NO_CMD 00452 } deviceCommand_t; 00453 /** 00454 * @} 00455 */ 00456 00457 00458 /** @defgroup Device_Parameters 00459 * @{ 00460 */ 00461 00462 /// Device Parameters Structure Type 00463 typedef struct { 00464 /// accumulator used to store speed increase smaller than 1 pps 00465 volatile uint32_t accu; 00466 /// Position in steps at the start of the goto or move commands 00467 volatile int32_t currentPosition; 00468 /// position in step at the end of the accelerating phase 00469 volatile uint32_t endAccPos; 00470 /// nb steps performed from the beggining of the goto or the move command 00471 volatile uint32_t relativePos; 00472 /// position in step at the start of the decelerating phase 00473 volatile uint32_t startDecPos; 00474 /// nb steps to perform for the goto or move commands 00475 volatile uint32_t stepsToTake; 00476 00477 /// acceleration in pps^2 00478 volatile uint16_t acceleration; 00479 /// deceleration in pps^2 00480 volatile uint16_t deceleration; 00481 /// max speed in pps (speed use for goto or move command) 00482 volatile uint16_t maxSpeed; 00483 /// min speed in pps 00484 volatile uint16_t minSpeed; 00485 /// current speed in pps 00486 volatile uint16_t speed; 00487 00488 /// command under execution 00489 volatile deviceCommand_t commandExecuted; 00490 /// FORWARD or BACKWARD direction 00491 volatile motorDir_t direction; 00492 /// Current State of the device 00493 volatile motorState_t motionState; 00494 }deviceParams_t; 00495 00496 /** 00497 * @} 00498 */ 00499 00500 /** 00501 * @} 00502 */ 00503 00504 /* Exported functions --------------------------------------------------------*/ 00505 00506 00507 /** @defgroup L6474_Exported_Functions 00508 * @{ 00509 */ 00510 00511 /** @defgroup Device_Control_Functions 00512 * @{ 00513 */ 00514 void L6474_AttachErrorHandler(void (*callback)(uint16_t)); //Attach a user callback to the error handler 00515 void L6474_AttachFlagInterrupt(void (*callback)(void)); //Attach a user callback to the flag Interrupt 00516 void L6474_Init(uint8_t nbDevices); //Start the L6474 library 00517 uint16_t L6474_GetAcceleration(uint8_t deviceId); //Return the acceleration in pps^2 00518 uint16_t L6474_GetCurrentSpeed(uint8_t deviceId); //Return the current speed in pps 00519 uint16_t L6474_GetDeceleration(uint8_t deviceId); //Return the deceleration in pps^2 00520 motorState_t L6474_GetDeviceState(uint8_t deviceId); //Return the device state 00521 motorDrv_t* L6474_GetMotorHandle(void); //Return handle of the motor driver handle 00522 uint8_t L6474_GetFwVersion(void); //Return the FW version 00523 int32_t L6474_GetMark(uint8_t deviceId); //Return the mark position 00524 uint16_t L6474_GetMaxSpeed(uint8_t deviceId); //Return the max speed in pps 00525 uint16_t L6474_GetMinSpeed(uint8_t deviceId); //Return the min speed in pps 00526 int32_t L6474_GetPosition(uint8_t deviceId); //Return the ABS_POSITION (32b signed) 00527 void L6474_GoHome(uint8_t deviceId); //Move to the home position 00528 void L6474_GoMark(uint8_t deviceId); //Move to the Mark position 00529 void L6474_GoTo(uint8_t deviceId, int32_t targetPosition); //Go to the specified position 00530 void L6474_HardStop(uint8_t deviceId); //Stop the motor and disable the power bridge 00531 void L6474_Move(uint8_t deviceId, //Move the motor of the specified number of steps 00532 motorDir_t direction, 00533 uint32_t stepCount); 00534 uint16_t L6474_ReadId(void); //Read Id to get driver instance 00535 void L6474_ResetAllDevices(void); //Reset all L6474 devices 00536 void L6474_Run(uint8_t deviceId, motorDir_t direction); //Run the motor 00537 bool L6474_SetAcceleration(uint8_t deviceId,uint16_t newAcc); //Set the acceleration in pps^2 00538 bool L6474_SetDeceleration(uint8_t deviceId,uint16_t newDec); //Set the deceleration in pps^2 00539 void L6474_SetHome(uint8_t deviceId); //Set current position to be the home position 00540 void L6474_SetMark(uint8_t deviceId); //Set current position to be the Markposition 00541 bool L6474_SetMaxSpeed(uint8_t deviceId,uint16_t newMaxSpeed); //Set the max speed in pps 00542 bool L6474_SetMinSpeed(uint8_t deviceId,uint16_t newMinSpeed); //Set the min speed in pps 00543 bool L6474_SoftStop(uint8_t deviceId); //Progressively stops the motor 00544 void L6474_WaitWhileActive(uint8_t deviceId); //Wait for the device state becomes Inactive 00545 /** 00546 * @} 00547 */ 00548 00549 /** @defgroup L6474_Control_Functions 00550 * @{ 00551 */ 00552 void L6474_CmdDisable(uint8_t deviceId); //Send the L6474_DISABLE command 00553 void L6474_CmdEnable(uint8_t deviceId); //Send the L6474_ENABLE command 00554 uint32_t L6474_CmdGetParam(uint8_t deviceId, //Send the L6474_GET_PARAM command 00555 uint32_t param); 00556 uint16_t L6474_CmdGetStatus(uint8_t deviceId); // Send the L6474_GET_STATUS command 00557 void L6474_CmdNop(uint8_t deviceId); //Send the L6474_NOP command 00558 void L6474_CmdSetParam(uint8_t deviceId, //Send the L6474_SET_PARAM command 00559 uint32_t param, 00560 uint32_t value); 00561 uint16_t L6474_ReadStatusRegister(uint8_t deviceId); // Read the L6474_STATUS register without 00562 // clearing the flags 00563 void L6474_Reset(void); //Set the L6474 reset pin 00564 void L6474_ReleaseReset(void); //Release the L6474 reset pin 00565 void L6474_SelectStepMode(uint8_t deviceId, // Step mode selection 00566 motorStepMode_t stepMod); 00567 void L6474_SetDirection(uint8_t deviceId, //Set the L6474 direction pin 00568 motorDir_t direction); 00569 /** 00570 * @} 00571 */ 00572 00573 /** @defgroup MotorControl_Board_Linked_Functions 00574 * @{ 00575 */ 00576 ///Delay of the requested number of milliseconds 00577 void BSP_MotorControlBoard_Delay(uint32_t delay); 00578 ///Enable Irq 00579 void BSP_MotorControlBoard_EnableIrq(void); 00580 ///Disable Irq 00581 void BSP_MotorControlBoard_DisableIrq(void); 00582 ///Initialise GPIOs used for L6474s 00583 void BSP_MotorControlBoard_GpioInit(uint8_t nbDevices); 00584 ///Set PWM1 frequency and start it 00585 void BSP_MotorControlBoard_Pwm1SetFreq(uint16_t newFreq); 00586 ///Set PWM2 frequency and start it 00587 void BSP_MotorControlBoard_Pwm2SetFreq(uint16_t newFreq); 00588 ///Set PWM3 frequency and start it 00589 void BSP_MotorControlBoard_Pwm3SetFreq(uint16_t newFreq); 00590 ///Init the PWM of the specified device 00591 void BSP_MotorControlBoard_PwmInit(uint8_t deviceId); 00592 ///Stop the PWM of the specified device 00593 void BSP_MotorControlBoard_PwmStop(uint8_t deviceId); 00594 ///Reset the L6474 reset pin 00595 void BSP_MotorControlBoard_ReleaseReset(void); 00596 ///Set the L6474 reset pin 00597 void BSP_MotorControlBoard_Reset(void); 00598 ///Set direction GPIO 00599 void BSP_MotorControlBoard_SetDirectionGpio(uint8_t deviceId, uint8_t gpioState); 00600 ///Initialise the SPI used for L6474s 00601 uint8_t BSP_MotorControlBoard_SpiInit(void); 00602 ///Write bytes to the L6474s via SPI 00603 uint8_t BSP_MotorControlBoard_SpiWriteBytes(uint8_t *pByteToTransmit, uint8_t *pReceivedByte, uint8_t nbDevices); 00604 /** 00605 * @} 00606 */ 00607 00608 00609 /** 00610 * @} 00611 */ 00612 00613 /** 00614 * @} 00615 */ 00616 00617 /** 00618 * @} 00619 */ 00620 00621 #ifdef __cplusplus 00622 } 00623 #endif 00624 00625 #endif /* #ifndef __L6474_H */ 00626 00627 00628 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
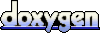