SPI Library for 240x320 TFT LCD with ILI9320, ILI9325 and ILI9328 chip
Dependents: KL25Z_ILI9320_Demo Mini-DK
SPI_TFT_ILI9320.h
00001 /************************************************************************************************** 00002 ***** ***** 00003 ***** Name: SPI_TFT.h ***** 00004 ***** Ver.: 1.0 ***** 00005 ***** Date: 04/01/2013 ***** 00006 ***** Auth: Frank Vannieuwkerke ***** 00007 ***** Erik Olieman ***** 00008 ***** Func: library for 240*320 pixel TFT with ILI9320 LCD Controller ***** 00009 ***** ***** 00010 ***** Rewrite from Peter Drescher code - http://mbed.org/cookbook/SPI-driven-QVGA-TFT ***** 00011 ***** ***** 00012 **************************************************************************************************/ 00013 00014 #ifndef MBED_SPI_TFT_H 00015 #define MBED_SPI_TFT_H 00016 00017 /* This library allows you to store a background image on the local flash memory of the microcontroller, 00018 from any filesystem (such as SD cards). This allows very fast writing of this specific image, and it allows 00019 you to write text in a nice way over the image. However it does cost the last 5 flash sectors of the LPC1768. 00020 Generally that won't be a problem, if it is a problem, add #define NO_FLASH_BUFFER before including this file. 00021 */ 00022 00023 00024 #include "GraphicsDisplay.h" 00025 #include "BurstSPI.h" 00026 #include "mbed.h" 00027 00028 #if defined(TARGET_LPC11U24) || defined(TARGET_LPC1768) 00029 #ifndef NO_FLASH_BUFFER 00030 #include "IAP.h" 00031 #endif 00032 #else 00033 #define NO_FLASH_BUFFER 00034 #define SPI_8BIT 00035 #endif 00036 00037 #define incx() x++, dxt += d2xt, t += dxt 00038 #define incy() y--, dyt += d2yt, t += dyt 00039 00040 #define SPI_F_LO 10000000 00041 #define SPI_F_HI 48000000 00042 00043 /* some RGB565 color definitions */ 00044 #define Black 0x0000 /* 0, 0, 0 */ 00045 #define Navy 0x000F /* 0, 0, 128 */ 00046 #define DarkGreen 0x03E0 /* 0, 128, 0 */ 00047 #define DarkCyan 0x03EF /* 0, 128, 128 */ 00048 #define Maroon 0x7800 /* 128, 0, 0 */ 00049 #define Purple 0x780F /* 128, 0, 128 */ 00050 #define Olive 0x7BE0 /* 128, 128, 0 */ 00051 #define LightGrey 0xC618 /* 192, 192, 192 */ 00052 #define DarkGrey 0x7BEF /* 128, 128, 128 */ 00053 #define Blue 0x001F /* 0, 0, 255 */ 00054 #define Green 0x07E0 /* 0, 255, 0 */ 00055 #define Cyan 0x07FF /* 0, 255, 255 */ 00056 #define Red 0xF800 /* 255, 0, 0 */ 00057 #define Magenta 0xF81F /* 255, 0, 255 */ 00058 #define Yellow 0xFFE0 /* 255, 255, 0 */ 00059 #define White 0xFFFF /* 255, 255, 255 */ 00060 #define Orange 0xFD20 /* 255, 165, 0 */ 00061 #define GreenYellow 0xAFE5 /* 173, 255, 47 */ 00062 00063 /** Class to use TFT displays with an ILI9320 controller using SPI mode 00064 */ 00065 class SPI_TFT : public GraphicsDisplay { 00066 public: 00067 00068 /** Create a SPI_TFT object connected to SPI and two pins 00069 * 00070 * @param mosi,miso,sclk SPI 00071 * @param cs pin connected to CS of display 00072 * @param reset pin connected to RESET of display 00073 * 00074 */ 00075 SPI_TFT(PinName mosi, PinName miso, PinName sclk, PinName cs, const char* name ="TFT"); 00076 00077 /** Write a value to the to a LCD register 00078 * 00079 * @param reg register to be written 00080 * @param val data to be written 00081 */ 00082 void wr_reg (unsigned char reg, unsigned short val); 00083 00084 /** Get the width of the screen in pixels 00085 * 00086 * @param 00087 * @returns width of screen in pixels 00088 * 00089 */ 00090 virtual int width(); 00091 00092 /** Get the height of the screen in pixels 00093 * 00094 * @returns height of screen in pixels 00095 * 00096 */ 00097 virtual int height(); 00098 00099 /** Draw a pixel at x,y with color 00100 * 00101 * @param x (horizontal position) 00102 * @param y (vertical position) 00103 * @param color (16 bit pixel color) 00104 */ 00105 virtual void pixel(int x, int y,int colour); 00106 00107 /** Set draw window region to whole screen 00108 * 00109 */ 00110 void WindowMax (void); 00111 00112 /** draw a 1 pixel line 00113 * 00114 * @param x0,y0 start point 00115 * @param x1,y1 stop point 00116 * @param color 16 bit color 00117 * 00118 */ 00119 void line(int x0, int y0, int x1, int y1, int colour); 00120 00121 /** draw a rect 00122 * 00123 * @param x0,y0 top left corner 00124 * @param w,h width and height 00125 * @param color 16 bit color 00126 * * 00127 */ 00128 void rect(int x0, int y0, int w, int h, int colour); 00129 00130 /** draw a filled rect 00131 * 00132 * @param x0,y0 top left corner 00133 * @param w,h width and height 00134 * @param color 16 bit color 00135 * 00136 */ 00137 void fillrect(int x0, int y0, int w, int h, int colour); 00138 00139 /** draw an ellipse - source : http://enchantia.com/graphapp/doc/tech/ellipses.html 00140 * 00141 * @param xc,yc center point 00142 * @param a,b semi-major axis and semi-minor axis 00143 * @param color 16 bit color 00144 * 00145 */ 00146 void draw_ellipse(int xc, int yc, int a, int b, unsigned int color); 00147 00148 /** draw a filled ellipse - source : http://enchantia.com/graphapp/doc/tech/ellipses.html 00149 * 00150 * @param xc,yc center point 00151 * @param a,b semi-major axis and semi-minor axis 00152 * @param color 16 bit color 00153 * 00154 */ 00155 void fill_ellipse(int xc, int yc, int a, int b, unsigned int color); 00156 00157 /** setup cursor position 00158 * 00159 * @param x x-position (top left) 00160 * @param y y-position 00161 */ 00162 virtual void locate(int x, int y); 00163 00164 /** Fill the screen with _background color 00165 * 00166 */ 00167 virtual void cls (void); 00168 00169 /** Read ILI9320 ID 00170 * 00171 * @returns LCD ID code 00172 * 00173 */ 00174 unsigned short Read_ID(void); 00175 00176 /** calculate the max number of char in a line 00177 * 00178 * @returns max columns 00179 * depends on actual font size 00180 * 00181 */ 00182 virtual int columns(void); 00183 00184 /** calculate the max number of rows 00185 * 00186 * @returns max rows 00187 * depends on actual font size 00188 * 00189 */ 00190 virtual int rows(void); 00191 00192 /** put a char on the screen 00193 * 00194 * @param value char to print 00195 * @returns printed char 00196 * 00197 */ 00198 virtual int _putc(int value); 00199 00200 /** draw a character on given position out of the active font to the TFT 00201 * 00202 * @param x x-position of char (top left) 00203 * @param y y-position 00204 * @param c char to print 00205 * 00206 */ 00207 virtual void character(int x, int y, int c); 00208 00209 /** paint a bitmap on the TFT 00210 * 00211 * @param x,y : upper left corner 00212 * @param w width of bitmap 00213 * @param h high of bitmap 00214 * @param *bitmap pointer to the bitmap data 00215 * 00216 * bitmap format: 16 bit R5 G6 B5 00217 * 00218 * use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 00219 * use winhex to load this file and mark data stating at offset 0x46 to end 00220 * use edit -> copy block -> C Source to export C array 00221 * paste this array into your program 00222 * 00223 * define the array as static const unsigned char to put it into flash memory 00224 * cast the pointer to (unsigned char *) : 00225 * tft.Bitmap(10,40,309,50,(unsigned char *)scala); 00226 */ 00227 void Bitmap(unsigned int x, unsigned int y, unsigned int w, unsigned int h, unsigned char *Name_BMP); 00228 00229 00230 /** paint a BMP (16/24 bit) from filesytem on the TFT (slow) 00231 * 00232 * The 16-bit format is RGB-565. Using 16-bit is faster than 24-bit, however every program can 00233 * output 24 bit BMPs (including MS paint), while it is hard to get them in the correct 16-bit format. 00234 * 00235 * @param x,y : upper left corner 00236 * @param *Name_BMP location of the BMP file (for example "/sd/test.bmp") 00237 * @returns 1 if bmp file was found and stored 00238 * @returns 0 if file was not found 00239 * @returns -1 if file was not a bmp 00240 * @returns -2 if bmp file is not 16/24bit 00241 * @returns -3 if bmp file is wrong for screen 00242 * @returns -4 if buffer malloc goes wrong 00243 * 00244 */ 00245 00246 int Bitmap(unsigned int x, unsigned int y, const char *Name_BMP); 00247 00248 00249 00250 /** select the font to use 00251 * 00252 * @param f pointer to font array 00253 * 00254 * font array can be created with GLCD Font Creator from http://www.mikroe.com 00255 * you have to add 4 parameter at the beginning of the font array to use: 00256 * - the number of bytes / char 00257 * - the vertial size in pixels 00258 * - the horizontal size in pixels 00259 * - the number of bytes per vertical line 00260 * you also have to change the array to char[] 00261 * 00262 */ 00263 void set_font(unsigned char* f); 00264 00265 /** Set the orientation of the screen 00266 * x,y: 0,0 is always top left 00267 * 00268 * @param o direction to use the screen (0-3) 90� Steps 00269 * 00270 */ 00271 void set_orientation(unsigned int o); 00272 00273 00274 /** Modify the orientation of the screen 00275 * x,y: 0,0 is always top left 00276 * 00277 * @param none 00278 * 00279 * ILI9320 limitation: The orientation can only be used with a window command (registers 0x50..0x53) 00280 * 00281 * reg 03h (Entry Mode) : BGR = 1 - ORG = 1 - ID0, ID1 and AM are set according to the orientation variable. 00282 * IMPORTANT : when ORG = 1, the GRAM writing direction follows the orientation (ID0, ID1, AM bits) 00283 * AND we need to use the window command (reg 50h..53h) to write to an area on the display 00284 * because we cannot change reg 20h and 21h to set the GRAM address (they both remain at 00h). 00285 * This means that the pixel routine does not work when ORG = 1. 00286 * Routines relying on the pixel routine first need to set reg 03h = 0x1030 00287 * (cls, circle and line do so) AND need to write the data according to the orientation variable. 00288 */ 00289 void mod_orientation(void); 00290 00291 #ifndef NO_FLASH_BUFFER 00292 /** Move an image to the background buffer 00293 * 00294 * The image must fit exactly on the screen (240x320). This function takes quite some time, depending on source filesystem. 00295 * 00296 * @param *Name_BMP location of the BMP file (for example "/sd/test.bmp") 00297 * @returns 1 if bmp file was found and stored 00298 * @returns 0 if file was not found 00299 * @returns -1 if file was not a bmp 00300 * @returns -2 if bmp file is not 16/24bit 00301 * @returns -3 if bmp file is wrong for screen 00302 * @returns -4 if buffer malloc goes wrong 00303 */ 00304 int fileToFlash(const char *Name_BMP); 00305 00306 /** Use the image on the flash memory as background 00307 * 00308 * @param active - true to use the image, false to use static color 00309 */ 00310 void backgroundImage(bool active); 00311 #endif 00312 00313 00314 00315 00316 00317 // ------------------ PROTECTED PART ------------------ 00318 protected: 00319 00320 /** draw a horizontal line 00321 * 00322 * @param x0 horizontal start 00323 * @param x1 horizontal stop 00324 * @param y vertical position 00325 * @param color 16 bit color 00326 * 00327 */ 00328 void hline(int x0, int x1, int y, int colour); 00329 00330 /** draw a vertical line 00331 * 00332 * @param x horizontal position 00333 * @param y0 vertical start 00334 * @param y1 vertical stop 00335 * @param color 16 bit color 00336 */ 00337 void vline(int y0, int y1, int x, int colour); 00338 00339 /** Set draw window region 00340 * 00341 * @param x horizontal position 00342 * @param y vertical position 00343 * @param w window width in pixel 00344 * @param h window height in pixels 00345 */ 00346 virtual void window (int x, int y, int w, int h); 00347 00348 /** Init the ILI9320 controller 00349 * 00350 */ 00351 void tft_reset(); 00352 00353 /** Write data to the LCD controller 00354 * 00355 * @param dat data written to LCD controller 00356 * 00357 */ 00358 void wr_dat(unsigned short value); 00359 00360 /** Start data sequence to the LCD controller 00361 * 00362 */ 00363 void wr_dat_start(void); 00364 00365 /** Write a command the LCD controller 00366 * 00367 * @param cmd: command to be written 00368 * 00369 */ 00370 void wr_cmd(unsigned char value); 00371 00372 /** Read data from the LCD controller 00373 * 00374 * @returns data from LCD controller 00375 * 00376 */ 00377 unsigned short rd_dat(void); 00378 00379 /** Read a LCD register 00380 * 00381 * @param reg register to be read 00382 * @returns value of the register 00383 */ 00384 unsigned short rd_reg (unsigned char reg); 00385 00386 /** Set the cursor position 00387 * 00388 * @param x (horizontal position) 00389 * @param y (vertical position) 00390 * 00391 * Can only be used when reg 03h = 0x1030 (see note in mod_orientation). 00392 * 00393 */ 00394 void SetCursor( unsigned short Xpos, unsigned short Ypos ); 00395 00396 struct bitmapData { 00397 int return_code; 00398 int width, height; 00399 int bits, pad; 00400 int start_data; 00401 FILE *file; 00402 }; 00403 00404 /** Get bitmap info 00405 * 00406 * @param *Name_BMP Bitmap filename 00407 * @returns structure: return_code 1 if bmp file was found and stored 00408 * 0 if file was not found 00409 * -1 if file was not a bmp 00410 * -2 if bmp file is not 16/24bit 00411 * -3 if bmp file is wrong for screen 00412 * width, height Bitmap size 00413 * bits, pad BPP, padding (multiple of 4 bytes) 00414 * start_data Starting address of the byte where the bitmap image data (pixel array) can be found 00415 * *file Bitmap filename 00416 */ 00417 bitmapData getBitmapData(const char *Name_BMP); 00418 00419 unsigned int orientation; 00420 unsigned int char_x; 00421 unsigned int char_y; 00422 bool backgroundimage; 00423 BurstSPI _spi; 00424 DigitalOut _cs; 00425 unsigned char* font; 00426 #ifndef NO_FLASH_BUFFER 00427 IAP iap; 00428 int backgroundOrientation; 00429 #endif 00430 00431 }; 00432 00433 #endif
Generated on Wed Jul 13 2022 08:41:16 by
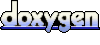