Class library for using the ESP8266 wifi module.
ESP8266 Class Reference
Class library for using the ESP8266 wifi module. More...
#include <ESP8266.h>
Public Member Functions | |
ESP8266 (PinName Tx, PinName Rx, PinName Rst) | |
Create a ESP8266 object connected to the specified pins. | |
bool | startup (uint8_t mode) |
Starts the ESP8266 in a specified mode. | |
void | getVersion (char *version) |
Get the version of AT Command Set from the ESP8266. | |
void | getAPList (char *list) |
Search available AP list and return it. | |
bool | setSoftAPParam (string ssid, string pwd, uint8_t chl=7, uint8_t ecn=4) |
Set SoftAP parameters. | |
bool | DHCP (bool enabled, int mode) |
Enable/Disable DHCP. | |
bool | joinAP (string ssid, string pwd) |
Join in AP. | |
void | getLocalIP (char *LocalIP) |
Get the local IP address of ESP8266. | |
bool | setLocalIP (string LocalIP) |
Sets the IP Address of the ESP8266 Station. | |
bool | setSoftAPIP (string LocalIP) |
Sets the IP Address of the ESP8266 SoftAP. | |
bool | TCPServerStart (uint32_t port=333) |
Start TCP Server(Only in multiple mode). | |
bool | TCPPacketReceived (void) |
Indicates whether a new packet has arrived from a connected client. | |
void | TCPPacketRead (uint8_t *ID, uint32_t *LEN, char DATA[]) |
Reads the current received TCP packet. | |
bool | TCPPacketSend (uint8_t ID, uint32_t LEN, char DATA[]) |
Send a data packet using TCP to a specified client. |
Detailed Description
Class library for using the ESP8266 wifi module.
Definition at line 36 of file ESP8266.h.
Constructor & Destructor Documentation
ESP8266 | ( | PinName | Tx, |
PinName | Rx, | ||
PinName | Rst | ||
) |
Create a ESP8266 object connected to the specified pins.
- Parameters:
-
Tx Pin used to connect to ESP8266's Tx pin Rx Pin used to connect to ESP8266's Rx pin
Definition at line 5 of file ESP8266.cpp.
Member Function Documentation
bool DHCP | ( | bool | enabled, |
int | mode | ||
) |
Enable/Disable DHCP.
- Parameters:
-
enabled DHCP enabled when true mode mode of DHCP 0-softAP, 1-station, 2-both
- Returns:
- true only if ESP8266 enables/disables DHCP successfully
Definition at line 53 of file ESP8266.cpp.
void getAPList | ( | char * | list ) |
Search available AP list and return it.
- Parameters:
-
list String variable where AP list will be stored.
- Note:
- This method will occupy a lot of memeory(hundreds of Bytes to a couple of KBytes). Do not call this method unless you must and ensure that your board has enough memory left.
Definition at line 42 of file ESP8266.cpp.
void getLocalIP | ( | char * | LocalIP ) |
Get the local IP address of ESP8266.
- Parameters:
-
version String variable where version will be stored.
Definition at line 61 of file ESP8266.cpp.
void getVersion | ( | char * | version ) |
Get the version of AT Command Set from the ESP8266.
- Parameters:
-
version String variable where version will be stored.
Definition at line 35 of file ESP8266.cpp.
bool joinAP | ( | string | ssid, |
string | pwd | ||
) |
Join in AP.
- Parameters:
-
ssid SSID of AP to join in. pwd Password of AP to join in.
- Return values:
-
true - success. false - failure.
- Note:
- This method will take a couple of seconds.
Definition at line 57 of file ESP8266.cpp.
bool setLocalIP | ( | string | LocalIP ) |
Sets the IP Address of the ESP8266 Station.
- Parameters:
-
LocalIP The IP Address of the ESP8266 Station
- Return values:
-
true - success. false - failure.
Definition at line 68 of file ESP8266.cpp.
bool setSoftAPIP | ( | string | LocalIP ) |
Sets the IP Address of the ESP8266 SoftAP.
- Parameters:
-
LocalIP The IP Address of the ESP8266 SoftAP
- Return values:
-
true - success. false - failure.
Definition at line 72 of file ESP8266.cpp.
bool setSoftAPParam | ( | string | ssid, |
string | pwd, | ||
uint8_t | chl = 7 , |
||
uint8_t | ecn = 4 |
||
) |
Set SoftAP parameters.
- Parameters:
-
ssid SSID of SoftAP. pwd PASSWORD of SoftAP. chl the channel (1 - 13, default: 7). ecn the way of encrypstion (0 - OPEN, 1 - WEP, 2 - WPA_PSK, 3 - WPA2_PSK, 4 - WPA_WPA2_PSK, default: 4).
- Note:
- This method should not be called when station mode.
Definition at line 49 of file ESP8266.cpp.
bool startup | ( | uint8_t | mode ) |
Starts the ESP8266 in a specified mode.
- Parameters:
-
mode The WiFi mode the ESP8266 must be set to (1=Station, 2=SoftAP, 3=Station+SoftAP)
- Return values:
-
true - succesfull false - not succesfull
Definition at line 10 of file ESP8266.cpp.
void TCPPacketRead | ( | uint8_t * | ID, |
uint32_t * | LEN, | ||
char | DATA[] | ||
) |
Reads the current received TCP packet.
- Parameters:
-
ID Pointer for the ID of client for the new received packet. LEN Pointer for the length of the new received packet. DATA String pointer for the string of the new received packet.
Definition at line 88 of file ESP8266.cpp.
bool TCPPacketReceived | ( | void | ) |
Indicates whether a new packet has arrived from a connected client.
- Return values:
-
true - A new packet has arrived. false - No new packets have arrived.
Definition at line 84 of file ESP8266.cpp.
bool TCPPacketSend | ( | uint8_t | ID, |
uint32_t | LEN, | ||
char | DATA[] | ||
) |
Send a data packet using TCP to a specified client.
- Parameters:
-
ID The identifier of client to send the packet to (available value: 0 - 4). LEN The length of packet to send. DATA The buffer of data to send.
- Return values:
-
true - success. false - failure.
Definition at line 95 of file ESP8266.cpp.
bool TCPServerStart | ( | uint32_t | port = 333 ) |
Start TCP Server(Only in multiple mode).
After started, only methods TCPPacketSend, TCPPacketRead, and TCPPacketArrived can be used. Once TCPServerStop is called, all other methods can be used again.
- Parameters:
-
port The port number to listen on (default: 333).
- Return values:
-
true - success. false - failure.
Definition at line 76 of file ESP8266.cpp.
Generated on Thu Jul 14 2022 01:57:29 by
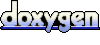