A wrapper Class library for using the touch LCD on the DISCO-F469NI Development board. The class uses the existing BSP class created by Team ST.
Dependencies: BSP_DISCO_F469NI LCD_DISCO_F469NI TS_DISCO_F469NI
DISCOF469LCD Class Reference
A wrapper Class library for using the touch lcd on the DISCO-F469NI Development board. More...
#include <DISCOF469LCD.h>
Public Member Functions | |
DISCOF469LCD (void) | |
Create a DISCOF469LCD object for using the graphics LCD on the DISCO-F469NI Development board. | |
void | Clear (uint32_t Color) |
Clears the LCD by filling it with the specified color pixels. | |
void | DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t Color) |
Draws a pixel on the LCD. | |
uint32_t | ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads the color of an LCD pixel. | |
void | DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint32_t Color) |
Draws a line on the LCD. | |
void | DrawRectangle (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height, uint32_t Color) |
Draws a rectangle on the LCD. | |
void | FillRectangle (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height, uint32_t Color) |
Draws a filled rectangle on the LCD. | |
void | DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius, uint32_t Color) |
Draws a circle on the LCD. | |
void | FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius, uint32_t Color) |
Draws a filled circle on the LCD. | |
void | DrawEllipse (uint16_t Xpos, uint16_t Ypos, uint16_t XRadius, uint16_t YRadius, uint32_t Color) |
Draws an ellipse on the LCD. | |
void | FillEllipse (uint16_t Xpos, uint16_t Ypos, uint16_t XRadius, uint16_t YRadius, uint32_t Color) |
Draws a filled ellipse on the LCD. | |
void | DrawStringAtXY (uint16_t Xpos, uint16_t Ypos, uint8_t FontSize, uint32_t TextColor, uint32_t BackColor, char *Text) |
Displays a string on the LCD at Xpos,Ypos. | |
void | DrawStringAtLine (uint16_t Line, uint8_t FontSize, uint32_t TextColor, uint32_t BackColor, uint8_t Mode, char *Text) |
Displays a string on the LCD at a certain line based on the font size. | |
uint8_t | Touches (void) |
Determines if the touchscreen is being touched or not. | |
void | GetTouch1 (uint16_t *x, uint16_t *y) |
Gets the x and y coordinates of the first touch on the touchscreen. | |
void | GetTouch2 (uint16_t *x, uint16_t *y) |
Gets the x and y coordinates of the second touch on the touchscreen. |
Detailed Description
A wrapper Class library for using the touch lcd on the DISCO-F469NI Development board.
The class uses the existing BSP class created by Team ST.
Example:
#include "mbed.h" #include "DISCOF469LCD.h" DISCOF469LCD lcd; void DisplayPattern(void); int main() { char buf[40]; int numtouches; bool cleared = false; uint16_t x1, y1, x2, y2; DisplayPattern(); wait(4.0); lcd.Clear(LCD_WHITE); while(1) { numtouches = lcd.Touches(); //read if the screen is touched if(numtouches > 0) //has the screen been touched? { cleared = false; //reset clear flag if(numtouches == 1) //if one finger is on the screen { lcd.GetTouch1(&x1, &y1); sprintf(buf, "Touch 1: x=%3u y=%3u", x1, y1); lcd.DrawStringAtLine(0,4,LCD_BLUE, LCD_WHITE, 0, buf); lcd.FillCircle(x1, y1, 50, LCD_RED); } else if(numtouches == 2) //if two fingers are on the screen { lcd.GetTouch1(&x1, &y1); lcd.GetTouch2(&x2, &y2); sprintf(buf, "Touch 1: x=%3u y=%3u", x1, y1); lcd.DrawStringAtLine(0,4,LCD_BLUE, LCD_WHITE, 0, buf); sprintf(buf, "Touch 2: x=%3u y=%3u", x2, y2); lcd.DrawStringAtLine(1,4,LCD_BLUE, LCD_WHITE, 0, buf); lcd.FillCircle(x1, y1, 50, LCD_RED); lcd.FillCircle(x2, y2, 50, LCD_BLUE); } } else { if(!cleared) //if the screen hasn't been cleared { lcd.Clear(LCD_WHITE); //clear the screen after fingers left screen cleared = true; } } } } void DisplayPattern(void) { lcd.FillRectangle(0, 0, 50, 479,LCD_WHITE); lcd.FillRectangle(50, 0, 50, 479,LCD_SILVER); lcd.FillRectangle(100, 0, 50, 479,LCD_GRAY); lcd.FillRectangle(150, 0, 50, 479,LCD_BLACK); lcd.FillRectangle(200, 0, 50, 479,LCD_RED); lcd.FillRectangle(250, 0, 50, 479,LCD_MAROON); lcd.FillRectangle(300, 0, 50, 479,LCD_YELLOW); lcd.FillRectangle(350, 0, 50, 479,LCD_OLIVE); lcd.FillRectangle(400, 0, 50, 479,LCD_LIME); lcd.FillRectangle(450, 0, 50, 479,LCD_GREEN); lcd.FillRectangle(500, 0, 50, 479,LCD_AQUA); lcd.FillRectangle(550, 0, 50, 479,LCD_TEAL); lcd.FillRectangle(600, 0, 50, 479,LCD_BLUE); lcd.FillRectangle(650, 0, 50, 479,LCD_NAVY); lcd.FillRectangle(700, 0, 50, 479,LCD_FUCHSIA); lcd.FillRectangle(750, 0, 50, 479,LCD_PURPLE); lcd.DrawStringAtXY(0, 0, 1, LCD_WHITE, LCD_BLACK, "White"); lcd.DrawStringAtXY(50, 0, 1, LCD_WHITE, LCD_BLACK, "Silver"); lcd.DrawStringAtXY(100, 0, 1, LCD_WHITE, LCD_BLACK, "Gray"); lcd.DrawStringAtXY(150, 0, 1, LCD_WHITE, LCD_BLACK, "Black"); lcd.DrawStringAtXY(200, 0, 1, LCD_WHITE, LCD_BLACK, "Red"); lcd.DrawStringAtXY(250, 0, 1, LCD_WHITE, LCD_BLACK, "Maroon"); lcd.DrawStringAtXY(300, 0, 1, LCD_WHITE, LCD_BLACK, "Yellow"); lcd.DrawStringAtXY(350, 0, 1, LCD_WHITE, LCD_BLACK, "Olive"); lcd.DrawStringAtXY(400, 0, 1, LCD_WHITE, LCD_BLACK, "Lime"); lcd.DrawStringAtXY(450, 0, 1, LCD_WHITE, LCD_BLACK, "Green"); lcd.DrawStringAtXY(500, 0, 1, LCD_WHITE, LCD_BLACK, "Aqua"); lcd.DrawStringAtXY(550, 0, 1, LCD_WHITE, LCD_BLACK, "Teal"); lcd.DrawStringAtXY(600, 0, 1, LCD_WHITE, LCD_BLACK, "Blue"); lcd.DrawStringAtXY(650, 0, 1, LCD_WHITE, LCD_BLACK, "Navy"); lcd.DrawStringAtXY(700, 0, 1, LCD_WHITE, LCD_BLACK, "Fuchsia"); lcd.DrawStringAtXY(750, 0, 1, LCD_WHITE, LCD_BLACK, "Purple"); }
Definition at line 145 of file DISCOF469LCD.h.
Constructor & Destructor Documentation
DISCOF469LCD | ( | void | ) |
Create a DISCOF469LCD object for using the graphics LCD on the DISCO-F469NI Development board.
Definition at line 6 of file DISCOF469LCD.cpp.
Member Function Documentation
void Clear | ( | uint32_t | Color ) |
Clears the LCD by filling it with the specified color pixels.
- Parameters:
-
Color Color to fill the screen with - represented in ARGB8888 color space format.
Definition at line 12 of file DISCOF469LCD.cpp.
void DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius, | ||
uint32_t | Color | ||
) |
Draws a circle on the LCD.
- Parameters:
-
Xpos X position Ypos Y position Radius Circle radius Color Circle color in ARGB mode (8-8-8-8)
Definition at line 44 of file DISCOF469LCD.cpp.
void DrawEllipse | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | XRadius, | ||
uint16_t | YRadius, | ||
uint32_t | Color | ||
) |
Draws an ellipse on the LCD.
- Parameters:
-
Xpos X position Ypos Y position XRadius Ellipse X radius YRadius Ellipse Y radius Color Ellipse color in ARGB mode (8-8-8-8)
Definition at line 56 of file DISCOF469LCD.cpp.
void DrawLine | ( | uint16_t | x1, |
uint16_t | y1, | ||
uint16_t | x2, | ||
uint16_t | y2, | ||
uint32_t | Color | ||
) |
Draws a line on the LCD.
- Parameters:
-
x1 Point 1 X position y1 Point 1 Y position x2 Point 2 X position y2 Point 2 Y position Color Line color in ARGB mode (8-8-8-8)
Definition at line 26 of file DISCOF469LCD.cpp.
void DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint32_t | Color | ||
) |
Draws a pixel on the LCD.
- Parameters:
-
Xpos X position Ypos Y position Color Pixel color in ARGB mode (8-8-8-8)
Definition at line 16 of file DISCOF469LCD.cpp.
void DrawRectangle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height, | ||
uint32_t | Color | ||
) |
Draws a rectangle on the LCD.
- Parameters:
-
Xpos X position Ypos Y position Width Rectangle width Height Rectangle height Color Rectangle color in ARGB mode (8-8-8-8)
Definition at line 32 of file DISCOF469LCD.cpp.
void DrawStringAtLine | ( | uint16_t | Line, |
uint8_t | FontSize, | ||
uint32_t | TextColor, | ||
uint32_t | BackColor, | ||
uint8_t | Mode, | ||
char * | Text | ||
) |
Displays a string on the LCD at a certain line based on the font size.
- Parameters:
-
Line Line where to display the string FontSize The size of the text (0 - 4) TextColor The text foreground color BackColor The text background color Mode Display mode (0=LEFT mode; 1=CENTER mode; 2=RIGHT mode) Text String to display on LCD
Definition at line 85 of file DISCOF469LCD.cpp.
void DrawStringAtXY | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | FontSize, | ||
uint32_t | TextColor, | ||
uint32_t | BackColor, | ||
char * | Text | ||
) |
Displays a string on the LCD at Xpos,Ypos.
- Parameters:
-
Xpos X position (in pixel) Ypos Y position (in pixel) FontSize The size of the text (0 - 4) TextColor The text foreground color BackColor The text background color Text String to display on LCD
Definition at line 69 of file DISCOF469LCD.cpp.
void FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius, | ||
uint32_t | Color | ||
) |
Draws a filled circle on the LCD.
- Parameters:
-
Xpos X position Ypos Y position Radius Circle radius Color Circle color in ARGB mode (8-8-8-8)
Definition at line 50 of file DISCOF469LCD.cpp.
void FillEllipse | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | XRadius, | ||
uint16_t | YRadius, | ||
uint32_t | Color | ||
) |
Draws a filled ellipse on the LCD.
- Parameters:
-
Xpos X position Ypos Y position XRadius Ellipse X radius YRadius Ellipse Y radius Color Ellipse color in ARGB mode (8-8-8-8)
Definition at line 63 of file DISCOF469LCD.cpp.
void FillRectangle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height, | ||
uint32_t | Color | ||
) |
Draws a filled rectangle on the LCD.
- Parameters:
-
Xpos X position Ypos Y position Width Rectangle width Height Rectangle height Color Rectangle color in ARGB mode (8-8-8-8)
Definition at line 38 of file DISCOF469LCD.cpp.
void GetTouch1 | ( | uint16_t * | x, |
uint16_t * | y | ||
) |
Gets the x and y coordinates of the first touch on the touchscreen.
- Parameters:
-
x Pointer to the x coordinate y Pointer to the y coordinate
Definition at line 116 of file DISCOF469LCD.cpp.
void GetTouch2 | ( | uint16_t * | x, |
uint16_t * | y | ||
) |
Gets the x and y coordinates of the second touch on the touchscreen.
- Parameters:
-
x Pointer to the x coordinate y Pointer to the y coordinate
Definition at line 125 of file DISCOF469LCD.cpp.
uint32_t ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads the color of an LCD pixel.
- Parameters:
-
Xpos X position Ypos Y position
- Return values:
-
uint32_t Pixel color in ARGB mode (8-8-8-8)
Definition at line 21 of file DISCOF469LCD.cpp.
uint8_t Touches | ( | void | ) |
Determines if the touchscreen is being touched or not.
- Return values:
-
uint8_t Number of simulantanous touches on screen. 0 = not touched.
Definition at line 108 of file DISCOF469LCD.cpp.
Generated on Thu Jul 14 2022 20:35:52 by
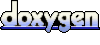