
fix bug crash after 3 minutes
Dependencies: BLE_API MbedJSONValue mbed nRF51822
Fork of BLE_RedBearNano-SAndroidE by
Queue.h
00001 // downloaded from: https://gist.github.com/ArnonEilat/4471278 00002 00003 #include <stdlib.h> 00004 #include <stdio.h> 00005 00006 #define TRUE 1 00007 #define FALSE 0 00008 #define QUEUE_STRING_LENGTH 40 00009 00010 /* a link in the queue, holds the info and point to the next Node*/ 00011 typedef struct { 00012 int length; 00013 char payload[QUEUE_STRING_LENGTH-1]; // null-terminated string, max 256 characters long, this may be JSON or any other format, depending on the application 00014 } DATA; 00015 00016 typedef struct Node_t { 00017 DATA data; 00018 struct Node_t *prev; 00019 } NODE; 00020 00021 /* the HEAD of the Queue, hold the amount of node's that are in the queue*/ 00022 typedef struct Queue { 00023 NODE *head; 00024 NODE *tail; 00025 int size; 00026 int limit; 00027 } Queue; 00028 00029 Queue *ConstructQueue(int limit); 00030 void DestructQueue(Queue *queue); 00031 int Enqueue(Queue *pQueue, NODE *item); 00032 NODE *Dequeue(Queue *pQueue); 00033 int isEmpty(Queue* pQueue); 00034 00035 Queue *ConstructQueue(int limit) { 00036 Queue *queue = (Queue*) malloc(sizeof (Queue)); 00037 if (queue == NULL) { 00038 return NULL; 00039 } 00040 if (limit <= 0) { 00041 limit = 65535; 00042 } 00043 queue->limit = limit; 00044 queue->size = 0; 00045 queue->head = NULL; 00046 queue->tail = NULL; 00047 00048 return queue; 00049 } 00050 00051 void DestructQueue(Queue *queue) { 00052 NODE *pN; 00053 while (!isEmpty(queue)) { 00054 pN = Dequeue(queue); 00055 free(pN); 00056 } 00057 free(queue); 00058 } 00059 00060 int Enqueue(Queue *pQueue, NODE *item) { 00061 /* Bad parameter */ 00062 if ((pQueue == NULL) || (item == NULL)) { 00063 return FALSE; 00064 } 00065 // if(pQueue->limit != 0) 00066 if (pQueue->size >= pQueue->limit) { 00067 return FALSE; 00068 } 00069 /*the queue is empty*/ 00070 item->prev = NULL; 00071 if (pQueue->size == 0) { 00072 pQueue->head = item; 00073 pQueue->tail = item; 00074 00075 } else { 00076 /*adding item to the end of the queue*/ 00077 pQueue->tail->prev = item; 00078 pQueue->tail = item; 00079 } 00080 pQueue->size++; 00081 return TRUE; 00082 } 00083 00084 NODE * Dequeue(Queue *pQueue) { 00085 /*the queue is empty or bad param*/ 00086 NODE *item; 00087 if (isEmpty(pQueue)) 00088 return NULL; 00089 item = pQueue->head; 00090 pQueue->head = (pQueue->head)->prev; 00091 pQueue->size--; 00092 return item; 00093 } 00094 00095 int isEmpty(Queue* pQueue) { 00096 if (pQueue == NULL) { 00097 return FALSE; 00098 } 00099 if (pQueue->size == 0) { 00100 return TRUE; 00101 } else { 00102 return FALSE; 00103 } 00104 } 00105 /* 00106 int main() { 00107 int i; 00108 Queue *pQ = ConstructQueue(7); 00109 NODE *pN; 00110 00111 for (i = 0; i < 9; i++) { 00112 pN = (NODE*) malloc(sizeof (NODE)); 00113 pN->data.info = 100 + i; 00114 Enqueue(pQ, pN); 00115 } 00116 00117 while (!isEmpty(pQ)) { 00118 pN = Dequeue(pQ); 00119 printf("\nDequeued: %d", pN->data); 00120 free(pN); 00121 } 00122 DestructQueue(pQ); 00123 return (EXIT_SUCCESS); 00124 } 00125 00126 */
Generated on Thu Jul 14 2022 07:09:42 by
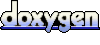