
LPC1768 Mini-DK EasyWeb application with SPI TFT output. Started from EasyWebCR and modified for DM9161 PHY support.
ew_systick.cpp
00001 //***************************************************************************** 00002 // +--+ 00003 // | ++----+ 00004 // +-++ | 00005 // | | 00006 // +-+--+ | 00007 // | +--+--+ 00008 // +----+ Copyright (c) 2009 Code Red Technologies Ltd. 00009 // 00010 // ew_systick.c provided SysTick functions for use by EasyWeb port to RDB1768 00011 // 00012 // Software License Agreement 00013 // 00014 // The software is owned by Code Red Technologies and/or its suppliers, and is 00015 // protected under applicable copyright laws. All rights are reserved. Any 00016 // use in violation of the foregoing restrictions may subject the user to criminal 00017 // sanctions under applicable laws, as well as to civil liability for the breach 00018 // of the terms and conditions of this license. 00019 // 00020 // THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00021 // OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00022 // MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00023 // USE OF THIS SOFTWARE FOR COMMERCIAL DEVELOPMENT AND/OR EDUCATION IS SUBJECT 00024 // TO A CURRENT END USER LICENSE AGREEMENT (COMMERCIAL OR EDUCATIONAL) WITH 00025 // CODE RED TECHNOLOGIES LTD. 00026 00027 #include "mbed.h" 00028 00029 extern void TCPClockHandler(void); 00030 00031 volatile uint32_t TimeTick = 0; 00032 volatile uint32_t TimeTick2 = 0; 00033 00034 DigitalOut myled(P3_25); 00035 Ticker SysT10; 00036 00037 // **************** 00038 // SysTick_Handler 00039 void SysTH(void) 00040 { 00041 TimeTick++; // Increment first SysTick counter 00042 TimeTick2++; // Increment second SysTick counter 00043 00044 // After 100 ticks (100 x 10ms = 1sec) 00045 if (TimeTick >= 100) { 00046 TimeTick = 0; // Reset counter 00047 myled = !myled; 00048 // LPC_GPIO1->FIOPIN ^= 1 << 25; // Toggle user LED 00049 } 00050 // After 20 ticks (20 x 10ms = 1/5sec) 00051 if (TimeTick2 >= 20) { 00052 TimeTick2 = 0; // Reset counter 00053 TCPClockHandler(); // Call TCP handler 00054 } 00055 } 00056 00057 // **************** 00058 // Setup SysTick Timer to interrupt at 10 msec intervals 00059 void Start_SysTick10ms(void) 00060 { 00061 SysT10.attach(&SysTH, 0.01); 00062 }
Generated on Thu Jul 14 2022 05:47:26 by
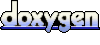