Changes added to reflect changes in MyBuffer which was modified to use static memory allocation.
Fork of BufferedSerialStatic by
BufferedSerial Class Reference
A serial port (UART) for communication with other serial devices. More...
#include <BufferedSerial.h>
Public Member Functions | |
BufferedSerial (PinName tx, PinName rx, const char *name=NULL) | |
Create a BufferedSerial port, connected to the specified transmit and receive pins. | |
virtual | ~BufferedSerial (void) |
Destroy a BufferedSerial port. | |
virtual int | readable (void) |
Check on how many bytes are in the rx buffer. | |
virtual int | writeable (void) |
Check to see if the tx buffer has room. | |
virtual int | getc (void) |
Get a single byte from the BufferedSerial Port. | |
virtual int | putc (int c) |
Write a single byte to the BufferedSerial Port. | |
virtual int | puts (const char *s) |
Write a string to the BufferedSerial Port. | |
virtual int | printf (const char *format,...) |
Write a formatted string to the BufferedSerial Port. | |
virtual ssize_t | write (const void *s, std::size_t length) |
Write data to the Buffered Serial Port. |
Detailed Description
A serial port (UART) for communication with other serial devices.
Can be used for Full Duplex communication, or Simplex by specifying one pin as NC (Not Connected)
Example:
#include "mbed.h" #include "BufferedSerial.h" BufferedSerial pc(USBTX, USBRX); int main() { while(1) { Timer s; s.start(); pc.printf("Hello World - buffered\n"); int buffered_time = s.read_us(); wait(0.1f); // give time for the buffer to empty s.reset(); printf("Hello World - blocking\n"); int polled_time = s.read_us(); s.stop(); wait(0.1f); // give time for the buffer to empty pc.printf("printf buffered took %d us\n", buffered_time); pc.printf("printf blocking took %d us\n", polled_time); wait(0.5f); } }
Software buffers and interrupt driven tx and rx for Serial
Definition at line 71 of file BufferedSerial.h.
Constructor & Destructor Documentation
BufferedSerial | ( | PinName | tx, |
PinName | rx, | ||
const char * | name = NULL |
||
) |
Create a BufferedSerial port, connected to the specified transmit and receive pins.
- Parameters:
-
tx Transmit pin rx Receive pin buf_size printf() buffer size tx_multiple amount of max printf() present in the internal ring buffer at one time name optional name
- Note:
- Either tx or rx may be specified as NC if unused
Definition at line 26 of file BufferedSerial.cpp.
~BufferedSerial | ( | void | ) | [virtual] |
Destroy a BufferedSerial port.
Definition at line 34 of file BufferedSerial.cpp.
Member Function Documentation
int getc | ( | void | ) | [virtual] |
Get a single byte from the BufferedSerial Port.
Should check readable() before calling this.
- Returns:
- A byte that came in on the Serial Port
Definition at line 52 of file BufferedSerial.cpp.
int printf | ( | const char * | format, |
... | |||
) | [virtual] |
Write a formatted string to the BufferedSerial Port.
- Parameters:
-
format The string + format specifiers to write to the Serial Port
- Returns:
- The number of bytes written to the Serial Port Buffer
Definition at line 81 of file BufferedSerial.cpp.
int putc | ( | int | c ) | [virtual] |
Write a single byte to the BufferedSerial Port.
- Parameters:
-
c The byte to write to the Serial Port
- Returns:
- The byte that was written to the Serial Port Buffer
Definition at line 57 of file BufferedSerial.cpp.
int puts | ( | const char * | s ) | [virtual] |
Write a string to the BufferedSerial Port.
Must be NULL terminated
- Parameters:
-
s The string to write to the Serial Port
- Returns:
- The number of bytes written to the Serial Port Buffer
Definition at line 65 of file BufferedSerial.cpp.
int readable | ( | void | ) | [virtual] |
Check on how many bytes are in the rx buffer.
- Returns:
- 1 if something exists, 0 otherwise
Definition at line 42 of file BufferedSerial.cpp.
virtual ssize_t write | ( | const void * | s, |
std::size_t | length | ||
) | [virtual] |
Write data to the Buffered Serial Port.
- Parameters:
-
s A pointer to data to send length The amount of data being pointed to
- Returns:
- The number of bytes written to the Serial Port Buffer
int writeable | ( | void | ) | [virtual] |
Check to see if the tx buffer has room.
- Returns:
- 1 always has room and can overwrite previous content if too small / slow
Definition at line 47 of file BufferedSerial.cpp.
Generated on Wed Jul 13 2022 09:47:30 by
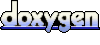