VGA 640x480 full graphic driver
Dependents: vga640x480graphic gps_fastlib Chua-VGA Wolfram-1D-VGA ... more
vga640x480g_functions.h
00001 #include "vga_font.h" 00002 #define FRAMEBUFFERMAX 32000 00003 #define EXTENSIONMAX 6400 00004 #define VGA_HEIGHT 400 00005 #define VGA_WIDTH 640 00006 #include "mbed.h" 00007 00008 extern unsigned char *framebuffer; 00009 extern unsigned char *framebuffer2; 00010 00011 static const unsigned char rmask[]={0x7f,0xbf,0xdf,0xef,0xf7,0xfb,0xfd,0xfe}; 00012 static const unsigned char smask[]={0x80,0x40,0x20,0x10,0x08,0x04,0x02,0x01}; 00013 00014 // clear the screen 00015 void vga_cls() { 00016 memset(framebuffer,0,FRAMEBUFFERMAX); 00017 memset(framebuffer2,0,EXTENSIONMAX); 00018 } 00019 00020 // scroll the screen one text line up 00021 void vga_scroll() { 00022 // move first part up 00023 memcpy(framebuffer,framebuffer+(FONTHEIGHT*80),FRAMEBUFFERMAX-80*(FONTHEIGHT)); 00024 // copy top line of second part into bottom of first part 00025 memcpy(framebuffer+FRAMEBUFFERMAX-80*(FONTHEIGHT),framebuffer2,FONTHEIGHT*80); 00026 // move bottom part up 00027 memcpy(framebuffer2,framebuffer2+(FONTHEIGHT*80),EXTENSIONMAX-80*(FONTHEIGHT)); 00028 // clear bottom row of second part 00029 memset(framebuffer2+EXTENSIONMAX-80*(FONTHEIGHT),0,FONTHEIGHT*80); 00030 } 00031 00032 // set or reset a single pixel on screen 00033 void vga_plot(int x,int y,char color) { 00034 unsigned char *ad; 00035 00036 ad=framebuffer+80*y+((x/8)^1); // calculate offset 00037 if (ad>=framebuffer+FRAMEBUFFERMAX) { // first test for end of framebuffer 00038 ad=framebuffer2+80*(y-400)+((x/8)^1); // calculate offset in framebuffer2 00039 if (ad>framebuffer2+EXTENSIONMAX) return; // clip if exceeded 00040 } 00041 *ad &= rmask[x%8]; // clear the bit 00042 if (color) *ad |= smask[x%8]; // if color != 0 set the bit 00043 00044 } 00045 00046 // put a character from the vga font on screen 00047 void vga_putchar(int x,int y,int ch, char color) { 00048 int n,m; 00049 for (n=0; n<FONTHEIGHT; n++) { 00050 for (m=0; m<FONTWIDTH; m++) { 00051 if (vga_font8x16[ch*FONTHEIGHT+n] & (1<<(7-m))) vga_plot(x+m,y+n, color); 00052 else vga_plot(x+m,y+n,0); 00053 } 00054 } 00055 } 00056 00057 // put a string on screen 00058 void vga_putstring(int x,int y, char *s, char color) { 00059 while (*s) { 00060 vga_putchar(x,y,*(s++),color); 00061 x+=FONTWIDTH; 00062 } 00063 } 00064 00065 // Bresenham line routine 00066 void vga_line(int x0, int y0, int x1, int y1,char color) { 00067 00068 char steep=1; 00069 int li,dx,dy,le; 00070 int sx,sy; 00071 00072 // if same coordinates 00073 if (x0==x1 && y0==y1) { 00074 vga_plot(x0,y0,color); 00075 return; 00076 } 00077 00078 dx = abs(x1-x0); 00079 sx = ((x1 - x0) >0) ? 1 : -1; 00080 dy=abs(y1-y0); 00081 sy = ((y1 - y0) >0) ? 1 : -1; 00082 00083 if (dy > dx) { 00084 steep=0; 00085 // swap X0 and Y0 00086 x0=x0 ^ y0; 00087 y0=x0 ^ y0; 00088 x0=x0 ^ y0; 00089 00090 // swap DX and DY 00091 dx=dx ^ dy; 00092 dy=dx ^ dy; 00093 dx=dx ^ dy; 00094 00095 // swap SX and SY 00096 sx=sx ^ sy; 00097 sy=sx ^ sy; 00098 sx=sx ^ sy; 00099 } 00100 00101 le = (dy << 1) - dx; 00102 00103 for (li=0; li<=dx; li++) { 00104 if (steep) { 00105 vga_plot(x0,y0,color); 00106 } else { 00107 vga_plot(y0,x0,color); 00108 } 00109 while (le >= 0) { 00110 y0 += sy; 00111 le -= (dx << 1); 00112 } 00113 x0 += sx; 00114 le += (dy << 1); 00115 } 00116 } 00117 00118 // draw an outline box 00119 void vga_box(int x0, int y0, int x1, int y1,char color) { 00120 vga_line(x0,y0,x1,y0,color); 00121 vga_line(x1,y0,x1,y1,color); 00122 vga_line(x1,y1,x0,y1,color); 00123 vga_line(x0,y1,x0,y0,color); 00124 } 00125 00126 // draw a filled box 00127 void vga_filledbox(int x0, int y0, int x1, int y1,char color) { 00128 int n,dn; 00129 00130 if (y1>y0) dn=1; 00131 else dn=-1; 00132 00133 for (n=abs(y1-y0); n>=0; n--) { 00134 vga_line(x0,y0,x1,y0,color); 00135 y0+=dn; 00136 } 00137 } 00138 00139 // Bresenham circle routine 00140 void vga_circle(int x0,int y0, int radius, char color) { 00141 00142 int cf = 1 - radius; 00143 int dx = 1; 00144 int dy = -2 * radius; 00145 int cx = 0; 00146 int py = radius; 00147 00148 vga_plot(x0, y0 + radius,color); 00149 vga_plot(x0, y0 - radius,color); 00150 vga_plot(x0 + radius, y0,color); 00151 vga_plot(x0 - radius, y0,color); 00152 00153 while (cx < py) { 00154 if (cf >= 0) { 00155 py--; 00156 dy += 2; 00157 cf += dy; 00158 } 00159 cx++; 00160 dx += 2; 00161 cf += dx; 00162 vga_plot(x0 + cx, y0 + py,color); 00163 vga_plot(x0 - cx, y0 + py,color); 00164 vga_plot(x0 + cx, y0 - py,color); 00165 vga_plot(x0 - cx, y0 - py,color); 00166 vga_plot(x0 + py, y0 + cx,color); 00167 vga_plot(x0 - py, y0 + cx,color); 00168 vga_plot(x0 + py, y0 - cx,color); 00169 vga_plot(x0 - py, y0 - cx,color); 00170 } 00171 }
Generated on Wed Jul 13 2022 01:37:25 by
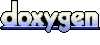