
Library for the SRF02 ultrasonic rangefinder
Embed:
(wiki syntax)
Show/hide line numbers
SRF02.cpp
00001 00002 00003 /*Library for SRF02 ultrasonic range finder*/ 00004 #include "SRF02.h" 00005 00006 00007 00008 SRF02::SRF02(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr){ 00009 //no initialisation code required here 00010 } 00011 00012 SRF02::~SRF02(){ 00013 00014 } 00015 00016 float SRF02::measurecm() { 00017 float result = dosonar(0x51); 00018 return result; 00019 } 00020 00021 float SRF02::measureus() { 00022 float result = dosonar(0x52); 00023 return result; 00024 } 00025 00026 float SRF02::measurein() { 00027 float result = dosonar(0x50); 00028 return result; 00029 } 00030 00031 00032 float SRF02::dosonar(char rangetype){ 00033 //local variables 00034 const char m_addr = 0xE0; //srf02 default slave address 00035 char commandsequence[2]; 00036 00037 // Get range data from SRF02 in cm 00038 // Send Tx burst command over I2C bus 00039 commandsequence[0] = 0x00; // Command register 00040 commandsequence[1] = rangetype; // Ranging results type 00041 m_i2c.write(m_addr, commandsequence, 2); // Request ranging from sensor 00042 wait(0.07); // Average wait time for sonar pulse and processing 00043 // Read back range over I2C bus 00044 commandsequence[0] = 0x02; // 00045 m_i2c.write(m_addr, commandsequence, 1, 1); // 00046 m_i2c.read(m_addr, commandsequence, 2); // Read two-byte echo result 00047 //combine upper and lower bytes in to a single value 00048 float range = ((commandsequence[0] << 8) + commandsequence[1]); 00049 return range; 00050 00051 } 00052
Generated on Fri Jul 15 2022 19:17:58 by
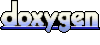