mbed library sources
Fork of mbed-src by
I2C Class Reference
An I2C Master, used for communicating with I2C slave devices. More...
#include <I2C.h>
Public Member Functions | |
I2C (PinName sda, PinName scl) | |
Create an I2C Master interface, connected to the specified pins. | |
void | frequency (int hz) |
Set the frequency of the I2C interface. | |
int | read (int address, char *data, int length, bool repeated=false) |
Read from an I2C slave. | |
int | read (int ack) |
Read a single byte from the I2C bus. | |
int | write (int address, const char *data, int length, bool repeated=false) |
Write to an I2C slave. | |
int | write (int data) |
Write single byte out on the I2C bus. | |
void | start (void) |
Creates a start condition on the I2C bus. | |
void | stop (void) |
Creates a stop condition on the I2C bus. | |
int | transfer (int address, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) |
Start non-blocking I2C transfer. | |
void | abort_transfer () |
Abort the on-going I2C transfer. |
Detailed Description
An I2C Master, used for communicating with I2C slave devices.
Example:
// Read from I2C slave at address 0x62 #include "mbed.h" I2C i2c(p28, p27); int main() { int address = 0x62; char data[2]; i2c.read(address, data, 2); }
Definition at line 50 of file I2C.h.
Constructor & Destructor Documentation
I2C | ( | PinName | sda, |
PinName | scl | ||
) |
Member Function Documentation
void abort_transfer | ( | void | ) |
void frequency | ( | int | hz ) |
int read | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Read from an I2C slave.
Performs a complete read transaction. The bottom bit of the address is forced to 1 to indicate a read.
- Parameters:
-
address 8-bit I2C slave address [ addr | 1 ] data Pointer to the byte-array to read data in to length Number of bytes to read repeated Repeated start, true - don't send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
int read | ( | int | ack ) |
void start | ( | void | ) |
int transfer | ( | int | address, |
const char * | tx_buffer, | ||
int | tx_length, | ||
char * | rx_buffer, | ||
int | rx_length, | ||
const event_callback_t & | callback, | ||
int | event = I2C_EVENT_TRANSFER_COMPLETE , |
||
bool | repeated = false |
||
) |
Start non-blocking I2C transfer.
- Parameters:
-
address 8/10 bit I2c slave address tx_buffer The TX buffer with data to be transfered tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data rx_length The length of RX buffer in bytes event The logical OR of events to modify callback The event callback function repeated Repeated start, true - do not send stop at end
- Returns:
- Zero if the transfer has started, or -1 if I2C peripheral is busy
int write | ( | int | data ) |
int write | ( | int | address, |
const char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Write to an I2C slave.
Performs a complete write transaction. The bottom bit of the address is forced to 0 to indicate a write.
- Parameters:
-
address 8-bit I2C slave address [ addr | 0 ] data Pointer to the byte-array data to send length Number of bytes to send repeated Repeated start, true - do not send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
Generated on Wed Jul 13 2022 01:02:35 by
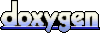