
Demo apps : receive a string from a client and respond with a different string, TCP/IP client
Dependencies: CC3000_Hostdriver mbed
cc3000.cpp
00001 /***************************************************************************** 00002 * 00003 * cc3000 - CC3000 Functions Implementation 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #include "cc3000.h" 00037 #include "doTCPIP.h" 00038 00039 00040 DigitalOut ledr (LED_RED); 00041 DigitalOut ledg (LED_GREEN); 00042 DigitalOut ledb (LED_BLUE); 00043 DigitalOut led1 (PTB8); 00044 DigitalOut led2 (PTB9); 00045 DigitalOut led3 (PTB10); 00046 00047 long ulSocket; 00048 00049 unsigned char pucIP_Addr[4]; 00050 unsigned char pucIP_DefaultGWAddr[4]; 00051 unsigned char pucSubnetMask[4]; 00052 unsigned char pucDNS[4]; 00053 00054 sockaddr tSocketAddr; 00055 00056 unsigned char prefixChangeFlag = 0; 00057 unsigned char prefixFromUser[3] = {0}; 00058 char * ftcPrefixptr; 00059 00060 char aucCC3000_prefix[] = {'T', 'T', 'T'}; // Smart Config Prefix 00061 00062 tNetappIpconfigRetArgs ipinfo; 00063 00064 char cc3000state = CC3000_UNINIT; 00065 extern unsigned char ConnectUsingSmartConfig; 00066 extern volatile unsigned long ulCC3000Connected; 00067 extern volatile unsigned long SendmDNSAdvertisment; 00068 //extern int server_running; 00069 extern char DevServname[]; 00070 volatile unsigned long ulSmartConfigFinished, ulCC3000DHCP, OkToDoShutDown, ulCC3000DHCP_configured; 00071 volatile unsigned char ucStopSmartConfig; 00072 unsigned char pucCC3000_Rx_Buffer[CC3000_APP_BUFFER_SIZE + CC3000_RX_BUFFER_OVERHEAD_SIZE]; 00073 00074 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 00075 const unsigned char smartconfigkey[] = {0x73,0x6d,0x61,0x72,0x74,0x63,0x6f,0x6e,0x66,0x69,0x67,0x41,0x45,0x53,0x31,0x36}; 00076 #endif 00077 00078 00079 void initLEDs(void) 00080 { 00081 RED_OFF; 00082 GREEN_OFF; 00083 BLUE_OFF; 00084 LED_D1_OFF; 00085 LED_D2_OFF; 00086 LED_D3_OFF; 00087 } 00088 00089 00090 int ConnectUsingSSID(char * ssidName) 00091 { 00092 unsetCC3000MachineState(CC3000_ASSOC); 00093 // Disable Profiles and Fast Connect 00094 wlan_ioctl_set_connection_policy(0, 0, 0); 00095 wlan_disconnect(); 00096 wait_ms(3); 00097 // This triggers the CC3000 to connect to specific AP with certain parameters 00098 //sends a request to connect (does not necessarily connect - callback checks that for me) 00099 // wlan_connect(SECURITY, SSID, strlen(SSID), NULL, PASSPHRASE, strlen(PASSPHRASE)); 00100 #ifndef CC3000_TINY_DRIVER 00101 #ifndef AP_KEY 00102 wlan_connect(0, ssidName, strlen(ssidName), NULL, NULL, 0); 00103 #else 00104 wlan_connect(AP_SECURITY, ssidName, strlen(ssidName), NULL, (unsigned char *)AP_KEY , strlen(AP_KEY)); 00105 #endif 00106 #else 00107 wlan_connect(ssidName, strlen(ssidName)); 00108 #endif 00109 // We don't wait for connection. This is handled somewhere else (in the main 00110 // loop for example). 00111 return 0; 00112 } 00113 00114 00115 void CC3000_UsynchCallback(long lEventType, char * data, unsigned char length) 00116 { 00117 if (lEventType == HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE) 00118 { 00119 ulSmartConfigFinished = 1; 00120 ucStopSmartConfig = 1; 00121 } 00122 if (lEventType == HCI_EVNT_WLAN_UNSOL_CONNECT) 00123 { 00124 ulCC3000Connected = 1; 00125 } 00126 if (lEventType == HCI_EVNT_WLAN_UNSOL_DISCONNECT) 00127 { 00128 ulCC3000Connected = 0; 00129 ulCC3000DHCP = 0; 00130 ulCC3000DHCP_configured = 0; 00131 } 00132 if (lEventType == HCI_EVNT_WLAN_UNSOL_DHCP) 00133 { 00134 // Notes: 00135 // 1) IP config parameters are received swapped 00136 // 2) IP config parameters are valid only if status is OK, i.e. ulCC3000DHCP becomes 1 00137 // only if status is OK, the flag is set to 1 and the addresses are valid 00138 if ( *(data + NETAPP_IPCONFIG_MAC_OFFSET) == 0) 00139 { 00140 sprintf( (char*)pucCC3000_Rx_Buffer,"IP:%d.%d.%d.%d\f\r", data[3],data[2], data[1], data[0] ); 00141 ulCC3000DHCP = 1; 00142 } 00143 else 00144 { 00145 ulCC3000DHCP = 0; 00146 } 00147 } 00148 if (lEventType == HCI_EVENT_CC3000_CAN_SHUT_DOWN) 00149 { 00150 OkToDoShutDown = 1; 00151 } 00152 } 00153 00154 00155 int initDriver(void) 00156 { 00157 wlan_start(0); 00158 #if IP_ALLOC_METHOD == USE_DHCP 00159 00160 // DHCP is used by default 00161 // Subnet mask is assumed to be 255.255.255.0 00162 pucSubnetMask[0] = 0; 00163 pucSubnetMask[1] = 0; 00164 pucSubnetMask[2] = 0; 00165 pucSubnetMask[3] = 0; 00166 00167 // CC3000's IP 00168 pucIP_Addr[0] = 0; 00169 pucIP_Addr[1] = 0; 00170 pucIP_Addr[2] = 0; 00171 pucIP_Addr[3] = 0; 00172 00173 // Default Gateway/Router IP 00174 // 192.168.1.1 00175 pucIP_DefaultGWAddr[0] = 0; 00176 pucIP_DefaultGWAddr[1] = 0; 00177 pucIP_DefaultGWAddr[2] = 0; 00178 pucIP_DefaultGWAddr[3] = 0; 00179 00180 // We assume the router is also a DNS server 00181 pucDNS[0] = 0; 00182 pucDNS[1] = 0; 00183 pucDNS[2] = 0; 00184 pucDNS[3] = 0; 00185 00186 // Force DHCP 00187 netapp_dhcp((unsigned long *)pucIP_Addr, 00188 (unsigned long *)pucSubnetMask, 00189 (unsigned long *)pucIP_DefaultGWAddr, 00190 (unsigned long *)pucDNS); 00191 00192 // reset the CC3000 00193 wlan_stop(); 00194 wait(1); 00195 wlan_start(0); 00196 00197 #elif IP_ALLOC_METHOD == USE_STATIC_IP 00198 00199 // Subnet mask is assumed to be 255.255.255.0 00200 pucSubnetMask[0] = 0xFF; 00201 pucSubnetMask[1] = 0xFF; 00202 pucSubnetMask[2] = 0xFF; 00203 pucSubnetMask[3] = 0x0; 00204 00205 // CC3000's IP 00206 pucIP_Addr[0] = STATIC_IP_OCT1; 00207 pucIP_Addr[1] = STATIC_IP_OCT2; 00208 pucIP_Addr[2] = STATIC_IP_OCT3; 00209 pucIP_Addr[3] = STATIC_IP_OCT4; 00210 00211 // Default Gateway/Router IP 00212 // 192.168.1.1 00213 pucIP_DefaultGWAddr[0] = STATIC_IP_OCT1; 00214 pucIP_DefaultGWAddr[1] = STATIC_IP_OCT2; 00215 pucIP_DefaultGWAddr[2] = STATIC_IP_OCT3; 00216 pucIP_DefaultGWAddr[3] = STATIC_GW_OCT4; 00217 00218 // We assume the router is also a DNS server 00219 pucDNS[0] = STATIC_IP_OCT1; 00220 pucDNS[1] = STATIC_IP_OCT2; 00221 pucDNS[2] = STATIC_IP_OCT3; 00222 pucDNS[3] = STATIC_GW_OCT4; 00223 00224 netapp_dhcp((unsigned long *)pucIP_Addr, 00225 (unsigned long *)pucSubnetMask, 00226 (unsigned long *)pucIP_DefaultGWAddr, 00227 (unsigned long *)pucDNS); 00228 00229 // reset the CC3000 to apply Static Setting 00230 wlan_stop(); 00231 wait(1); 00232 wlan_start(0); 00233 00234 #else 00235 #error No IP Configuration Method Selected. One must be configured. 00236 #endif 00237 00238 // Mask out all non-required events from CC3000 00239 wlan_set_event_mask(HCI_EVNT_WLAN_KEEPALIVE| 00240 HCI_EVNT_WLAN_UNSOL_INIT| 00241 HCI_EVNT_WLAN_ASYNC_PING_REPORT); 00242 00243 // CC3000 has been initialized 00244 setCC3000MachineState(CC3000_INIT); 00245 return(0); 00246 } 00247 00248 00249 char highestCC3000State() 00250 { 00251 // We start at the highest state and go down, checking if the state 00252 // is set. 00253 char mask = 0x80; 00254 while(!(cc3000state & mask)) 00255 { 00256 mask = mask >> 1; 00257 } 00258 return mask; 00259 } 00260 00261 00262 char currentCC3000State(void) 00263 { 00264 return cc3000state; 00265 } 00266 00267 00268 void setCC3000MachineState(char stat) 00269 { 00270 cc3000state |= stat; 00271 } 00272 00273 00274 void unsetCC3000MachineState(char stat) 00275 { 00276 char bitmask = stat; 00277 cc3000state &= ~bitmask; 00278 00279 // Set all upper bits to 0 as well since state machine cannot have 00280 // those states. 00281 while(bitmask < 0x80) 00282 { 00283 cc3000state &= ~bitmask; 00284 bitmask = bitmask << 1; 00285 } 00286 } 00287 00288 00289 void resetCC3000StateMachine() 00290 { 00291 cc3000state = CC3000_UNINIT; 00292 } 00293 00294 00295 #ifndef CC3000_TINY_DRIVER 00296 tNetappIpconfigRetArgs * getCC3000Info() 00297 { 00298 if(!(currentCC3000State() & CC3000_SERVER_INIT)) 00299 { 00300 // If we're not blocked by accept or others, obtain the latest 00301 netapp_ipconfig(&ipinfo); 00302 } 00303 return (&ipinfo); 00304 } 00305 #endif 00306 00307 00308 void StartSmartConfig(void) 00309 { 00310 // server_running = 1; 00311 RED_OFF; 00312 GREEN_OFF; 00313 BLUE_ON; 00314 00315 // Reset all the previous configuration 00316 wlan_ioctl_set_connection_policy(0, 0, 0); 00317 wlan_ioctl_del_profile(255); 00318 00319 //Wait until CC3000 is disconected 00320 while (ulCC3000Connected == 1) 00321 { 00322 wait_us(5); 00323 hci_unsolicited_event_handler(); 00324 } 00325 00326 // Trigger the Smart Config process 00327 // Start blinking RED/GREEN during Smart Configuration process 00328 wlan_smart_config_set_prefix(aucCC3000_prefix); 00329 // Start the Smart Config process with AES disabled 00330 wlan_smart_config_start(0); 00331 BLUE_OFF; 00332 RED_ON; 00333 // Wait for Smart config finished 00334 while (ulSmartConfigFinished == 0) 00335 { 00336 wait_ms(250); 00337 RED_ON; 00338 GREEN_OFF; 00339 wait_ms(250); 00340 GREEN_ON; 00341 RED_OFF; 00342 } 00343 BLUE_ON; 00344 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 00345 // create new entry for AES encryption key 00346 nvmem_create_entry(NVMEM_AES128_KEY_FILEID,16); 00347 // write AES key to NVMEM 00348 aes_write_key((unsigned char *)(&smartconfigkey[0])); 00349 // Decrypt configuration information and add profile 00350 wlan_smart_config_process(); 00351 #endif 00352 // wlan_smart_config_process(); 00353 00354 // Configure to connect automatically to the AP retrieved in the 00355 // Smart config process 00356 wlan_ioctl_set_connection_policy(0, 1, 1); 00357 00358 // reset the CC3000 00359 wlan_stop(); 00360 wait(2); 00361 wlan_start(0); 00362 wait(2); 00363 ConnectUsingSmartConfig = 1; 00364 00365 // Mask out all non-required events 00366 wlan_set_event_mask(HCI_EVNT_WLAN_KEEPALIVE|HCI_EVNT_WLAN_UNSOL_INIT|HCI_EVNT_WLAN_ASYNC_PING_REPORT); 00367 RED_OFF; 00368 BLUE_OFF; 00369 GREEN_OFF; 00370 } 00371 00372 00373
Generated on Wed Jul 13 2022 08:35:23 by
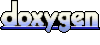