Class library for using the ESP8266 wifi module.
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.h
00001 /* ESP8266 Library v1.0 00002 * Copyright (c) 2017 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * This library was adapted from the WeeESP8266 version by ITEAD STUDIO. 00006 * (https://os.mbed.com/users/itead/code/WeeESP8266/) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 */ 00026 00027 #ifndef ESP8266_H 00028 #define ESP8266_H 00029 00030 #include "mbed.h" 00031 #include <string> 00032 00033 /** Class library for using the ESP8266 wifi module. 00034 */ 00035 00036 class ESP8266 { 00037 public: 00038 /** Create a ESP8266 object connected to the specified pins. 00039 * @param Tx Pin used to connect to ESP8266's Tx pin 00040 * @param Rx Pin used to connect to ESP8266's Rx pin 00041 */ 00042 ESP8266(PinName Tx, PinName Rx, PinName Rst); 00043 00044 /** Starts the ESP8266 in a specified mode. 00045 * @param mode The WiFi mode the ESP8266 must be set to (1=Station, 2=SoftAP, 3=Station+SoftAP) 00046 * 00047 * @retval true - succesfull 00048 * @retval false - not succesfull 00049 */ 00050 bool startup(uint8_t mode); 00051 00052 /** Get the version of AT Command Set from the ESP8266. 00053 * 00054 * @param version String variable where version will be stored. 00055 */ 00056 void getVersion(char* version); 00057 00058 /** Search available AP list and return it. 00059 * 00060 * @param list String variable where AP list will be stored. 00061 * @note This method will occupy a lot of memeory(hundreds of Bytes to a couple of KBytes). 00062 * Do not call this method unless you must and ensure that your board has enough memory left. 00063 */ 00064 void getAPList(char* list); 00065 00066 /** Set SoftAP parameters. 00067 * 00068 * @param ssid SSID of SoftAP. 00069 * @param pwd PASSWORD of SoftAP. 00070 * @param chl the channel (1 - 13, default: 7). 00071 * @param ecn the way of encrypstion (0 - OPEN, 1 - WEP, 00072 * 2 - WPA_PSK, 3 - WPA2_PSK, 4 - WPA_WPA2_PSK, default: 4). 00073 * @note This method should not be called when station mode. 00074 */ 00075 bool setSoftAPParam(string ssid, string pwd, uint8_t chl = 7, uint8_t ecn = 4); 00076 00077 /** Enable/Disable DHCP 00078 * 00079 * @param enabled DHCP enabled when true 00080 * @param mode mode of DHCP 0-softAP, 1-station, 2-both 00081 * @return true only if ESP8266 enables/disables DHCP successfully 00082 */ 00083 bool DHCP(bool enabled, int mode); 00084 00085 /** Join in AP. 00086 * 00087 * @param ssid SSID of AP to join in. 00088 * @param pwd Password of AP to join in. 00089 * @retval true - success. 00090 * @retval false - failure. 00091 * @note This method will take a couple of seconds. 00092 */ 00093 bool joinAP(string ssid, string pwd); 00094 00095 /** Get the local IP address of ESP8266. 00096 * 00097 * @param version String variable where version will be stored. 00098 */ 00099 void getLocalIP(char* LocalIP); 00100 00101 /** Sets the IP Address of the ESP8266 Station. 00102 * 00103 * @param LocalIP The IP Address of the ESP8266 Station 00104 * @retval true - success. 00105 * @retval false - failure. 00106 */ 00107 bool setLocalIP(string LocalIP); 00108 00109 /** Sets the IP Address of the ESP8266 SoftAP. 00110 * 00111 * @param LocalIP The IP Address of the ESP8266 SoftAP 00112 * @retval true - success. 00113 * @retval false - failure. 00114 */ 00115 bool setSoftAPIP(string LocalIP); 00116 00117 /** Start TCP Server(Only in multiple mode). 00118 * 00119 * After started, only methods TCPPacketSend, TCPPacketRead, and TCPPacketArrived can be used. 00120 * Once TCPServerStop is called, all other methods can be used again. 00121 * 00122 * @param port The port number to listen on (default: 333). 00123 * @retval true - success. 00124 * @retval false - failure. 00125 */ 00126 bool TCPServerStart(uint32_t port = 333); 00127 00128 /** Indicates whether a new packet has arrived from a connected client. 00129 * 00130 * @retval true - A new packet has arrived. 00131 * @retval false - No new packets have arrived. 00132 */ 00133 bool TCPPacketReceived(void); 00134 00135 /** Reads the current received TCP packet 00136 * 00137 * @param ID Pointer for the ID of client for the new received packet. 00138 * @param LEN Pointer for the length of the new received packet. 00139 * @param DATA String pointer for the string of the new received packet. 00140 */ 00141 void TCPPacketRead(uint8_t *ID, uint32_t *LEN, char DATA[]); 00142 00143 /** Send a data packet using TCP to a specified client. 00144 * 00145 * @param ID The identifier of client to send the packet to (available value: 0 - 4). 00146 * @param LEN The length of packet to send. 00147 * @param DATA The buffer of data to send. 00148 * @retval true - success. 00149 * @retval false - failure. 00150 */ 00151 bool TCPPacketSend(uint8_t ID, uint32_t LEN, char DATA[]); 00152 00153 00154 private: 00155 Serial esp; //Serial object for connecting to ESP8266 00156 DigitalOut _rst; 00157 bool mDEBUG; 00158 Timer t; 00159 00160 char packetData[1000]; 00161 int packetID; 00162 int packetLen; 00163 char rxtemp[1000]; 00164 int rxtemp_idx; 00165 bool new_rxmsg; //flag to indicate if a new complete message was received 00166 bool rxpass1, rxpass2, rxpass3; 00167 void RxInterrupt(void); //Interrupt to receive data when TCP server is set 00168 00169 bool eAT(void); 00170 bool eATRST(void); 00171 bool eATGMR(string &version); 00172 bool sATCWMODE(uint8_t mode); 00173 bool sATCIPMUX(uint8_t mode); 00174 bool eATCWLAP(string &list); 00175 bool sATCWJAP(string ssid, string pwd); 00176 bool eATCIFSR(string &list); 00177 bool sATCIPSERVER(uint8_t mode, uint32_t port = 333); 00178 bool sATCIPSENDMultiple(uint8_t mux_id, char buffer[], uint32_t len); 00179 bool sATCWSAP(string ssid, string pwd, uint8_t chl, uint8_t ecn); 00180 bool sATCWDHCP(bool enabled, int mode); 00181 bool sATCIPSTA(string ip); 00182 bool sATCIPAP(string ip); 00183 /* 00184 00185 bool qATCWMODE(uint8_t *mode); 00186 00187 00188 00189 bool eATCWQAP(void); 00190 bool sATCWSAP(string ssid, string pwd, uint8_t chl, uint8_t ecn); 00191 bool eATCWLIF(string &list); 00192 00193 bool eATCIPSTATUS(string &list); 00194 bool eATCIFSR(string &list); 00195 00196 */ 00197 00198 00199 00200 00201 /** Verify whether ESP8266 is connected correctly or not. 00202 * @param None 00203 * 00204 * @retval true - connected. 00205 * @retval false - not connected or found. 00206 */ 00207 bool kick(void); 00208 00209 /** Restart ESP8266 by "AT+RST". This method will take 3 seconds or more. 00210 * 00211 * @retval true - success. 00212 * @retval false - failure. 00213 */ 00214 bool reset(void); 00215 00216 /** Sets the ESP8266's WiFi mode. 00217 * @param mode The WiFi mode the ESP8266 must be set to (1=Station, 2=SoftAP, 3=Station+SoftAP) 00218 * 00219 * @retval true - success. 00220 * @retval false - failure. 00221 */ 00222 bool setWiFiMode(uint8_t mode); 00223 00224 /** Enable of disable multiple connections on the ESP8266 00225 * @param onoff Value to Enable(=true) or Disable(=false) multiple connections. 00226 * 00227 * @retval true - success. 00228 * @retval false - failure. 00229 */ 00230 bool setMux(bool onoff); 00231 00232 /* Receive data from uart and search first target. Return true if target found, false for timeout. */ 00233 bool recvFind(string target, uint32_t timeout = 1000); 00234 00235 /* Receive data from uart. Return all received data if target found or timeout. */ 00236 string recvString(string target, uint32_t timeout = 1000); 00237 00238 /* Receive data from uart. Return all received data if one of target1 and target2 found or timeout. */ 00239 string recvString(string target1, string target2, uint32_t timeout = 1000); 00240 00241 /* Receive data from uart. Return all received data if one of target1, target2 and target3 found or timeout. */ 00242 string recvString(string target1, string target2, string target3, uint32_t timeout = 1000); 00243 00244 /* Receive data from uart and search first target and cut out the substring between begin and end(excluding begin and end self). 00245 * Return true if target found, false for timeout. */ 00246 bool recvFindAndFilter(string target, string begin, string end, string &data, uint32_t timeout = 1000); 00247 00248 00249 }; 00250 00251 #endif
Generated on Thu Jul 14 2022 01:57:29 by
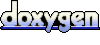