
Port from Avnet's Internet Of Things full WiGo demo: SmartConfig - WebServer - Exosite - Android sensor Fusion App
Dependencies: NVIC_set_all_priorities mbed cc3000_hostdriver_mbedsocket TEMT6200 TSI Wi-Go_eCompass_Lib_V3 WiGo_BattCharger
doTCPIP.cpp
00001 /**************************************************************************** 00002 * 00003 * doTCPIP.cpp - CC3000 TCP/IP 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #include "mbed.h" 00037 #include "defLED.h" 00038 #include "doTCPIP.h" 00039 #include "Wi-Go_eCompass_Lib_V3.h" 00040 00041 extern DigitalOut ledr; 00042 extern DigitalOut ledg; 00043 extern DigitalOut ledb; 00044 extern DigitalOut led1; 00045 extern DigitalOut led2; 00046 extern DigitalOut led3; 00047 00048 extern int do_mDNS; 00049 extern axis6_t axis6; 00050 extern unsigned char newData; 00051 extern int HsecondFlag; 00052 extern unsigned int seconds; 00053 00054 // Setup the functions to handle our CGI parameters 00055 extern char requestBuffer[]; 00056 00057 //Device name - used for Smart config in order to stop the Smart phone configuration process 00058 extern char DevServname; 00059 00060 void runTCPIPserver(void) 00061 { 00062 while(1) 00063 { 00064 long temp, stat, sss, skip, skipc, newsock; 00065 LED_D3_OFF; 00066 LED_D2_OFF; 00067 printf("\n\nStarting TCP/IP Server\n"); 00068 TCPSocketServer server; 00069 server.bind(TCPIP_PORT); 00070 server.listen(); 00071 printf("\r\nWait for new connection...\r\n"); 00072 skip = 0; 00073 skipc = 0; 00074 while(1) 00075 { 00076 newsock = -2; 00077 printf("\r\nServer waiting for connection\r\n"); 00078 TCPSocketConnection client; 00079 LED_D2_ON; 00080 while((newsock == -1) || (newsock == -2)) 00081 { 00082 newsock = server.accept(client); 00083 client.set_blocking(true); 00084 if(do_mDNS) 00085 { 00086 printf("mDNS= 0x%08x\n", wifi._socket.mdns_advertiser(1, (uint8_t *)DevServname, sizeof(DevServname))); 00087 do_mDNS = 0; 00088 } 00089 } 00090 printf("Connection from: %s \r\n", client.get_address()); 00091 sss = seconds; 00092 //receive TCP data 00093 temp = 0; 00094 if(newsock >= 0) 00095 { 00096 client.receive((char *)requestBuffer,20); 00097 printf("Input = %s\n", requestBuffer); 00098 HsecondFlag = 0; 00099 while(1) 00100 { 00101 while(!newData) __wfi(); 00102 newData = 0; 00103 if(HsecondFlag) 00104 { 00105 printf("FB= %d\n", wifi._simple_link.get_number_free_buffers()); 00106 HsecondFlag = 0; 00107 } 00108 LED_D2_ON; 00109 stat = -2; 00110 stat = client.send_all((char *)&axis6, sizeof(axis6)); 00111 LED_D2_OFF; 00112 if(stat != 96) 00113 { 00114 if(stat == -2) 00115 { 00116 skip++; 00117 skipc++; 00118 } 00119 if(stat == -1) break; 00120 } 00121 else 00122 { 00123 temp++; 00124 skipc = 0; 00125 } 00126 if(skipc > 150) 00127 { 00128 printf("Zero Buffer Error Sent=%d, time=%d\n", temp, seconds - sss); 00129 break; 00130 } 00131 } 00132 } else printf("bad socket= %d\n", newsock); 00133 client.close(); 00134 printf("Done %d, time= %d, skipped= %d\n", temp, seconds - sss, skip); 00135 skip = 0; 00136 } 00137 } 00138 }
Generated on Thu Jul 14 2022 00:58:11 by
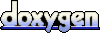