Tricolor LED driver for any 3 pin tricolor LED.
Embed:
(wiki syntax)
Show/hide line numbers
tricolor.h
00001 #ifndef TRICOLOR_H 00002 #define TRICOLOR_H 00003 00004 #include "mbed.h" 00005 00006 /** Tricolor LED driver 00007 * 00008 * This is a reentrant LED controller 00009 * 00010 * @code 00011 #include "mbed.h" 00012 #include "tricolor.h" 00013 00014 Tricolor led(p23, p24, p25); // red, green, blue 00015 00016 int main() { 00017 led.SetLEDColor(0, 100, 0); // Green @ about 40% 00018 00019 while(1) { 00020 wait(0.5); 00021 led.Toggle(); 00022 } 00023 } 00024 * @endcode 00025 */ 00026 class Tricolor 00027 { 00028 public: 00029 /** Basic Constructor 00030 */ 00031 Tricolor(PinName r, PinName g, PinName b) : _Red(r), _Green(g), _Blue(b) { 00032 SetLEDColor(0, 0, 0); 00033 } 00034 /** Color Constructor 00035 * Adds color values to constructor. 00036 */ 00037 Tricolor(PinName r, uint8_t r_color, 00038 PinName g, uint8_t g_color, 00039 PinName b, uint8_t b_color) : 00040 _Red(r), _Green(g), _Blue(b) { 00041 SetLEDColor(r_color, g_color, b_color); 00042 } 00043 /// Sets LED to blue (LED will be on afterwards) 00044 void Blue(void); 00045 /// Sets LED to green (LED will be on afterwards) 00046 void Green(void); 00047 /// Turns the LED off (saves color) 00048 void LEDOff(void); 00049 /// Restores LED to set color (color MUST be set beforehand) 00050 void LEDOn(void); 00051 /// Sets LED to red (LED will be on afterwards) 00052 void Red(void); 00053 /** Sets custom color for LED (LED will be on afterwards) 00054 * @param red Amount of red color (0-255) 00055 * @param green Amount of green color (0-255) 00056 * @param blue Amount of blue color (0-255) 00057 */ 00058 void SetLEDColor(uint8_t, uint8_t, uint8_t); 00059 /// Toggles the LED on and off 00060 void Toggle(void); 00061 00062 private: 00063 // LED Output 00064 bool _on; // Is the LED on 00065 PwmOut _Red; // LED red pin 00066 PwmOut _Green; // LED green pin 00067 PwmOut _Blue; // LED blue pin 00068 00069 // LED Color 00070 float _RedPwm; // Amount of red 00071 float _GreenPwm;// Amount of green 00072 float _BluePwm; // Amount of blue 00073 }; 00074 00075 #endif
Generated on Tue Jul 12 2022 16:54:56 by
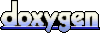